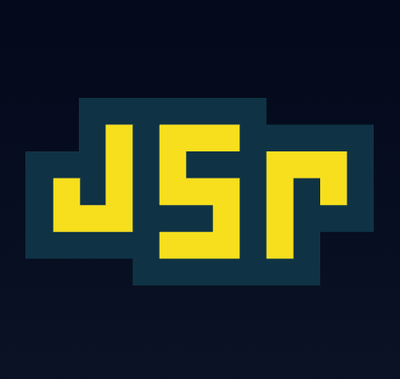
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@actions/core
Advanced tools
The @actions/core package provides a set of core functions for GitHub Actions users to help manage inputs, outputs, and other features within their GitHub Actions workflows. It simplifies the process of coding actions by offering utility functions for common tasks.
Getting action inputs
This feature allows you to easily retrieve inputs defined in your action's YAML file. The example code demonstrates how to get an input with the name 'inputName', marking it as required.
const input = core.getInput('inputName', { required: true });
Setting action outputs
Enables you to set outputs for your action, which can be used by other steps in your workflow. The code sample shows how to set an output with a key of 'outputKey' and a value of 'outputValue'.
core.setOutput('outputKey', 'outputValue');
Logging
Provides various logging functions to help debug or provide information during the execution of your action. The code demonstrates how to log an information message, a warning message, and an error message.
core.info('Information message');
core.warning('Warning message');
core.error('Error message');
Setting environment variables
Allows you to set environment variables that will be available to subsequent steps in your workflow. The example code sets an environment variable named 'VAR_NAME' with a value of 'value'.
core.exportVariable('VAR_NAME', 'value');
Setting a secret
This function registers a value as a secret, which masks the value from logs. The code sample demonstrates how to mask 'secretValue' from GitHub Actions logs.
core.setSecret('secretValue');
While @actions/core provides general utilities for GitHub Actions, @actions/github offers GitHub-specific functionalities, such as interacting with GitHub API directly from your actions. It complements @actions/core by providing a higher level of GitHub integration.
This package is designed to execute system commands within GitHub Actions. It's similar to @actions/core in that it provides utility functions for actions, but focuses on command execution, allowing you to run shell commands, capture output, and handle errors.
Offers utilities for file system operations, such as moving, copying, and deleting files or directories within GitHub Actions. It's a complementary package to @actions/core, focusing on file and directory manipulation tasks.
@actions/core
Core functions for setting results, logging, registering secrets and exporting variables across actions
You can use this library to get inputs or set outputs:
const core = require('@actions/core');
const myInput = core.getInput('inputName', { required: true });
// Do stuff
core.setOutput('outputKey', 'outputVal');
You can also export variables for future steps. Variables get set in the environment.
const core = require('@actions/core');
// Do stuff
core.exportVariable('envVar', 'Val');
You can explicitly add items to the path for all remaining steps in a workflow:
const core = require('@actions/core');
core.addPath('pathToTool');
You should use this library to set the failing exit code for your action:
const core = require('@actions/core');
try {
// Do stuff
}
catch (err) {
// setFailed logs the message and sets a failing exit code
core.setFailed(`Action failed with error ${err}`);
}
Finally, this library provides some utilities for logging. Note that debug logging is hidden from the logs by default. This behavior can be toggled by enabling the Step Debug Logs.
const core = require('@actions/core');
const myInput = core.getInput('input');
try {
core.debug('Inside try block');
if (!myInput) {
core.warning('myInput was not set');
}
// Do stuff
}
catch (err) {
core.error(`Error ${err}, action may still succeed though`);
}
This library can also wrap chunks of output in foldable groups.
const core = require('@actions/core')
// Manually wrap output
core.startGroup('Do some function')
doSomeFunction()
core.endGroup()
// Wrap an asynchronous function call
const result = await core.group('Do something async', async () => {
const response = await doSomeHTTPRequest()
return response
})
FAQs
Actions core lib
We found that @actions/core demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.