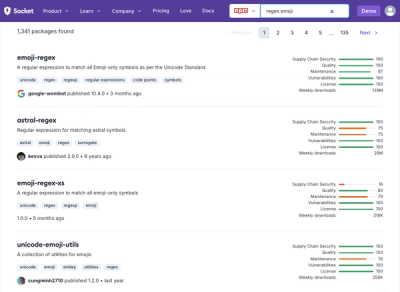
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
@alicloud/kms-sdk
Advanced tools
npm install @alicloud/kms-sdk
Node.js >= 8.5.0 required.
const KmsClient = require('@alicloud/kms-sdk');
const client = new KmsClient({
endpoint: 'kms.cn-hangzhou.aliyuncs.com', // check this from kms console
accessKeyId: '***************', // check this from aliyun console
accessKeySecret: '***************', // check this from aliyun console
});
Credentials file example (~/.alibabacloud/credentials):
[default]
enable = true
type = access_key
access_key_id = ******
access_key_secret = ******
[kms-demo]
enable = true
type = ram_role_arn
access_key_id = ******
access_key_secret = ******
role_arn = acs:ram::******:role/******
role_session_name = ******
Actually @alicloud/credentials will automatically load credentials from the credentials file above.
Client example:
const KmsClient = require('@alicloud/kms-sdk');
const Credentials = require('@alicloud/credentials');
const client = new KmsClient({
endpoint: 'kms.cn-hangzhou.aliyuncs.com', // check this from kms console
credential: new Credentials({ profile: 'kms-demo' })
});
Similarly, we also support setting explicit credentials file path like:
const KmsClient = require('@alicloud/kms-sdk');
const Credentials = require('@alicloud/credentials');
const client = new KmsClient({
endpoint: 'kms.cn-hangzhou.aliyuncs.com', // check this from kms console
credential: new Credentials({
credentialsFile: '/path/to/your/credential'
profile: 'kms-demo'
})
});
Please see @alicloud/credentials docs for more information.
async function demo() {
// describe regions
const regions = await client.describeRegions();
console.log(`regions: ${JSON.stringify(regions)}`);
// create key
const creation = await client.createKey('Aliyun_KMS', `demo`, 'ENCRYPT/DECRYPT');
const keyId = creation.KeyMetadata.KeyId;
console.log(`creation: ${JSON.stringify(creation)}`);
// list keys
const keys = await client.listKeys(1, 100);
console.log(`keys: ${JSON.stringify(keys)}`);
// describe key
const description = await client.describeKey(keyId);
console.log(`description: ${JSON.stringify(description)}`);
// encrypt
const plaintext = 'hello kms sdk for node.js';
const encrypt = await client.encrypt(keyId, plaintext.toString('base64'), JSON.stringify({ k: 'v' }));
const blob = encrypt.CiphertextBlob;
console.log(`description: ${JSON.stringify(description)}`);
// decrypt
const decrypt = await client.decrypt(blob, JSON.stringify({ k: 'v' }));
const rawtext = decrypt.Plaintext;
console.log(`rawtext: ${rawtext}`);
// disable key
const disable = await client.disableKey(keyId);
console.log(`disable: ${JSON.stringify(disable)}`);
// enable key
const enable = await client.enableKey(keyId);
console.log(`enable: ${JSON.stringify(enable)}`);
// generate local data key
const generateKey = await client.generateDataKey(keyId);
console.log(`generateKey: ${JSON.stringify(generateKey)}`);
// get params for import
const res = await client.createKey('EXTERNAL');
const externalKeyId = res.KeyMetadata.KeyId;
const params = await client.getParametersForImport(externalKeyId, 'RSAES_OAEP_SHA_256', 'RSA_2048');
const importTokean = res1.ImportToken;
console.log(`import params: ${JSON.stringify(params)}`);
// import key material
const importKey = await client.importKeyMaterial(externalKeyId, 'test'.toString('base64'), importTokean, Date.now() + 24 * 60 * 60 * 1000);
console.log(`import key: ${JSON.stringify(importKey)}`);
// delete key material
const deleteKeyMaterial = await client.deleteKeyMaterial(externalKeyId);
console.log(`delete key material: ${JSON.stringify(deleteKeyMaterial)}`);
// schedule delete key
const deletion = await client.scheduleKeyDeletion(keyId, 7);
console.log(`deletion: ${JSON.stringify(deletion)}`);
// cancel deletion
const cancel = await client.cancelKeyDeletion(keyId);
console.log(`cancel: ${JSON.stringify(cancel)}`);
// create alias
const alias = `alias/demo`;
const createAlias = await client.createAlias(keyId, alias);
console.log(`createAlias: ${JSON.stringify(createAlias)}`);
// update alias
const creation1 = await client.createKey('Aliyun_KMS', `demo`, 'ENCRYPT/DECRYPT');
const keyId1 = creation1.KeyMetadata.KeyId;
const alias1 = `alias/demo1`;
await client.createAlias(keyId, alias1);
const updateAlias = await client.updateAlias(keyId1, alias1);
console.log(`updateAlias: ${JSON.stringify(updateAlias)}`);
// list aliases
const listAlias = await client.listAliases(1, 100);
console.log(`listAlias: ${JSON.stringify(listAlias)}`);
// list alias by id
const listAliasById = await client.listAliasesByKeyId(keyId, 1, 100);
console.log(`listAliasById: ${JSON.stringify(listAliasById)}`);
// delete alias
const deleteAlias = await client.deleteAlias(alias);
console.log(`deleteAlias: ${JSON.stringify(deleteAlias)}`);
}
demo();
describeRegions()
createKey(origin, description, keyUsage)
listKeys(pageNumber, pageSize)
describeKey(keyId)
encrypt(keyId, plaintext, encryptionContext)
decrypt(ciphertextBlob, encryptionContext)
disableKey(keyId)
enableKey(keyId)
generateDataKey(keyId, keySpec, numberOfBytes, encryptionContex)
getParametersForImport(keyId, wrappingAlgorithm, wrappingKeySpec)
importKeyMaterial(keyId, encryptedKeyMaterial, importToken, keyMaterialExpireUnix)
deleteKeyMaterial(keyId)
scheduleKeyDeletion(keyId, pendingWindowInDays)
cancelKeyDeletion(keyId)
createAlias(keyId, aliasName)
updateAlias(keyId, aliasName)
listAliases(pageNumber, pageSize)
listAliasesByKeyId(keyId, pageNumber, pageSize)
deleteAlias(aliasName)
You should set environment variables before running the test or coverage. For example:
ACCESS_KEY=<your access key> SECRET_KEY=<your secret key> ENDPOINT=<endpoint> npm run test
ACCESS_KEY=<your access key> SECRET_KEY=<your secret key> ENDPOINT=<endpoint> npm run cov
FAQs
alibaba cloud kms client for node.js
The npm package @alicloud/kms-sdk receives a total of 33 weekly downloads. As such, @alicloud/kms-sdk popularity was classified as not popular.
We found that @alicloud/kms-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 9 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.