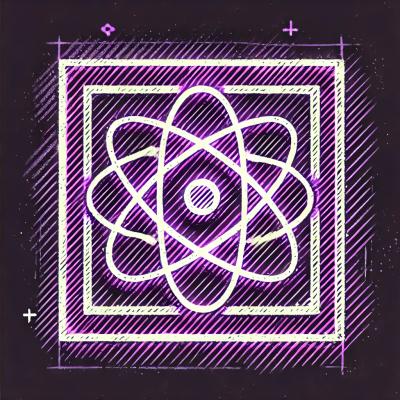
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@airma/react-state
Advanced tools
@airma/react-state
is a simple state management tool for react app. You can visit the document for details.
It works like that:
import React from 'react';
import {render} from 'react-dom'
import {useModel} from '@airma/react-state';
function App(){
const {count, increase, decrease} = useModel((state:number)=>{
const baseState = state >= 0? state : 0;
return {
count: baseState,
increase(){
return baseState + 1;
},
decrease(){
return baseState - 1;
}
};
},0);
return (
<div>
<button onClick={decrease}>-</button>
<span>{count}</span>
<button onClick={increase}>+</button>
</div>
);
}
render(<App/>, document.getElementById('root'));
API useModel
can generate an instance
object by calling a model
function with its default parameter. Call instance
method can generate a new parameter, and then make instance
to refresh itself by recalling the newest model
and parameter again.
It looks like useReducer
, but more free for usage, you can forget dispatch
now.
To make a state change sharable, you need to create a factory
, and use it on a ModelProvider
, then use useModel
or useSelector
to link it.
import React,{memo} from 'react';
import {render} from 'react-dom'
import {
ModelProvider,
useModel,
useSelector,
factory
} from '@airma/react-state';
const counter = (count:number = 0) =>{
return {
count,
isNegative: count<0,
increase:()=>count+1,
decrease:()=>count-1
};
};
// Use API `factory` to build a model factory.
// It can be used as a `function` key for state link.
// A factory is not a store, it only carries a default state.
const couterFactory = factory(counter);
const Increase = memo(()=>{
// use API `useSelector` to fetch the `increase` method.
// The method from instance is persistent,
// so, the none props memo component will not rerender.
const increase = useSelector(couterFactory, c=>c.increase);
return (
<button onClick={increase}>+</button>
);
});
const Decrease = memo(()=>{
// same as the usage in `Increase`
const decrease = useSelector(couterFactory, c=>c.decrease);
return (
<button onClick={decrease}>-</button>
);
});
const CountValue = memo(()=>{
// use API `useModel` with a factory can link the store state.
const {count,isNegative} = useModel(couterFactory);
return (
<span style={isNegative?{color:'red'}:undefined}>{count}</span>
);
});
function Counter({index}:{index:number}){
return (
<div>
counter:{index}
{/* ModelProvider can hold factories, */}
{/* and create a instance store in it, */}
{/* then we can use factory as key to fetch instance */}
<ModelProvider value={couterFactory}>
<div>
<Decrease/>
<CountValue/>
<Increase/>
</div>
</ModelProvider>
</div>
);
}
render(<Counter index={1}/>, document.getElementById('root'));
As you can see, when click the button in Decrease/Increase component, the CountValue changes.
You can reuse your model in a controlled customized hook or component by useControlledModel
.
import React,{memo} from 'react';
import {
useModel,
useControlledModel
} from '@airma/react-state';
const counter = (count:number = 0) =>{
return {
count,
isNegative: count<0,
increase:()=>count+1,
decrease:()=>count-1
};
};
const useControlledCounter = (
value:number,
onChange:(v:number)=>void
)=>{
return useControlledModel(counter, value, onChange);
};
const App = memo(()=>{
const [state, setState] = useState(0);
// use outside `state <-> setState`
const {
count,
isNegative,
increase,
decrease,
} = useControlledCounter(state, setState);
......
});
Make a tuple instance model:
import {useModel} from '@airma/react-state';
const toggleModel = (visible:boolean):[boolean, ()=>boolean]=>[
visible,
()=>!visible
];
......
const [visible, toggle] = useModel(toggleModel, false);
If you want to know more about @airma/react-state
, take the document detail now.
We support the browsers:
chrome: '>=58',
edge: '>=16',
firefox: '=>57',
safari: '>=11'
If you want to support less version browsers, you'd better have your own polyfills.
We hope you can enjoy this tool, and help us to enhance it in future.
FAQs
the purpose of this project is make useReducer more simplify
We found that @airma/react-state demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.