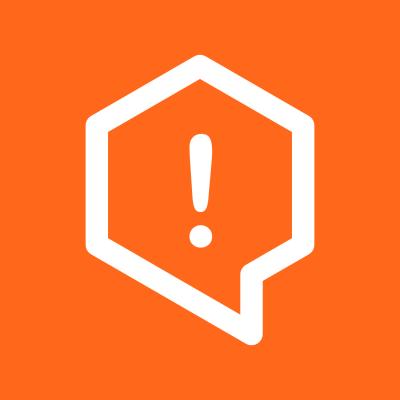
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
5htp-auth-linkedin
Advanced tools
Easy LinkedIn Authentication Plugin for 5HTP.
This plugin is only compatible with projects based on 5HTP, a early-stage Node/TS/Preact framework designed for performance and productivity.
5htp-auth-linkedin
receive an Authorization token, which is then converted to an access token you can finally use to make API requests on LinkedInlinkedinAuth
global
// Run on user's action (ex: when he clicks on the "Login with LinkedIn" button)
$.linkedinAuth.requestAuthCode( context?: object, scopes?: string[] ): Promise<string>;
// Run on LinkedIn's callback response
$.linkedinAuth.handleResponse( linkedInResponse: Request ): Promise<{
profile: { firstName: string, lastName: string, email: string },
context: object,
accessToken: string
}>;
npm i --save 5htp-auth-linkedin
Sign In with LinkedIn
Enable this service plugin in your app by importing it in @/server/index.ts
:
import '5htp-auth-linkedin';
Then, add the following entry in @/server/config.ts
:
{
linkedinAuth: {
id: "<linkedin client ID>",
secret: "<linkedin secret key>",
callbackUrl: '/auth/linkedin/callback'
}
}
@/client/pages/auth.tsx
// Deps
import React from 'react';
import route from '@router';
import Button from '@client/components/button';
// Component
route.page('/auth', {}, null, () => (
<Button link="/auth/linkedin" target="_blank">
Login with Linkedin
</Button>
)
@/server/routes/auth.ts
// Core deps
import app, { $ } from '@server/app';
const route = $.route;
import { Forbidden } from '@common/errors';
// Step 1: Redurect to LinkedIn's authorizatin page
route.get('/auth/linkedin', { }, async ({ response, user }) => {
const redirectUri = await $.linkedinAuth.requestAuthCode({
// You can pass context data to callback (step 2)
userId: user.id
});
return response.redirect(redirectUri);
});
// Step 2: Receive LinkedIn's response and retrieve profile data
route.get('/auth/linkedin/callback', { }, async ({ request }) => {
const {
profile, // User profile data: firstName, lastName, email
context, // The context you passed in step 1
accessToken // The LinkedIn access token if you want do to other API calls
} = await $.linkedinAuth.handleResponse(linkedInResponse);
return `
Hi ${profile.firstName} ${profile.lastName},
Your email is ${profile.email} and I also remind your user ID is ${res.context.userId}.
`
});
FAQs
LinkedIn Authentication Plugin for 5HTP apps.
We found that 5htp-auth-linkedin demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.