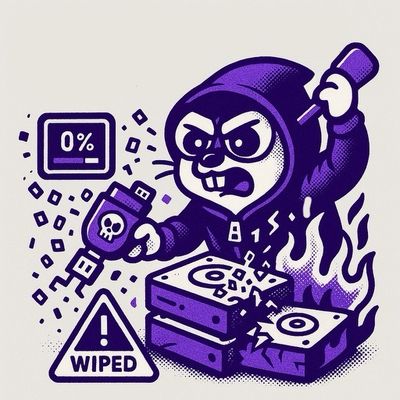
Research
wget to Wipeout: Malicious Go Modules Fetch Destructive Payload
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
@aller/cyclops-frontend-api
Advanced tools
API-part of the cyclops-frontend. This package contains all non-DOM changes, and interactions with CYCLOPS, from login, to payment-initiations, to user-fetching and whatnot. Below are more content and info on functions, schemas, interfaces that are being used in this package..
This package used to use Ajv for response-validation of the json schema. This package, it's validation vs unvalidated functions and tests have been removed to allow the client more controll of the response.
When fetching a user through /users/me
a cyclops_seg
cookie is set, if the user has adSegments
as a property.
Please note that this library is still in active development..
Interfaces can be found in ./src/**/interface.ts
.
If you are not afraid of bloat, this package can be used with @aller/cyclops-frontend-api
.
If you are afraid of bloat, everything can be referenced with @aller/cyclops-frontend-api/lib/node|client/**
(e.g. @aller/cyclops-frontend-api/lib/user/login
). In this, you'll only include the parts of code you actually need, instead of the whole package.
For packages that require node-like environment-transpiled code, point to the lib/node
folder, while client-side code should point to lib/client
.
/**
* Get the current (possibly-cached) user based on
* the cyclops-session cookie
* Use this function if you want to check if a user is logged.
*/
async getCurrentUser(
domain: string = '',
options?: any
): Promise<IUser>
/**
* Get the current non-cached user based on the
* cyclops-session cookie
* This function has a performance cost and should only be used
* if the exact state of the user is required (minside)
*/
async getCannonicalUser(
domain: string = '',
options?: any
): Promise<IUser>
/**
* Sends a subscribe request to cyclops
*/
async subscribe(
productId: string,
accessCode: string,
dealId: string,
options?: any,
): Promise<boolean>
/**
* Get the current (possibly-cached) user
* based on the cyclops-session cookie
* Use this function if you want to check if a user is logged.
*/
async unsubscribe(
productId: string,
domain: string = '',
options?: any
): Promise<boolean>
/**
* Initiates a payment for a product, and deal
*/
async initiatePayment(
productId: string,
dealId: string,
options: IPaymentOptions = defaultPaymentOptions,
domain: string = '',
fetchOptions?: any
): Promise<IPaymentInitResponse>
/**
* Gets from cyclops
*/
async cyclopsGet<T>(
path: string,
options?: any,
absoluteUrl?: boolean
): Promise<T>
/**
* Posts to cyclops
*/
async cyclopsPost<T>(
path: string,
data: any,
options: any = {},
version?: string,
): Promise<T> =>
/**
* Sends a delete request to cyclops
*/
async cyclopsDelete<T>(
path: string,
data: any,
options: any = {},
): Promise<T>
/**
* Initiates a cyclops login redirect. Redirect parameter
* says whether to add a redirect url
*/
cyclopsLogin(
redirect?: string,
domain: string = '',
options?: any
)
/**
* Initiates a cyclops logout
*/
async cyclopsLogout(
refresh: boolean = false,
domain: string = '',
options?: any
)
The listener-system is to allow for listening to requests being made to certain endpoints, in case you want a reactive component, be reactive on a separate component without a bunch of hacks
import { addListener, removeListener } from '@aller/cyclops-frontend-api/lib/client/cyclops/listener'
const listenerFunction = (event:CustomEvent) => {
if(!event.detail) {
return
}
DO YOUR STUFF HERE
}
addListener(ENDPOINT_URL_GOES_HERE, listenerFunction)
// Handle event, then remove
removeListener(ENDPOINT_URL_GOES_HERE, listenerFunction)
/**
* Gets catalogue and validates the response
* to be valid ICatalogue
*/
async getCatalogue(
domain: string = '',
options?: any
): Promise<ICatalogue>
/**
* Creates relative loginURL
