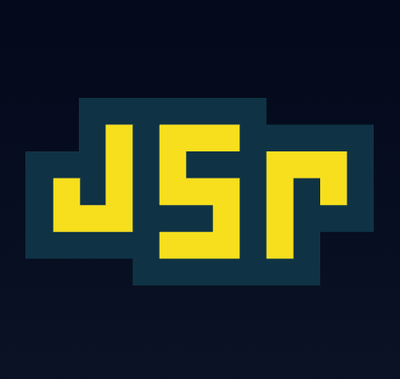
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@antv/util
Advanced tools
@antv/util is a utility library that provides a collection of functions for common programming tasks. It is part of the AntV ecosystem, which is a suite of data visualization tools. The library includes functions for data manipulation, type checking, deep cloning, and more.
Type Checking
The @antv/util package provides various type-checking functions to determine the type of a given value. In this example, `isString` is used to check if a value is a string.
const isString = require('@antv/util/lib/type/isString');
console.log(isString('Hello World')); // true
console.log(isString(123)); // false
Deep Clone
The `deepClone` function creates a deep copy of an object, ensuring that nested objects are also cloned. This is useful for creating independent copies of complex data structures.
const deepClone = require('@antv/util/lib/deepClone');
const obj = { a: 1, b: { c: 2 } };
const clonedObj = deepClone(obj);
console.log(clonedObj); // { a: 1, b: { c: 2 } }
console.log(clonedObj === obj); // false
Data Manipulation
The `groupBy` function allows you to group an array of objects by a specified key. This is useful for organizing data into categories or groups.
const groupBy = require('@antv/util/lib/array/groupBy');
const data = [
{ category: 'fruit', name: 'apple' },
{ category: 'fruit', name: 'banana' },
{ category: 'vegetable', name: 'carrot' }
];
const groupedData = groupBy(data, 'category');
console.log(groupedData); // { fruit: [{ category: 'fruit', name: 'apple' }, { category: 'fruit', name: 'banana' }], vegetable: [{ category: 'vegetable', name: 'carrot' }] }
Lodash is a popular utility library that provides a wide range of functions for data manipulation, type checking, and more. It is widely used and has a large community. Compared to @antv/util, Lodash offers a more extensive set of utilities and is more commonly used in the JavaScript ecosystem.
Underscore is another utility library that offers a variety of functions for common programming tasks. It is similar to Lodash but has a smaller footprint. While @antv/util is part of the AntV ecosystem, Underscore is a standalone library that focuses solely on utility functions.
Ramda is a functional programming library for JavaScript that emphasizes immutability and side-effect-free functions. It provides a different approach compared to @antv/util, focusing on functional programming paradigms. Ramda is ideal for developers who prefer a functional style of coding.
AntV 底层依赖的工具库,不建议在自己业务中使用。
import { gradient } from '@antv/util';
提供以下 Path 工具方法,包含转换、几何计算等。
将 PathArray 转换成字符串形式,不会对原始定义中的命令进行修改:
const str: PathArray = [
['M', 10, 10],
['L', 100, 100],
['l', 10, 10],
['h', 20],
['v', 20],
];
expect(path2String(str)).toEqual('M10 10L100 100l10 10h20v20');
将定义中的相对命令转换成绝对命令,例如:
完整方法签名如下:
path2Absolute(pathInput: string | PathArray): AbsoluteArray;
const str: PathArray = [
['M', 10, 10],
['L', 100, 100],
['l', 10, 10],
['h', 20],
['v', 20],
];
const arr = path2Absolute(str);
expect(arr).toEqual([
['M', 10, 10],
['L', 100, 100],
['L', 110, 110],
['H', 130],
['V', 130],
]);
将部分命令转曲,例如 L / A 转成 C 命令,借助 cubic bezier 易于分割的特性用于实现形变动画。 该方法内部会调用 path2Absolute,因此最终返回的 PathArray 中仅包含 M 和 C 命令。
完整方法签名如下:
path2Curve(pathInput: string | PathArray): CurveArray;
expect(
path2Curve([
['M', 0, 0],
['L', 100, 100],
]),
).toEqual([
['M', 0, 0],
['C', 44.194173824159215, 44.194173824159215, 68.75, 68.75, 100, 100],
]);
复制路径:
const cloned = clonePath(pathInput);
const pathArray: CurveArray = [
['M', 170, 90],
['C', 150, 90, 155, 10, 130, 10],
['C', 105, 10, 110, 90, 90, 90],
['C', 70, 90, 75, 10, 50, 10],
['C', 25, 10, 30, 90, 10, 90],
];
const reversed = reverseCurve(pathArray);
获取几何定义下的包围盒,形如:
export interface PathBBox {
width: number;
height: number;
x: number;
y: number;
x2: number;
y2: number;
cx: number;
cy: number;
cz: number;
}
const bbox = getPathBBox([['M', 0, 0], ['L', 100, 0], ['L', 100, 100], ['L', 0, 100], ['Z']]);
expect(bbox).toEqual({ cx: 50, cy: 50, cz: 150, height: 100, width: 100, x: 0, x2: 100, y: 0, y2: 100 });
获取路径总长度。
const length = getTotalLength([['M', 0, 0], ['L', 100, 0], ['L', 100, 100], ['L', 0, 100], ['Z']]);
expect(length).toEqual(400);
获取路径上从起点出发,到指定距离的点。
const point = getPointAtLength([['M', 0, 0], ['L', 100, 0], ['L', 100, 100], ['L', 0, 100], ['Z']], 0);
expect(point).toEqual({ x: 0, y: 0 });
计算路径包围的面积。内部实现中首先通过 path2Curve 转曲,再计算 cubic curve 面积,详见。
方法签名如下:
function getPathArea(path: PathArray): number;
判断一个点是否在路径上,仅通过几何定义计算,不考虑其他样式属性例如线宽、lineJoin、miter 等。
方法签名如下:
isPointInStroke(pathInput: string | PathArray, point: Point): boolean;
const result = isPointInStroke(segments, { x: 10, y: 10 });
计算两点之间的距离。
方法签名如下:
distanceSquareRoot(a: [number, number], b: [number, number]): number;
将两条路径处理成段数相同,用于形变动画前的分割操作。
const [formattedPath1, formattedPath2] = equalizeSegments(path1, path2);
MIT@AntV.
FAQs
> AntV 底层依赖的工具库,不建议在自己业务中使用。
The npm package @antv/util receives a total of 249,934 weekly downloads. As such, @antv/util popularity was classified as popular.
We found that @antv/util demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 69 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.