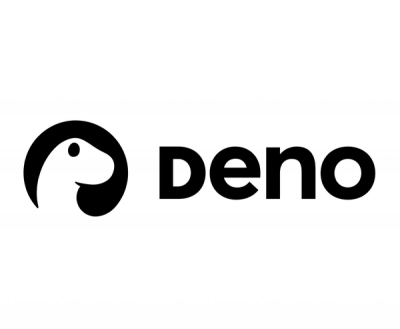
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@aircall/tractor
Advanced tools
The Aircall Tractor design system Foundations.
npm install --save @aircall/tractor
yarn add @aircall/tractor
import { ThemeProvider, Spacer, Typography } from '@aircall/tractor';
const App = () => (
<ThemeProvider>
<Spacer space="s">
<Typography variant="displayM">Hello</Typography>
<Typography variant="displayL">World</Typography>
</Spacer>
</ThemeProvider>
);
You must wrap your React Tree components with the ThemeProvider
component otherwise it will throw an error.
There is no need to import the styles of the components because they are going to be injected for you at runtime.
You first need to clone the repository locally:
$ git clone git@bitbucket.org:aircall/tractor.git
$ cd tractor
$ yarn
$ yarn start:storybook
If you want to test this Design System with your project, you need to:
$ cd tractor
$ yarn link
$ yarn build:watch
$ cd path/to/your/project
$ yarn link "@aircall/tractor"
Make sure that your project is using a single version of React otherwise you will end up having errors:
In the real world, we usually have to modify default webpack config for custom needs. We can achieve that by using craco which is one of create-react-app's custom config solutions.
Install craco and modify the scripts field in package.json
.
$ npm install --save-dev @craco/craco
$ yarn add --dev @craco/craco
/* package.json */
"scripts": {
- "start": "react-scripts start",
- "build": "react-scripts build",
- "test": "react-scripts test",
+ "start": "craco start",
+ "build": "craco build",
+ "test": "craco test",
}
Then create a craco.config.js
at root directory of your project for further overriding.
/* craco.config.js */
const path = require('path');
module.exports = {
webpack: {
alias: {
react: path.resolve('./node_modules/react')
}
}
};
/* webpack.config.js */
const path = require('path');
module.exports = {
resolve: {
alias: {
react: path.resolve('./node_modules/react')
}
}
};
We decided to use commitlint which is a small library that enforces the conventional commit format.
In general the pattern mostly looks like this:
type(scope?): subject #scope is optional
Real world examples can look like this:
chore: run tests on travis ci
fix(spacer): fix the margin on the small variant
feat(button): implement new button component
We recommend to use the type of the commit followed by the component name if possible and then followed when the actual commit message.
We also encourage developers to split as much as possible their commits into small chunks so that it easy for us to review.
Common types according to commitlint-config-conventional can be:
When a or several PRs are merged into master, standard-version will generate the changelog and the bump the version of the package depending on all the commits between the previous tags and the latest commit on master.
Publishing the repository to NPM is a matter of clicking on the Bitbucket Pipeline Publish Button.
FAQs
UI Component Library for Modern Design
The npm package @aircall/tractor receives a total of 2,867 weekly downloads. As such, @aircall/tractor popularity was classified as popular.
We found that @aircall/tractor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.