*/
export const cyclopsLoginRelativeURL = (
redirect?: string,
domain: string = '',
options?: any,
): string => {
const red = redirect ? redirect : window.location.pathname;
const relativeUrl = `${domain}${CYCLOPS_API}/login?cyclops-redirect=${red}`;
return relativeUrl;
};
/**
* Creates relative loginURL without utilizing any window
* or document elements
*/
export const cyclopsLoginRelativeURLServer = (
redirect?: string,
domain: string = '',
options?: any,
): string => {
const red = redirect ? `?cyclops-redirect=${redirect}` : '';
const relativeUrl = `${domain}${CYCLOPS_API}/${red}`;
return relativeUrl;
};
interface IUser {
readonly cyclopsId: string;
readonly mediaConnectId: string;
readonly aid: string;
readonly email: string;
readonly fullName: string;
readonly phoneNumber: string;
readonly subscriptions?: ReadonlyArray<ISubscription>;
readonly newsletters?: ReadonlyArray<INewsletter>;
readonly legacySubscriptions?: ReadonlyArray<ILegacySubscription>;
readonly paymentHistory: IPaymentHistory;
readonly adSegments?: ReadonlyArray<string>;
readonly consents?: ReadonlyArray<IConsentType>;
readonly newsletterPreferences?: ReadonlyArray<INewsLetterPreference>;
readonly mobileSubscriptions?: IMobileSubscription[];
}
interface IBaseSubscription {
readonly productId: string;
readonly status: 'ACTIVE' | 'CANCELLED';
}
interface ISubscription extends IBaseSubscription {
readonly endDate?: string;
readonly subscriptionId?: string;
readonly startDate?: string;
readonly nextChargeDate?: string;
readonly chargeAmount?: number;
readonly chargeInterval?: string;
readonly dealId?: string;
}
interface ILegacySubscription extends IBaseSubscription {}
interface IMobileSubscription extends IBaseSubscription {
readonly endDate?: string;
readonly subscriptionId?: string;
readonly startDate?: string;
readonly dealId?: string;
readonly dataGb?: string;
readonly phoneNumber?: string;
readonly signupDate?: string;
readonly chargeAmount?: number;
readonly chargeInterval?: string;
}
interface INewsletter {
readonly newsletterId: string;
readonly site: string;
}
interface IProduct {
readonly productName: string;
readonly productDescription: string;
readonly productId: string;
readonly productLogo: string;
readonly productType: 'digital' | 'print';
readonly deals: ReadonlyArray<IProductDeal>;
}
interface IProductDeal {
readonly dealName: string;
readonly dealDescription: string;
readonly dealId: string;
readonly dealLogo: string;
readonly fullTermsDescription: string;
}
type TPaymentProvider = 'vipps' | 'nets';
interface IPaymentInitRequest {
productId: string;
dealId: string;
embeddedCheckoutUrl: string;
allowUnauthenticatedCheckout: boolean;
paymentProvider?: TPaymentProvider;
redirectURL?: string;
}
interface IPaymentInitResponse {
paymentId: string;
paymentProvider: TPaymentProvider;
reservationId?: string;
confirmationUrl?: string;
}
interface IPaymentOptions {
allowUnauthenticatedCheckout?: boolean;
embeddedCheckoutUrl?: string;
paymentProvider?: TPaymentProvider;
redirectURL?: string;
}
interface ICatalogue {
readonly brands: ReadonlyArray<IBrand>;
}
interface IBrand {
readonly brandName: string;
readonly brandDescription: string;
readonly brandId: string;
readonly brandLogo: string;
readonly products: ReadonlyArray<IProduct>;
}
FAQs
Cyclops frontend API
We found that @aller/cyclops-frontend-api demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 11 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Socket's research uncovers three dangerous Go modules that contain obfuscated disk-wiping malware, threatening complete data loss.
Research
Socket uncovers malicious packages on PyPI using Gmail's SMTP protocol for command and control (C2) to exfiltrate data and execute commands.
Product
We redesigned Socket's first logged-in page to display rich and insightful visualizations about your repositories protected against supply chain threats.