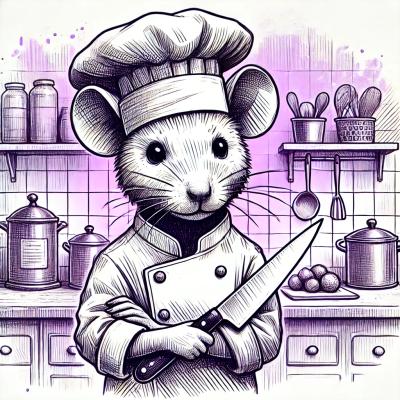
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@ant-design-vue/pro-layout
Advanced tools
Recommend look Examples or Use Template
# yarn
yarn add @ant-design-vue/pro-layout
# npm
npm i @ant-design-vue/pro-layout -S
First, you should add the @ant-design-vue/pro-layout
that you need into the library.
// main.[js|ts]
import "@ant-design-vue/pro-layout/dist/style.css"; // pro-layout css or style.less
import { createApp } from "vue";
import App from "./App.vue";
import Antd from "ant-design-vue";
import ProLayout, { PageContainer } from "@ant-design-vue/pro-layout";
const app = createApp(App);
app.use(Antd).use(ProLayout).use(PageContainer).mount("#app");
After that, you can use pro-layout in your Vue components as simply as this:
<template>
<pro-layout
:locale="locale"
v-bind="layoutConf"
v-model:openKeys="state.openKeys"
v-model:collapsed="state.collapsed"
v-model:selectedKeys="state.selectedKeys"
>
<router-view />
</pro-layout>
</template>
<script setup lang="ts">
import { reactive, useRouter } from "vue";
import { getMenuData, clearMenuItem } from "@ant-design-vue/pro-layout";
const locale = (i18n: string) => i18n;
const router = useRouter();
const { menuData } = getMenuData(clearMenuItem(router.getRoutes()));
const state = reactive({
collapsed: false, // default value
openKeys: ["/dashboard"],
selectedKeys: ["/welcome"],
});
const layoutConf = reactive({
navTheme: "dark",
layout: "mix",
splitMenus: false,
menuData,
});
</script>
Property | Description | Type | Default Value |
---|---|---|---|
title | layout in the upper left corner title | VNode | String | 'Ant Design Pro' |
logo | layout top left logo url | VNode | render | - |
loading | layout loading status | boolean | - |
layout | layout menu mode, sidemenu: right navigation, topmenu: top navigation | 'side' | 'top' | 'mix' | 'side' |
contentWidth | content mode of layout, Fluid: adaptive, Fixed: fixed width 1200px | 'Fixed' | 'Fluid' | Fluid |
navTheme | Navigation theme | 'light' |'dark' | 'light' |
headerTheme | Header Bar theme | 'light' |'dark' | 'light' |
menuData | Vue-router routes prop | Object | [{}] |
collapsed | control menu's collapse and expansion | boolean | true |
selectedKeys | menu selected keys | string[] | [] |
openKeys | menu openKeys | string[] | [] |
isMobile | is mobile | boolean | false |
onCollapse | @collapse | folding collapse event of menu | (collapsed: boolean) => void | - |
menuHeaderRender | render header logo and title | v-slot | VNode | (logo,title)=>VNode | false | - |
menuExtraRender | render extra menu item | v-slot | VNode | (props)=>VNode | false | - |
menuFooterRender | render footer menu item | v-slot | VNode | (props)=>VNode | false | - |
menuItemRender | custom render Menu.Item | v-slot#menuItemRender="{ item, icon }" | ({ item, icon }) => VNode | null |
subMenuItemRender | custom render Menu.SubItem | v-slot#subMenuItemRender="{ item, icon }" | ({ item, icon }) => VNode | null |
collapsedButtonRender | custom collapsed button method | slot | (collapsed: boolean) => VNode | - |
headerRender | custom header render method | slot | (props: BasicLayoutProps) => VNode | - |
headerContentRender | header content render method only layout side | slot | (props: BasicLayoutProps) => VNode | - |
rightContentRender | header right content render method | slot | (props: BasicLayoutProps) => VNode | - |
footerRender | custom footer render method | slot | ({ width, ...BasicLayoutProps }) => VNode | false |
tabRender | custom tab render method | slot | ({ width, ...BasicLayoutProps }) => VNode | false |
breadcrumbRender | custom breadcrumb render method | slot | ({ route, params, routes, paths, h }) => VNode[] | - |
locale | i18n | Function (key: string) => string | false | false |
Menu generation requires
getMenuData
andclearMenuItem
function e.g.const { menuData } = getMenuData(clearMenuItem(routes))
Property | Description | Type | Default Value |
---|---|---|---|
