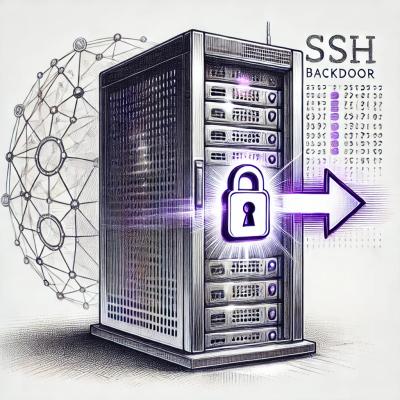
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@aceworks-studio/random
Advanced tools
A Luau utility library to work with randomness.
Add @aceworks-studio/random
in your dependencies:
yarn add @aceworks-studio/random
Or if you are using npm
:
npm install @aceworks-studio/random
function create(random: Random): RandomGenerator
This creates a new instance of the library where each function will use the given random
object.
Contains various utilities to generate simple values.
function boolean(chance: number?): boolean
Returns true
or false
. The chance
argument tells how likely the boolean will be true
and it must be between 0 and 1 (default is 0.5).
function character.pick(characters: string): string
Returns one character from the given characters
string.
function character.letter(): string
Returns one letter from the english alphabet (upper or lower case).
function character.upperCaseLetter(): string
Returns one upper case letter from the english alphabet.
function character.lowerCaseLetter(): string
Returns one lower case letter from the english alphabet.
function character.digit(): string
Returns a digit (0-9
).
function character.alphaNumeric(): string
Returns a digit or a letter from the english alphabet (upper or lower case).
function character.hexDigit(): string
Returns a digit from an hexadecimal base (0-9
or A-F
).
function color.saturated(saturation: number?, value: number?): Color3
Returns a random color with the given saturation and value.
saturation
: between 0 and 1 (default is 1)value
: between 0 and 1 (default is 1)function color.brightness(hue: number, saturation: number?): Color3
Returns a random color with a random brightness.
hue
: between 0 and 1saturation
: between 0 and 1 (default is 1)function color.spreadHue(color: Color3, hueSpan: number): Color3
Returns a random color where the hue is shifted by a maximum amount given by hueSpan
. A hueSpan
of 1 means that it can shift across all colors.
function color.gray(): Color3
Returns a random gray color.
function color.black(chance: number?, fallback: Color3?): Color3
Returns black randomly using the given chance
value.
chance
: how likely the color will be black, between 0-1 (default is 0.5).fallback
: default is whitefunction color.white(chance: number?, fallback: Color3?): Color3
chance
: how likely the color will be white, between 0-1 (default is 0.5).fallback
: default is blackfunction enum(enum: Enum): Enumitem
Returns a random enum item from an enum. Example:
local randomMaterial = values.enum(Enum.Material)
function number.between(
minValue: number,
maxValue: number,
decimals: number?
): number
Returns a number between the given bounds and rounds it with the given number of decimals (if provided)
function number.spread(value: number, span: number): number
Returns a random number shifted by a maximum amount given by span
. The generated number will be between [value - span/2, value + span/2]
.
function number.sign(positiveChance: number?): number
Returns 1
or -1
.
positiveChance
: how likely ([0-1]
) it is to return 1
(default is 0.5).function number.integer.above(value: number): number
Returns a number above value
and the largest safe integer, without including value
.
function number.integer.aboveOrEqual(value: number): number
Returns a number above or equal to value
and the largest safe integer.
function number.integer.below(value: number): number
Returns a number below value
and the smallest safe integer, without including value
.
function number.integer.belowOrEqual(value: number): number
Returns a number below or equal to value
and the smallest safe integer.
function number.integer.between(minValue: number, maxValue: number): number
Returns an integer between the provided bounds.
function string.ofLength(
length: number,
characterGenerator: CharacterSetGenerator
): string
-- where
type CharacterSetGenerator = string | (() -> string)?
