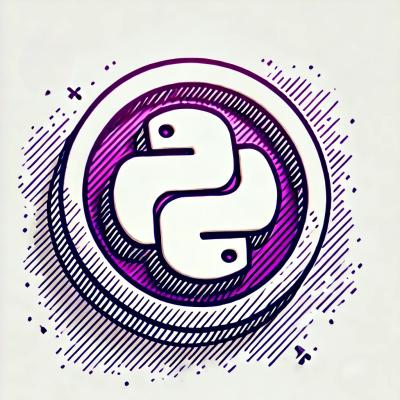
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
ch.ubique.android:qrscanner-zxing
Advanced tools
ZXing based image decoders for the Ubique QR code scanner library
QR Code Scanner for Android built by Ubique based on AndroidX Camera2 with support for ZXing or MLKit decoding.
This library is available on mavenCentral()
// Core library containing the camera view and image analysis use case
implementation 'ch.ubique.android:qrscanner-core:1.1.0'
// ZXing based image decoders
implementation 'ch.ubique.android:qrscanner-zxing:1.1.0'
// MLKit based image decoders
implementation 'ch.ubique.android:qrscanner-mlkit:1.1.0'
// Jetpack Compose support
implementation 'ch.ubique.android:qrscanner-compose:1.1.0'
Check out the example app included in this repository on how to use the library. Note: Camera permission handling is not handled by the library, it is the responsibility of the client app to request the permission.
Include the view in your layout:
<ch.ubique.qrscanner.view.QrScannerView
android:id="@+id/qrScanner"
android:layout_width="match_parent"
android:layout_height="match_parent" />
Define the image decoders to be used to scan for QR codes:
val formats = listOf(BarcodeFormat.QR_CODE, BarcodeFormat.CODE_128)
qrScanner.setImageDecoders(
MLKitImageDecoder(formats), // Available with the qrscanner-mlkit dependency
GlobalHistogramImageDecoder(formats), // Available with the qrscanner-zxing dependency
HybridImageDecoder(formats), // Available with the qrscanner-zxing dependency
)
Set a callback for the decoding state:
qrScanner.setScannercallback { state ->
when (state) {
is DecodingState.NotFound -> {} // None of the image decoders could detect a QR code
is DecodingState.Decoded -> {} // state.content contains the decoded QR code content
is DecodingState.Error -> {} // state.errorCode indicates the type of error that occured during the decoding
}
}
Optional settings:
qrScanner.setFlash(false) // Activate/Deactivate the camera flash
qrScanner.setLinearZoom(0f) // Set the camera zoom on a linear scale from 0f to 1f
qrScanner.setFocusOnTap(true) // Enable/disable camera focus on tap
qrScanner.setScanningMode(ScanningMode.PARALLEL) // Change the scanning behavior when using multiple image decoders
Invoke the composable in your screen:
val formats = listOf(BarcodeFormat.QR_CODE, BarcodeFormat.CODE_128)
QrScanner(
imageDecoders = listOf(
MLKitImageDecoder(formats), // Available with the qrscanner-mlkit dependency
GlobalHistogramImageDecoder(formats), // Available with the qrscanner-zxing dependency
HybridImageDecoder(formats), // Available with the qrscanner-zxing dependency
),
scannerCallback = QrScannerCallback { state ->
when (state) {
is DecodingState.NotFound -> {} // None of the image decoders could detect a QR code
is DecodingState.Decoded -> {} // state.content contains the decoded QR code content
is DecodingState.Error -> {} // state.errorCode indicates the type of error that occured during the decoding
}
},
modifier = Modifier.fillMaxSize(),
scanningMode = ScanningMode.PARALLEL,
isFlashEnabled = remember { mutableStateOf(false) },
linearZoom = remember { mutableStateOf(0f) },
)
This project is licensed under the terms of the MPL 2 license. See the LICENSE file for details.
FAQs
ZXing based image decoders for the Ubique QR code scanner library
We found that ch.ubique.android:qrscanner-zxing demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.