content | Content area | VNode | v-slot | - |
extra | Extra content area, on the right side of content | VNode | v-slot | - |
extraContent | Extra content area, on the right side of content | VNode | v-slot | - |
tabList | Tabs title list | Array<{key: string, tab: sting}> | - |
tab-change | Switch panel callback | (key) => void | - |
tab-active-key | The currently highlighted tab item | string | - |
breadcrumb | Show Bread crumbs bar | Boolean | - |
Property | Description | Type | Default Value |
---|---|---|---|
markStyle | mark style | CSSProperties | - |
markClassName | mark class | string | - |
gapX | Horizontal spacing between water-mark | number | 212 |
gapY | Vertical spacing between watermark | number | 222 |
offsetLeft | Horizontal offset | number | offsetTop = gapX / 2 |
offsetTop | Vertical offset | number | offsetTop = gapY / 2 |
width | number | 120 | |
height | number | 64 | |
rotate | Angle of rotation, unit ° | number | -22 |
image | image src | string | - |
zIndex | water-mark z-index | number | 9 |
content | water-mark Content | string | - |
fontColor | font-color | string | rgba(0,0,0,.15) |
fontSize | font-size | string| number | 16 |
<template #rightContentRender>
<div style="margin-right: 12px">
<a-avatar shape="square" size="small">
<template #icon>
<UserOutlined />
</template>
</a-avatar>
</div>
</template>
<template #menuItemRender="{ item, icon }">
<a-menu-item
:key="item.path"
:disabled="item.meta?.disabled"
:danger="item.meta?.danger"
:icon="icon"
>
<router-link :to="{ path: item.path }">
<span class="ant-pro-menu-item">
<a-badge count="5" dot>
<span class="ant-pro-menu-item-title">{{ item.meta.title }}</span>
</a-badge>
</span>
</router-link>
</a-menu-item>
</template>
<template #menuExtraRender="{ collapsed }">
<a-input-search v-if="!collapsed" />
</template>
<template #menuFooterRender>
<div>menu footer</div>
</template>
<template #breadcrumbRender="{ route, params, routes }">
<span v-if="routes.indexOf(route) === routes.length - 1">
{{ route.breadcrumbName }}
</span>
<router-link v-else :to="{ path: route.path, params }">
{{ route.breadcrumbName }}
</router-link>
</template>
<template #collapsedButtonRender="collapsed">
<HeartOutlined v-if="collapsed" />
<SmileOutlined v-else />
</template>
<template #tabRender="{ width, fixedHeader }">
<div>
<header
class="ant-layout-header"
style="height: 36px; line-height: 36px; background: transparent"
v-if="fixedHeader"
></header>
<div
:style="{
margin: '0',
height: '36px',
lineHeight: '36px',
right: '0px',
top: '48px',
position: fixedHeader ? 'fixed' : 'unset',
zIndex: 14,
padding: '4px 16px',
width: width,
background: '#fff',
boxShadow: '0 1px 4px #0015291f',
transition: 'background 0.3s, width 0.2s',
}"
>
tabRender fixedHeader:{{fixedHeader}} width:{{ width }}
</div>
</div>
</template>
<template #footerRender="{ width, headerTheme }">
<div>
<footer class="ant-layout-footer" style="height: 36px; line-height: 36px; background: transparent"></footer>
<div
:style="{
margin: '0',
height: '36px',
lineHeight: '36px',
right: '0px',
bottom: '0px',
position: headerTheme == 'dark' ? 'fixed' : 'unset',
zIndex: 14,
padding: '4px 16px',
width: width,
background: '#fff',
boxShadow: '0 1px 4px #0015291f',
transition: 'background 0.3s, width 0.2s'
}"
>
footerRender headerTheme:{{ headerTheme }} width:{{ width }}
</div>
</div>
</template>
<GlobalFooter
:links="[
{ title: 'Link 1', href: '#' },
{ title: 'Link 2', href: '#' },
]"
copyright="Pro Layout © 2021 Sendya."
/>
<router-view v-slot="{ Component }">
<WaterMark content="Pro Layout">
<component :is="Component" />
</WaterMark>
</router-view>
pnpm build # Build library and .d.ts
FAQs
Ant Design Pro Layout of Vue, easy to use pro scaffolding.
The npm package @ant-design-vue/pro-layout receives a total of 1,209 weekly downloads. As such, @ant-design-vue/pro-layout popularity was classified as popular.
We found that @ant-design-vue/pro-layout demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.