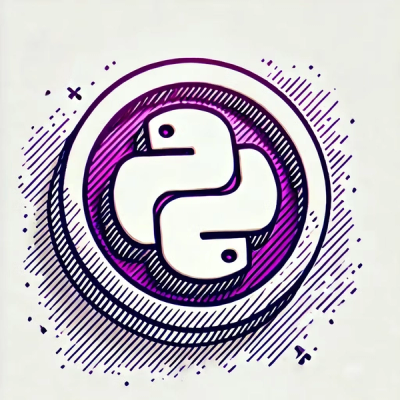
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
@angular-devkit/schematics
Advanced tools
@angular-devkit/schematics is a powerful tool for creating and managing code transformations in Angular projects. It allows developers to automate tasks such as generating components, services, and other Angular constructs, as well as performing code modifications and migrations.
Generating a Component
This feature allows you to generate a new Angular component by creating the necessary TypeScript file with a basic component structure.
const { Rule, SchematicContext, Tree } = require('@angular-devkit/schematics');
function createComponent(options) {
return (tree, _context) => {
const content = `import { Component } from '@angular/core';
@Component({
selector: 'app-${options.name}',
templateUrl: './${options.name}.component.html',
styleUrls: ['./${options.name}.component.css']
})
export class ${options.name.charAt(0).toUpperCase() + options.name.slice(1)}Component { }
`;
tree.create(`/src/app/${options.name}/${options.name}.component.ts`, content);
return tree;
};
}
module.exports = { createComponent };
Updating a File
This feature allows you to update an existing file by replacing specified text with new text.
const { Rule, SchematicContext, Tree } = require('@angular-devkit/schematics');
function updateFile(options) {
return (tree, _context) => {
const filePath = options.path;
if (tree.exists(filePath)) {
const content = tree.read(filePath).toString('utf-8');
const updatedContent = content.replace(options.oldText, options.newText);
tree.overwrite(filePath, updatedContent);
}
return tree;
};
}
module.exports = { updateFile };
Deleting a File
This feature allows you to delete a specified file from the project.
const { Rule, SchematicContext, Tree } = require('@angular-devkit/schematics');
function deleteFile(options) {
return (tree, _context) => {
const filePath = options.path;
if (tree.exists(filePath)) {
tree.delete(filePath);
}
return tree;
};
}
module.exports = { deleteFile };
Yeoman Generator is a scaffolding tool that allows developers to create custom generators for various types of projects. It is more general-purpose compared to @angular-devkit/schematics, which is specifically tailored for Angular projects.
Plop is a micro-generator framework that allows developers to create custom generators for code scaffolding. It is simpler and more lightweight compared to @angular-devkit/schematics, making it suitable for smaller projects or those not specifically tied to Angular.
Hygen is a fast and lightweight code generator that allows developers to create custom templates and generators. It is similar to @angular-devkit/schematics in terms of functionality but is more focused on simplicity and ease of use.
A scaffolding library for the modern web.
Schematics are generators that transform an existing filesystem. They can create files, refactor existing files, or move files around.
What distinguishes Schematics from other generators, such as Yeoman or Yarn Create, is that schematics are purely descriptive; no changes are applied to the actual filesystem until everything is ready to be committed. There is no side effect, by design, in Schematics.
Term | Description |
---|---|
Schematics | A generator that executes descriptive code without side effects on an existing file system. |
Collection | A list of schematics metadata. Schematics can be referred by name inside a collection. |
Tool | The code using the Schematics library. |
Tree | A staging area for changes, containing the original file system, and a list of changes to apply to it. |
Rule | A function that applies actions to a Tree . It returns a new Tree that will contain all transformations to be applied. |
Source | A function that creates an entirely new Tree from an empty filesystem. For example, a file source could read files from disk and create a Create Action for each of those. |
Action | An atomic operation to be validated and committed to a filesystem or a Tree . Actions are created by schematics. |
Sink | The final destination of all Action s. |
Task | A Task is a way to execute an external command or script in a schematic. A Task can be used to perform actions such as installing dependencies, running tests, or building a project. A Task is created by using the SchematicContext object and can be scheduled to run before or after the schematic Tree is applied. |
Schematics is a library, and does not work by itself. A reference CLI is available on this repository, and is published on NPM at @angular-devkit/schematics-cli. This document explains the library usage and the tooling API, but does not go into the tool implementation itself.
The tooling is responsible for the following tasks:
The tooling API is composed of the following pieces:
The SchematicEngine
is responsible for loading and constructing Collection
s and Schematics
. When creating an engine, the tooling provides an EngineHost
interface that understands how to create a CollectionDescription
from a name, and how to create a SchematicDescription
.
Schematics are generators and part of a Collection
.
