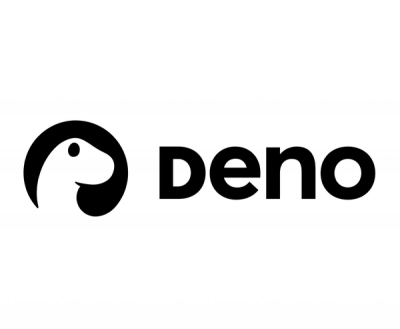
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@alipay/faas-biz-web-sdk
Advanced tools
@alipay/faas-biz-web-sdk 可以在 Web 端(例如 PC Web 页面、H5 等)使用 Javascript 访问云开发用户身份服务。
可以通过 npm 安装:
npm i @alipay/faas-biz-web-sdk
参数:
字段 | 类型 | 必填 | 说明 |
---|---|---|---|
envId | string | 是 | 云开发的 envId |
userPoolUid | string | 是 | 云开发 UserPool 的 UID |
persistence | string | 否 | 登录成功后会返回AccessToken+RefreshToken,通过 persistence 配置这两个 token 的存储位置,默认为 session: |
UserPool 是在云开发创建出来的,会分配一个 Uid,一般一个应用使用一个 UserPool 即可,云开发也支持创建多个。 示例代码:
import { Auth } from "@alipay/faas-biz-web-sdk";
const auth = new Auth({
envId: "your-env-id",
userPoolUid: "your-userpool-uid",
persistence: "local"
});
接口功能:用户名密码登录 输入参数:
字段 | 类型 | 必填 | 说明 |
---|---|---|---|
idpUserId | string | 是 | 用户UID |
password | string | 是 | 密码 |
返回结果:
字段 | 类型 | 不为空 | 说明 |
---|---|---|---|
user | User | 是 | 用户信息 |
loginType | string | 是 | 登录方式 |
isAlipayAuth | boolean | 是 | 支付宝三方登录 |
isUsernameAuth | boolean | 是 | 账密登录 |
示例代码:
import { Auth } from "@alipay/faas-biz-web-sdk";
const userPoolConfig = {
envId: 'your-env-id',
userPoolUid: 'your-userPool-uid',
};
const auth = new Auth(userPoolConfig);
auth.loginWithUsernameAndPassword(username, password).then((res) => {
// 登录成功
});
接口功能:获取 HTTP 鉴权头部,如果未登录,则返回 null。 输入参数:无 返回结果:
字段 | 类型 | 不为空 | 说明 |
---|---|---|---|
x-faas-context-authorization | string | 是 | 鉴权头部信息 |
示例代码:
import { Auth } from "@alipay/faas-biz-web-sdk";
const userPoolConfig = {
envId: 'your-env-id',
userPoolUid: 'your-userPool-uid',
};
const auth = new Auth(userPoolConfig);
auth.getAuthHeader().then((ah) => {
//获取鉴权头部成功
});
接口功能:获取当前登录用户对象,如果未登录,则返回 null。 输入参数:无 返回结果:
字段 | 类型 | 不为空 | 说明 |
---|---|---|---|
user | User | 是 | 用户对象 |
示例代码:
import { Auth } from "@alipay/faas-biz-web-sdk";
const userPoolConfig = {
envId: 'your-env-id',
userPoolUid: 'your-userPool-uid',
};
const auth = new Auth(userPoolConfig);
auth.getCurrentUser()
.then((res) => {
//获取当前登录用户对象成功
});
接口功能:退出登录 输入参数:无 返回结果:无 示例代码:
import { Auth } from "@alipay/faas-biz-web-sdk";
const userPoolConfig = {
envId: 'your-env-id',
userPoolUid: 'your-userPool-uid',
};
const auth = new Auth(userPoolConfig);
auth.logout();
接口功能:跳转到支付宝登录页面 输入参数:
字段 | 类型 | 必填 | 说明 |
---|---|---|---|
appId | string | 是 | 商户的 appId |
返回结果:无 示例代码:
import { Auth } from "@alipay/faas-biz-web-sdk";
const userPoolConfig = {
envId: 'your-env-id',
userPoolUid: 'your-userPool-uid',
};
const auth = new Auth(userPoolConfig);
const authProvider = auth.getAlipayAuthProvider();
authProvider.loginWithRedirect({
appId: 'your-app-id'
});
接口功能:支付宝登录页面重定向回来后,使用重定向的返回值登录,并获取登录态。 输入参数:
字段 | 类型 | 必填 | 说明 |
---|---|---|---|
createUser | boolean | 否 | 当支付宝 openid 没有对应的云开发用户时,是否自动创建一个新的云开发用户,默认为 true |
返回结果:
字段 | 类型 | 不为空 | 说明 |
---|---|---|---|
user | User | 是 | 用户信息 |
loginType | string | 是 | 登录方式 |
isAlipayAuth | boolean | 是 | 支付宝三方登录 |
isUsernameAuth | boolean | 是 | 账密登录 |
示例代码:
import { Auth } from "@alipay/faas-biz-web-sdk";
const userPoolConfig = {
envId: 'your-env-id',
userPoolUid: 'your-userPool-uid',
};
const auth = new Auth(userPoolConfig);
const authProvider = auth.getAlipayAuthProvider();
authProvider.getRedirectResult()
.then((res) => {
//登录成功
});
FAQs
支付宝云开发业务 SDK(WEB 端)
The npm package @alipay/faas-biz-web-sdk receives a total of 0 weekly downloads. As such, @alipay/faas-biz-web-sdk popularity was classified as not popular.
We found that @alipay/faas-biz-web-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.