Returns a string of the given length
. Uses the characterGenerator
to fill each character.
function string.between(
minLength: number,
maxLength: number,
characterGenerator: CharacterSetGenerator
): string
-- where
type CharacterSetGenerator = string | (() -> string)?
Returns a string of a random length between the given bounds. Uses the characterGenerator
to fill each character.
function string.substring(value: string, length: number?): string
Returns a substring of the given value
. If the length
is not provided, it uses chooses a random length between 1 and the length of value
.
function vector2.unit(): Vector2
Returns a Vector2 of length equal to 1.
function vector2.ofLength(length: number): Vector2
Returns a Vector2 the given length.
function vector2.inCircle(radius: number, center: Vector2?): Vector2
Returns a Vector2 within the bounds of a circle centered at center
(default to (0, 0)
).
function vector2.inRectangle(rectangle: Rectangle): Vector2
-- where
type Rectangle =
{ size: Vector2, center: Vector2 }
| { pointA: Vector2, pointB: Vector2 }
Returns a Vector2 within the bounds of the provided rectangle.
function vector3.unit(): Vector3
Returns a Vector3 of length equal to 1.
function vector3.ofLength(length: number): Vector3
Returns a Vector3 the given length.
function vector3.inSphere(radius: number, center: Vector3?): Vector3
Returns a Vector3 within the bounds of a sphere centered at center
(default to (0, 0, 0)
).
function vector3.inBox(size: Vector3, center: Vector3): Vector3
Returns a Vector3 within the bounds of a sphere centered at center
(default to (0, 0, 0)
).
function array.ofLength<T>(length: number, generator: () -> T) -> { T },
Creates an array of the given length using the generator function.
function array.between<T>(minLength: number, maxLength: number, generator: () -> T) -> { T },
Creates an array of a random length between the given bounds using the generator function.
function array.shuffle<T>(array: { T }) -> { T },
Returns a new array with the same elements in a different order.
This function does not modify the original array.
function array.shuffleInPlace<T>(array: { T }) -> (),
Mutates an array to mix the elements in a different order.
function array.pickOne<T>(array: { T }) -> T?,
Returns one element from the array. Returns nil
if array
is empty.
function array.pickMultiple<T>(array: { T }, count: number) -> { T },
Returns a new array with count
elements picked from array
. The returned array may be empty if array
is empty.
function array.pickMultipleOnce<T>(array: { T }, count: number) -> { T },
Similar to pickMultiple
, but it returns a new array with count
elements picked from array
only once. The returned array cannot be larger than the provided array
and it may be empty if array
is empty.
function weighted.array<T>(elements: { T }, weights: { number }): WeightedChoiceGenerator<T>
Creates a WeightedChoiceGenerator that can pick values from elements
. Each relative probability of the values in elements
are provided through weights
.
function weighted.map<T>(map: { [T]: number }): WeightedChoiceGenerator<T>
Creates a WeightedChoiceGenerator that can pick values from the keys of map
. Each key is mapped to the relative probability to get picked.
Provides functions to build a WeightedChoiceGenerator<T>
from a set of choices and their relative probability weights. It has these three functions:
function pickOne(): T?
Returns one element from the array. Returns nil
if the original array is empty.
function pickMultiple(count: number): { T }
Returns a new array with count
elements picked from the original array. The returned array may be empty if the original array is empty.
function pickMultipleOnce(count: number): { T }
Similar to pickMultiple
, but it returns a new array with count
elements picked from original array only once. The returned array cannot be larger than the original array and it may be empty if original array is empty.
This project is available under the MIT license. See LICENSE.txt for details.
If you would like to use this library on a Lua environment where it is currently incompatible, open an issue (or comment on an existing one) to request the appropriate modifications.
The library uses darklua to process its code.
FAQs
A Luau utility library to work with randomness
The npm package @aceworks-studio/random receives a total of 1 weekly downloads. As such, @aceworks-studio/random popularity was classified as not popular.
We found that @aceworks-studio/random demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.