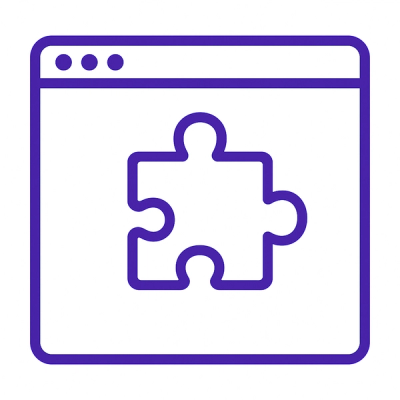
Research
Security News
The Growing Risk of Malicious Browser Extensions
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
io.github.g0dkar:qrcode-kotlin-android-debug
Advanced tools
A Kotlin Library to generate QR Codes without any other dependencies.
💚 Disponível em Português (Brasil) 💛
Creating QRCodes in Kotlin and Java is harder than it should be. QRCode-Kotlin aims to bring a simple, straightforward and customizable way to create QRCodes into the JVM domain, especially in the backend.
~65KB
and it does what it says on the tin.* Well, except maybe the
org.jetbrains.kotlin:kotlin-stdlib-jdk8
one if you use Java...
This library is available from Maven Central,
so you can add QRCode-Kotlin
to your project as a dependency like any other:
Now this is a Multiplatform lib: Starting from version
3.0.0
this library became a Kotlin Multiplatform library. We hope that to you using the library the only change is to declare eitherqrcode-kotlin-jvm
orqrcode-kotlin-android
as the dependency.
If you're using Maven - pom.xml:
<!-- Use this one for normal applications -->
<dependency>
<groupId>io.github.g0dkar</groupId>
<artifactId>qrcode-kotlin-jvm</artifactId>
<version>3.0.0</version>
</dependency>
<!-- Or this one for Android apps 👀 -->
<dependency>
<groupId>io.github.g0dkar</groupId>
<artifactId>qrcode-kotlin-android</artifactId>
<version>3.0.0</version>
</dependency>
If you're using Gradle:
// Kotlin ❤️
// Use this one for normal applications
implementation("io.github.g0dkar:qrcode-kotlin-jvm:3.0.0")
// Or this one for Android apps 👀
implementation("io.github.g0dkar:qrcode-kotlin-android:3.0.0")
// Groovy
// Use this one for normal applications
implementation 'io.github.g0dkar:qrcode-kotlin-jvm:3.0.0'
// Or this one for Android apps 👀
implementation 'io.github.g0dkar:qrcode-kotlin-android:3.0.0'
Here are a few examples of how to use the library to achieve some nice results. If you are interested in more advanced uses and/or fancier QRCodes, please read the documentation :)
Also, make sure to check our examples folder for codes in Kotlin and Java, and the resulting QRCodes!
To generate a simple QRCode:
// By default, the writeImage() method outputs PNGs
val fileOut = FileOutputStream("example01.png")
QRCode("https://github.com/g0dkar/qrcode-kotlin").render().writeImage(fileOut)
Same code as above, but in Java:
// By default, the writeImage() method outputs PNGs
FileOutputStream fileOut = new FileOutputStream("example01-java.png");
new QRCode("https://github.com/g0dkar/qrcode-kotlin").render().writeImage(fileOut);
The render()
function can receive a cellSize
to adjust the size of the resulting QRCode. This parameter represents
the size in pixels of each square of the resulting QRCode. Its default value is 25
:
val fileOut = FileOutputStream("example02.png")
QRCode("https://github.com/g0dkar/qrcode-kotlin")
.render(cellSize = 50)
.writeImage(fileOut)
In Java:
FileOutputStream fileOut = new FileOutputStream("example02-java.png");
new QRCode("https://github.com/g0dkar/qrcode-kotlin")
.render(50)
.writeImage(fileOut);
As of the time of writing, Google's ZXing library is widely used to render QRCodes.
