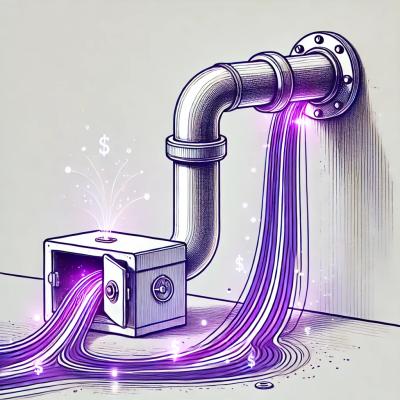
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
gopkg.in/dghubble/sling.v0
Sling is a Go REST client library for creating and sending API requests.
Slings store http Request properties to simplify sending requests and decoding responses. Check usage or the examples to learn how to compose a Sling into your API client.
go get github.com/dghubble/sling
Read GoDoc
Use a simple Sling to set request properties (Path
, QueryParams
, etc.) and then create a new http.Request
by calling Request()
.
req, err := sling.New().Get("https://example.com").Request()
client.Do(req)
Slings are much more powerful though. Use them to create REST clients which wrap complex API endpoints. Copy a base Sling with New()
to avoid repeating common configuration.
const twitterApi = "https://api.twitter.com/1.1/"
base := sling.New().Base(twitterApi).Client(httpAuthClient)
users := base.New().Path("users/")
statuses := base.New().Path("statuses/")
Choose an http method, set query parameters, and send the request.
statuses.New().Get("show.json").QueryStruct(params).Receive(tweet)
The sections below provide more details about setting headers, query parameters, body data, and decoding a typed response after sending.
Add
or Set
headers which should be applied to all Requests created by a Sling.
base := sling.New().Base(baseUrl).Set("User-Agent", "Gophergram API Client")
req, err := base.New().Get("gophergram/list").Request()
Define url parameter structs and use QueryStruct
to encode query parameters.
// Github Issue Parameters
type IssueParams struct {
Filter string `url:"filter,omitempty"`
State string `url:"state,omitempty"`
Labels string `url:"labels,omitempty"`
Sort string `url:"sort,omitempty"`
Direction string `url:"direction,omitempty"`
Since string `url:"since,omitempty"`
}
githubBase := sling.New().Base("https://api.github.com/").Client(httpClient)
path := fmt.Sprintf("repos/%s/%s/issues", owner, repo)
params := &IssueParams{Sort: "updated", State: "open"}
req, err := githubBase.New().Get(path).QueryStruct(params).Request()
Make a Sling include JSON in the Body of its Requests using JsonBody
.
type IssueRequest struct {
Title string `json:"title,omitempty"`
Body string `json:"body,omitempty"`
Assignee string `json:"assignee,omitempty"`
Milestone int `json:"milestone,omitempty"`
Labels []string `json:"labels,omitempty"`
}
githubBase := sling.New().Base("https://api.github.com/").Client(httpClient)
path := fmt.Sprintf("repos/%s/%s/issues", owner, repo)
body := &IssueRequest{
Title: "Test title",
Body: "Some issue",
}
req, err := githubBase.New().Post(path).JsonBody(body).Request()
Requests will include an application/json
Content-Type header.
Make a Sling include a url-tagged struct as a url-encoded form in the Body of its Requests using BodyStruct
.
type StatusUpdateParams struct {
Status string `url:"status,omitempty"`
InReplyToStatusId int64 `url:"in_reply_to_status_id,omitempty"`
MediaIds []int64 `url:"media_ids,omitempty,comma"`
}
tweetParams := &StatusUpdateParams{Status: "writing some Go"}
req, err := twitterBase.New().Post(path).BodyStruct(tweetParams).Request()
Requests will include an application/x-www-form-urlencoded
Content-Type header.
Define expected value structs. Use Receive(v interface{})
to send a new Request that will automatically decode the response into the value.
// Github Issue (abbreviated)
type Issue struct {
Id int `json:"id"`
Url string `json:"url"`
Number int `json:"number"`
State string `json:"state"`
Title string `json:"title"`
Body string `json:"body"`
}
issues := new([]Issue)
resp, err := githubBase.New().Get(path).QueryStruct(params).Receive(issues)
fmt.Println(issues, resp, err)
APIs typically define an endpoint (also called a service) for each type of resource. For example, here is a tiny Github IssueService which creates and lists repository issues.
type IssueService struct {
sling *sling.Sling
}
func NewIssueService(httpClient *http.Client) *IssueService {
return &IssueService{
sling: sling.New().Client(httpClient).Base(baseUrl),
}
}
func (s *IssueService) Create(owner, repo string, issueBody *IssueRequest) (*Issue, *http.Response, error) {
issue := new(Issue)
path := fmt.Sprintf("repos/%s/%s/issues", owner, repo)
resp, err := s.sling.New().Post(path).JsonBody(issueBody).Receive(issue)
return issue, resp, err
}
func (srvc IssueService) List(owner, repo string, params *IssueParams) ([]Issue, *http.Response, error) {
var issues []Issue
path := fmt.Sprintf("repos/%s/%s/issues", owner, repo)
resp, err := srvc.sling.New().Get(path).QueryStruct(params).Receive(&issues)
return *issues, resp, err
}
Create a Pull Request to add a link to your own API.
Many client libraries follow the lead of google/go-github (our inspiration!), but do so by reimplementing logic common to all clients.
This project borrows and abstracts those ideas into a Sling, an agnostic component any API client can use for creating and sending requests.
FAQs
Unknown package
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.