Adonis Ace :triangular_ruler:
Ace is powerful command line to create command line applications in Node.js and extensively used by Adonis framework.
This repo contains the code to use and build ace commands.

What's in the box?
- Colorful help screen.
- Manage and register commands via
Es6 classes
. - Lean and simple API.
- Inbuilt prompts.
- Namespaced commands
- Colorful messages via chalk.
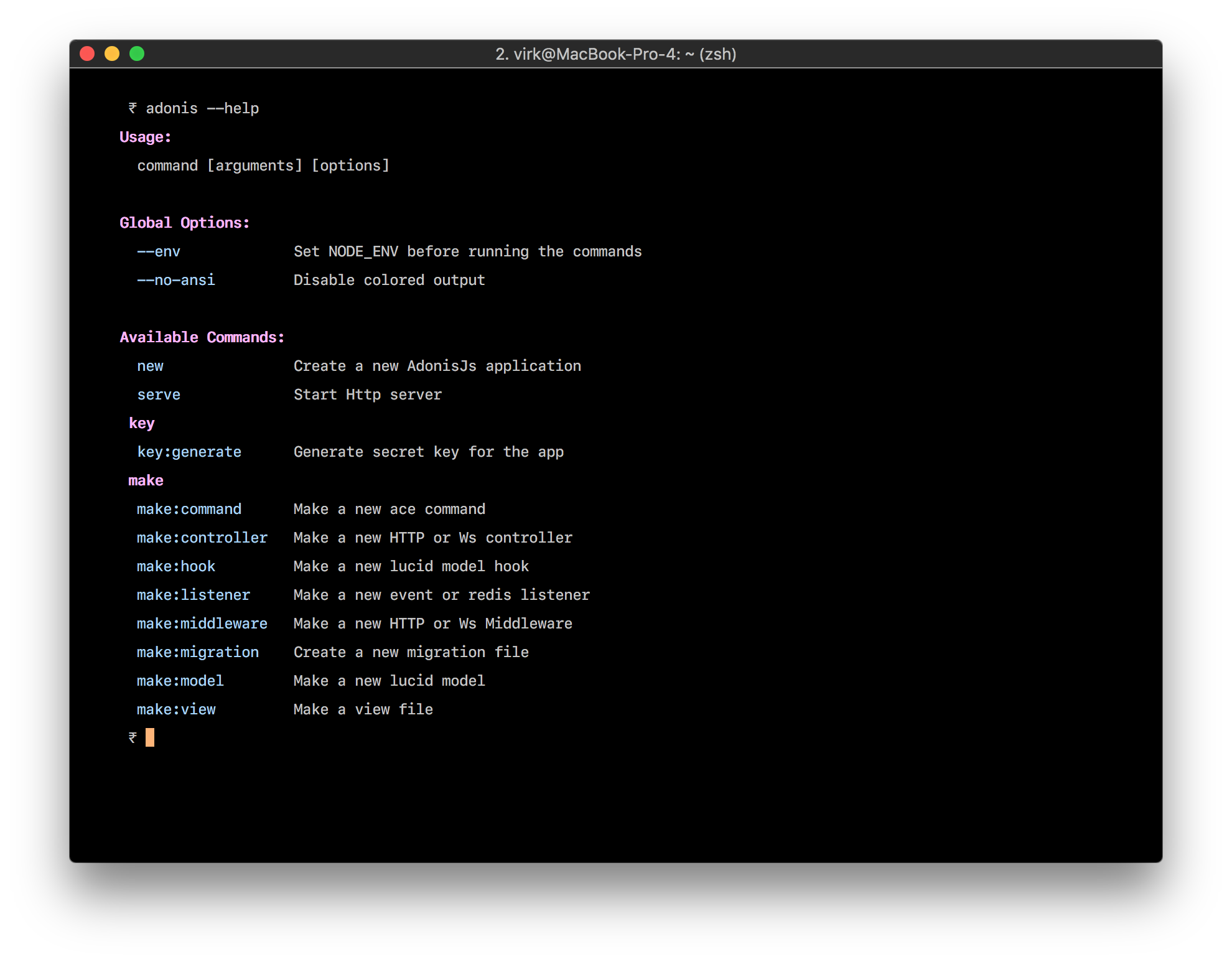
Setup
npm i --save @adonisjs/ace
Next create index.js
file.
const ace = require('@adonisjs/ace')
ace.command(
'greet {name: Name of the user to greet}',
'Command description',
function ({ name }) {
console.log(`Hello ${name}`)
}
)
ace.wireUpWithCommander()
ace.invoke()
The command method expects three arguments as follows.
- signature: The command signature to define the command name and the expected/required inputs.
- description: The command description
- callback Callback to run when command is executed. The callback will receive an object of
inputs
and options
.
Command as classes
Ace has first-class support for registering commands by passing Es6 classes
.
Let's create a new command inside Greet.js
file.
const { Command } = require('@adonisjs/ace')
class Greet extends Command {
static get signature () {
return 'greet {name: Name of the user to greet}'
}
static get description () {
return 'Command description'
}
async handle ({ name }) {
console.log(`Hello ${name}`)
}
}
module.exports = Greet
Next is to register the command.
const ace = require('@adonisjs/ace')
ace.addCommand(require('./Greet'))
ace.wireUpWithCommander()
ace.invoke()
Error handling
You can also listen for errors thrown by commands and display them the way you want.
const ace = require('@adonisjs/ace')
ace.addCommand(require('./Greet'))
ace.onError(function (error, commandName) {
console.log(`${commandName} reported ${error.message}`)
process.exit(1)
})
ace.wireUpWithCommander()
ace.invoke()
Node/OS Target
This repo/branch is supposed to run fine on all major OS platforms and targets Node.js >=7.0
Development
Great! If you are planning to contribute to the framework, make sure to adhere to following conventions, since a consistent code-base is always joy to work with.
Run the following command to see list of available npm scripts.
npm run
Tests & Linting
- Lint your code using standardJs. Run
npm run lint
command to check if there are any linting errors. - Make sure you write tests for all the changes/bug fixes.
- Also you can write regression tests, which shows that something is failing but doesn't breaks the build. Which is actually a nice way to show that something fails. Regression tests are written using
test.failing()
method. - Make sure all the tests are passing on
travis
and appveyor
.
General Practices
Since Es6 is in, you should strive to use latest features. For example:
- Use
Spread
over arguments
keyword. - Never use
bind
or call
. After calling these methods, we cannot guarantee the scope of any methods and in AdonisJs codebase we do not override the methods scope. - Make sure to write proper docblock.
Issues & PR
It is always helpful if we try to follow certain practices when creating issues or PR's, since it will save everyone's time.
- Always try creating regression tests when you find a bug (if possible).
- Share some context on what you are trying to do, with enough code to reproduce the issue.
- For general questions, please create a forum thread.
- When creating a PR for a feature, make sure to create a parallel PR for docs too.
Documentation
You can learn more about ace in the official documentation