Its rendering of QRCodes usually adds a "border" (aka "margin") around the QRCode, usually equal to 1 cell. The
render()
function can receive a margin
parameter as well, which is how many pixels we want to have as a margin
around our QRCode. By default, the margin
parameter is equal to 0
.
To have one of these nice looking and spaced QRCode, try doing this:
val fileOut = FileOutputStream("example03.png")
val cellSize = 30 // pixels
QRCode("https://github.com/g0dkar/qrcode-kotlin")
.render(cellSize, margin = cellSize)
.writeImage(fileOut)
In Java:
FileOutputStream fileOut = new FileOutputStream("example03-java.png");
int cellSize = 30; // pixels
new QRCode("https://github.com/g0dkar/qrcode-kotlin")
.render(cellSize, cellSize);
.writeImage(fileOut);
Want to have a colorful QRCode? Easy-peasy! The render()
function also have the brightColor
, darkColor
and
marginColor
parameters just for that. Their default values are Black-and-White squares with a White margin.
Starting from v2.0.0 these are simply Int
values in the RGBA space. These can be created with either the new
Colors helper class or if you are running in the JRE
with plain, (very) old java.awt.Color
classes :)
For fun, this will make a QRCode with GitHub's Dark Mode colors:
import io.github.g0dkar.qrcode.render.Colors
val background = Colors.css("#8b949e")
val foreground = Colors.css("#0d1117")
val fileOut = FileOutputStream("example04.png")
QRCode("https://github.com/g0dkar/qrcode-kotlin").render(
brightColor = background, // Background
darkColor = foreground, // Foreground (aka the "black squares")
marginColor = background // Margin (ignored since margin = 0)
).writeImage(fileOut)
In Java:
import java.awt.Color;
Color background = new Color(13, 17, 23);
Color foreground = new Color(139, 148, 158);
FileOutputStream fileOut = new FileOutputStream("example04-java.png");
new QRCode("https://github.com/g0dkar/qrcode-kotlin")
.render(25, 0, background.getRGB(), foreground.getRGB(), background.getRGB())
.writeImage(fileOut);
If you don't want to rely on the basic data-type identification logic implemented by the library, you can specify what
is the type of data that your input string is composed of. You can pass it as the dataType
parameter in the
constructor of the QRCode
class like this:
// Create a "String" typed QRCode instead of a "Number" (which would be automatically identified)
QRCode("42", dataType = QRCodeDataType.DEFAULT)
One of the main reasons I developed this library was to use it on a Spring Boot API that needed to generate QRCodes. So it is only natural to show how to do that :)
import org.springframework.core.io.ByteArrayResource
import org.springframework.http.HttpHeaders.CONTENT_DISPOSITION
import org.springframework.http.MediaType.IMAGE_PNG_VALUE
@GetMapping("/qrcode")
fun generateQrCode(content: String): ResponseEntity<ByteArrayResource> {
val imageOut = ByteArrayOutputStream()
QRCode(content).render().writeImage(imageOut)
val imageBytes = imageOut.toByteArray()
val resource = ByteArrayResource(imageBytes, IMAGE_PNG_VALUE)
return ResponseEntity.ok()
.header(CONTENT_DISPOSITION, "attachment; filename=\"qrcode.png\"")
.body(resource)
}
Copyright 2021 Rafael M. Lins, Licensed under the MIT License.
QR Code is trademarked by Denso Wave, inc.
If you enjoyed the library and want to get me some coffee, use the button below :love_you_gesture:
FAQs
A Kotlin Library to generate QR Codes without any other dependencies.
We found that io.github.g0dkar:qrcode-kotlin-android-debug demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover how browser extensions in trusted stores are used to hijack sessions, redirect traffic, and manipulate user behavior.
Research
Security News
An in-depth analysis of credential stealers, crypto drainers, cryptojackers, and clipboard hijackers abusing open source package registries to compromise Web3 development environments.
Security News
pnpm 10.12.1 introduces a global virtual store for faster installs and new options for managing dependencies with version catalogs.