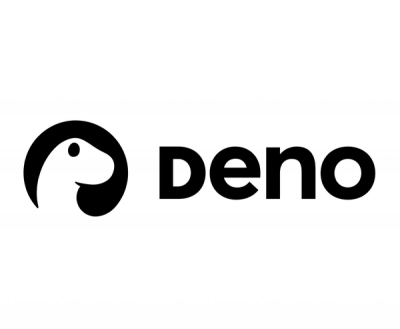
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@10up/component-tooltip
Advanced tools
An accessible tooltip component.
npm install --save @10up/component-tooltip
Clone this repo and import tooltip.js
and tooltip.css
from the dist/
directory.
This component accepts one argument, an optional callback.
This is the markup template expected by the tooltip.
<!--
A Tooltip with a span
-->
<div>
<span class="a11y-tip">
<span class="a11y-tip__trigger">
Tooltip Trigger span
</span>
<span class="a11y-tip__help">
Tooltip content goes here
</span>
</span><!--/.a11y-tip-->
</div>
<!--
A Tooltip with a link
-->
<div>
<span class="a11y-tip a11y-tip--no-delay">
<a href="#tt_id" class="a11y-tip__trigger">
Link w/top tooltip
</a>
<span id="tt_id" class="a11y-tip__help a11y-tip__help--top">
Activate this link to go somewhere!
</span>
</span><!--/.a11y-tip-->
</div>
<!--
A Tooltip with a button
-->
<div>
<span class="a11y-tip">
<button type="button" class="a11y-tip__trigger" aria-describedby="tt_id" aria-controls="tt_id">
Button w/bottom tooltip
</button>
<span id="tt_id" role="tooltip" class="a11y-tip__help a11y-tip__help--bottom">
Buttons do things on the page. Activate it to perform an action.
</span>
</span><!--/.a11y-tip-->
</div>
<!--
A Tooltip with an input
-->
<div>
<label for="test">
Input with right tooltip
</label>
<span class="a11y-tip">
<input id="test" placeholder="enter text" class="a11y-tip__trigger" aria-describedby="test_desc" type="text">
<span id="test_desc" role="tooltip" class="a11y-tip__help a11y-tip__help--right">
Enter something here. Text would be fine.
</span>
</span><!--/.a11y-tip-->
</div>
<!--
Click to show/hide tooltip
-->
<div>
<span class="a11y-tip a11y-tip--toggle">
<span class="a11y-tip__trigger">
Tooltip Toggle Trigger
</span>
<span class="a11y-tip__help">
Tooltip content goes here
</span>
</span><!--/.a11y-tip-->
</div>
The styles can be imported into your existing codebase by using PostCSS imports, or by including the standalone CSS file in your project.
@import '@10up/component-tooltip';
Include the tooltip.css
file from the dist/
directory.
Run the tooltip with an optional callback and the correct mark up to generate the a11y tooltips.
import tooltip from '@10up/component-tooltip';
tooltip( () => {
console.log( 'my awesome callback' );
} );
Include the tooltip.js
file from the dist/
directory and access the component from the gobal TenUp
object.
let myTooltip = new TenUp.tooltip( () => {
console.log( 'my awesome callback' );
} );
Example implementations can be found in the demo
directory.
FAQs
Accessible tooltip component.
The npm package @10up/component-tooltip receives a total of 603 weekly downloads. As such, @10up/component-tooltip popularity was classified as not popular.
We found that @10up/component-tooltip demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.