A Collection is defined by a collection.json
file (in the reference CLI). This JSON defines the following properties:
Prop Name | Type | Description |
---|---|---|
name | string | The name of the collection. |
version | string | Unused field. |
A Source
is a generator of a Tree
; it creates an entirely new root tree from nothing. A Rule
is a transformation from one Tree
to another. A Schematic
(at the root) is a Rule
that is normally applied on the filesystem.
FileOperator
s apply changes to a single FileEntry
and return a new FileEntry
. The result follows these rules:
FileEntry
returned is null, a DeleteAction
will be added to the action list.RenameAction
will be added to the action list.OverwriteAction
will be added to the action list.It is impossible to create files using a FileOperator
.
The Schematics library provides multiple Operator
factories by default that cover basic use cases:
FileOperator | Description |
---|---|
contentTemplate<T>(options: T) | Apply a content template (see the Templating section) |
pathTemplate<T>(options: T) | Apply a path template (see the Templating section) |
The Schematics library additionally provides multiple Source
factories by default:
Source | Description |
---|---|
empty() | Creates a source that returns an empty Tree . |
source(tree: Tree) | Creates a Source that returns the Tree passed in as argument. |
url(url: string) | Loads a list of files from the given URL and returns a Tree with the files as CreateAction applied to an empty Tree . |
apply(source: Source, rules: Rule[]) | Apply a list of Rule s to a source, and return the resulting Source . |
The schematics library also provides Rule
factories by default:
Rule | Description |
---|---|
noop() | Returns the input Tree as is. |
chain(rules: Rule[]) | Returns a Rule that's the concatenation of other Rule s. |
forEach(op: FileOperator) | Returns a Rule that applies an operator to every file of the input Tree . |
move(root: string) | Moves all the files from the input to a subdirectory. |
merge(other: Tree) | Merge the input Tree with the other Tree . |
contentTemplate<T>(options: T) | Apply a content template (see the Template section) to the entire Tree . |
pathTemplate<T>(options: T) | Apply a path template (see the Template section) to the entire Tree . |
template<T>(options: T) | Apply both path and content templates (see the Template section) to the entire Tree . |
filter(predicate: FilePredicate<boolean>) | Returns the input Tree with files that do not pass the FilePredicate . |
As referenced above, some functions are based upon a file templating system, which consists of path and content templating.
The system operates on placeholders defined inside files or their paths as loaded in the Tree
and fills these in as defined in the following, using values passed into the Rule
which applies the templating (i.e. template<T>(options: T)
).
Placeholder | Description |
---|---|
__variable__ | Replaced with the value of variable . |
__variable@function__ | Replaced with the result of the call function(variable) . Can be chained to the left (__variable@function1@function2__ etc). |
Placeholder | Description |
---|---|
<%= expression %> | Replaced with the result of the call of the given expression. This only supports direct expressions, no structural (for/if/...) JavaScript. |
<%- expression %> | Same as above, but the value of the result will be escaped for HTML when inserted (i.e. replacing '<' with '<') |
<% inline code %> | Inserts the given code into the template structure, allowing to insert structural JavaScript. |
<%# text %> | A comment, which gets entirely dropped. |
An example of a simple Schematics which creates a "hello world" file, using an option to determine its path:
import { Tree } from '@angular-devkit/schematics';
export default function MySchematic(options: any) {
return (tree: Tree) => {
tree.create(options.path + '/hi', 'Hello world!');
return tree;
};
}
A few things from this example:
Rule
, which is a transformation from a Tree
to another Tree
.A simplified example of a Schematics which creates a file containing a new Class, using an option to determine its name:
// files/__name@dasherize__.ts
export class <%= classify(name) %> {
}
// index.ts
import { strings } from '@angular-devkit/core';
import {
Rule,
SchematicContext,
SchematicsException,
Tree,
apply,
branchAndMerge,
mergeWith,
template,
url,
} from '@angular-devkit/schematics';
import { Schema as ClassOptions } from './schema';
export default function (options: ClassOptions): Rule {
return (tree: Tree, context: SchematicContext) => {
if (!options.name) {
throw new SchematicsException('Option (name) is required.');
}
const templateSource = apply(url('./files'), [
template({
...strings,
...options,
}),
]);
return branchAndMerge(mergeWith(templateSource));
};
}
Additional things from this example:
strings
provides the used dasherize
and classify
functions, among others.index.ts
and loaded into a Tree
.template
Rule
fills in the specified templating placeholders. For this, it only knows about the variables and functions passed to it via the options-object.Tree
, containing the new file, is merged with the existing files of the project which the Schematic is run on.FAQs
Angular Schematics - Library
The npm package @angular-devkit/schematics receives a total of 8,512,174 weekly downloads. As such, @angular-devkit/schematics popularity was classified as popular.
We found that @angular-devkit/schematics demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.