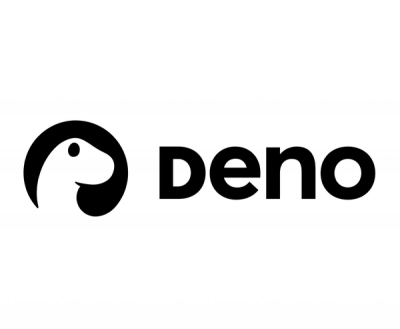
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@alwatr/logger
Advanced tools
Fancy colorful console debugger with custom scope written in tiny TypeScript, ES module.
A lightweight, flexible, and colorful console logging library for TypeScript and ES modules.
npm install @alwatr/logger
import { createLogger } from '@alwatr/logger';
const logger = createLogger('my-module'); // Create a logger with a specific scope
function greet(name: string) {
logger.logMethodArgs?.('greet', { name }); // Log the method call with its arguments
console.log(`Hello, ${name}!`);
}
greet('Ali');
logProperty(propertyName, value)
: Logs a property change (useful for tracking state).logFileModule(fileName)
: Logs the module's file name for easy identification.logMethod(methodName)
: Logs the entry into a function or method.logMethodArgs(methodName, args)
: Logs a method call with its arguments.logStep(methodName, stepName, props?)
: Logs specific steps within a method.logMethodFull(methodName, args, result)
: Logs a method call with arguments and result.incident(methodName, code, ...args)
: Logs an event or expected incident (informational).accident(methodName, code, ...args)
: Logs an unexpected incident or handled error (warning).error(methodName, code, ...args)
: Logs an unexpected error (critical).logOther(...args)
: General-purpose logging with styled scope.time(label)
: Starts a timer.timeEnd(label)
: Ends a timer and logs the elapsed time.banner(message)
: Logs a large, prominent banner message.debug
with the value 1
.Or use the following code snippet in the browser console:
window.localStorage?.setItem('debug', '1');
Note: Ensure the browser console's log level is set to include "Verbose" or "All" to see debug messages.
DEBUG=1 node index.js
The following companies, organizations, and individuals support Nanolib ongoing maintenance and development. Become a Sponsor to get your logo on our README and website.
Contributions are welcome! Please read our contribution guidelines before submitting a pull request.
This project is licensed under the AGPL-3.0 License.
FAQs
Fancy colorful console debugger with custom scope written in tiny TypeScript, ES module.
The npm package @alwatr/logger receives a total of 543 weekly downloads. As such, @alwatr/logger popularity was classified as not popular.
We found that @alwatr/logger demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.