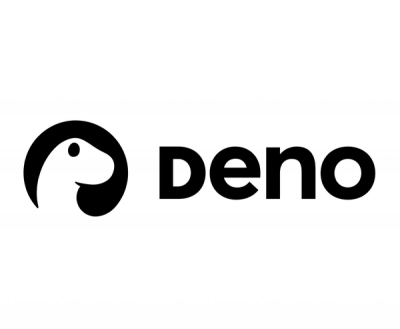
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@actions/github
Advanced tools
The @actions/github npm package provides a set of tools and functionalities to interact with GitHub within GitHub Actions. It allows for the automation of GitHub workflows, including repository management, issue handling, and pull requests among other GitHub operations. This package leverages the GitHub API and the GitHub Actions environment to enable developers to create sophisticated CI/CD workflows.
Creating an issue
This code demonstrates how to create a new issue in a GitHub repository using the @actions/github package. It utilizes the `getOctokit` method to authenticate with the GitHub API using a token and then calls the `create` method on `issues` to create a new issue.
const github = require('@actions/github');
const core = require('@actions/core');
async function createIssue() {
const token = core.getInput('repo-token');
const octokit = github.getOctokit(token);
const response = await octokit.rest.issues.create({
owner: 'owner-name',
repo: 'repo-name',
title: 'New Issue Title',
body: 'Issue description.'
});
console.log(response.url);
}
createIssue();
Commenting on a pull request
This example shows how to automatically comment on a pull request using the @actions/github package. It first checks if the GitHub Actions context has a pull request payload, extracts the pull request number, and then uses the `createComment` method to post a comment.
const github = require('@actions/github');
const core = require('@actions/core');
async function commentOnPR() {
const token = core.getInput('repo-token');
const octokit = github.getOctokit(token);
const context = github.context;
if (context.payload.pull_request) {
const prNumber = context.payload.pull_request.number;
const response = await octokit.rest.issues.createComment({
owner: context.repo.owner,
repo: context.repo.repo,
issue_number: prNumber,
body: 'Automated comment on PR.'
});
console.log(response.url);
}
}
commentOnPR();
github-api is another JavaScript package that provides an interface to the GitHub API. It offers functionalities similar to @actions/github, such as repository management and issue handling. However, github-api is not specifically tailored for GitHub Actions and might be more suitable for general GitHub API interactions within JavaScript applications. It differs from @actions/github by its broader focus, not being limited to GitHub Actions workflows.
@actions/github
A hydrated Octokit client.
Returns an authenticated Octokit client that follows the machine proxy settings and correctly sets GHES base urls. See https://octokit.github.io/rest.js for the API.
const github = require('@actions/github');
const core = require('@actions/core');
async function run() {
// This should be a token with access to your repository scoped in as a secret.
// The YML workflow will need to set myToken with the GitHub Secret Token
// myToken: ${{ secrets.GITHUB_TOKEN }}
// https://help.github.com/en/actions/automating-your-workflow-with-github-actions/authenticating-with-the-github_token#about-the-github_token-secret
const myToken = core.getInput('myToken');
const octokit = github.getOctokit(myToken)
// You can also pass in additional options as a second parameter to getOctokit
// const octokit = github.getOctokit(myToken, {userAgent: "MyActionVersion1"});
const { data: pullRequest } = await octokit.rest.pulls.get({
owner: 'octokit',
repo: 'rest.js',
pull_number: 123,
mediaType: {
format: 'diff'
}
});
console.log(pullRequest);
}
run();
You can also make GraphQL requests. See https://github.com/octokit/graphql.js for the API.
const result = await octokit.graphql(query, variables);
Finally, you can get the context of the current action:
const github = require('@actions/github');
const context = github.context;
const newIssue = await octokit.rest.issues.create({
...context.repo,
title: 'New issue!',
body: 'Hello Universe!'
});
The npm module @octokit/webhooks-definitions
provides type definitions for the response payloads. You can cast the payload to these types for better type information.
First, install the npm module npm install @octokit/webhooks-definitions
Then, assert the type based on the eventName
import * as core from '@actions/core'
import * as github from '@actions/github'
import {PushEvent} from '@octokit/webhooks-definitions/schema'
if (github.context.eventName === 'push') {
const pushPayload = github.context.payload as PushEvent
core.info(`The head commit is: ${pushPayload.head_commit}`)
}
@octokit/core
now supports the plugin architecture. You can extend the GitHub instance using plugins.
For example, using the @octokit/plugin-enterprise-server
you can now access enterprise admin apis on GHES instances.
import { GitHub, getOctokitOptions } from '@actions/github/lib/utils'
import { enterpriseServer220Admin } from '@octokit/plugin-enterprise-server'
const octokit = GitHub.plugin(enterpriseServer220Admin)
// or override some of the default values as well
// const octokit = GitHub.plugin(enterpriseServer220Admin).defaults({userAgent: "MyNewUserAgent"})
const myToken = core.getInput('myToken');
const myOctokit = new octokit(getOctokitOptions(token))
// Create a new user
myOctokit.rest.enterpriseAdmin.createUser({
login: "testuser",
email: "testuser@test.com",
});
FAQs
Actions github lib
The npm package @actions/github receives a total of 1,246,534 weekly downloads. As such, @actions/github popularity was classified as popular.
We found that @actions/github demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.