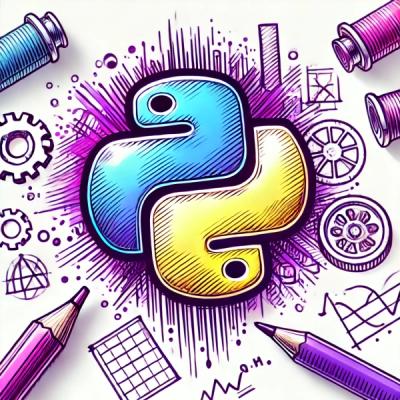
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
@apollo-elements/mixins
Advanced tools
🍹 Moon mixins for cosmic components 👩🚀
A set of class mixin functions that add Apollo GraphQL goodness to your web component classes.
🔎 Read the Full API Docs 🔎
Apollo element mixins are distributed through npm
, the node package manager. To install a copy of the latest version in your project's node_modules
directory, install npm on your system then run the following command in your project's root directory:
npm install --save @apollo-elements/mixins
This is the basic class which all others inherit from. You usually shouldn't need to use this directly.
Connects a web component to apollo client and associates it with a specific GraphQL query. When the query's data updates, so will the element's data
property.
With it, you can create vanilla custom elements that render query data, for example:
Create a template
const template = document.createElement('template');
template.innerHTML = `
<style>
:host([loading]) span {
opacity: 0;
}
span {
opacity: 1;
will-change: opacity;
transition: opacity 0.2s ease-in-out;
}
</style>
<article id="error">
<pre><code></code></pre>
</article>
<p>
<span id="greeting"></span>
<span id="name"></span>
</p>
`;
Define the custom element
import { ApolloQueryMixin } from '@apollo-elements/mixins/apollo-query-mixin.js';
import { gql } from '@apollo/client/core';
class HelloQueryElement extends ApolloQueryMixin(HTMLElement) {
query = gql`
HelloQuery($user: ID, $greeting: String) {
helloWorld(user: $user) {
name
greeting
}
}
`;
variables = {
greeting: "shalom",
user: "haver"
};
constructor() {
super();
this.attachShadow({ mode: 'open' });
this.shadowRoot.append(template.content.cloneNode(true));
}
}
customElements.define('hello-query', HelloQueryElement);
Add reactivity
#data = null;
get data() { return this.#data; }
set data(data) { this.#data = data; this.render(); }
#loading = false;
get loading() { return this.#loading; }
set loading(loading) { this.#loading = loading; this.render(); }
#error = null;
get error() { return this.#error; }
set error(error) { this.#error = error; this.render(); }
Render the data
$(id) { return this.shadowRoot.getElementById(id); }
render() {
if (this.loading)
this.setAttribute('loading', '');
else
this.removeAttribute('loading');
this.$('error').hidden =
!this.error;
this.$('error').querySelector("code").textContent =
this.error?.message ?? '';
this.$('greeting').textContent =
this.data?.helloWorld?.greeting ?? 'Hello';
this.$('name').textContent =
this.data?.helloWorld?.name ?? 'Friend';
}
And use it in HTML
<hello-query></hello-query>
Connects a web component to apollo client and associates it with a specific GraphQL mutation. When the mutation resolves, so will the element's data
property.
Connects a web component to apollo client and associates it with a specific GraphQL subscription. When the subscription gets new data, the element's data
property will update.
Optional mixin which connects an element to a specific ApolloClient
instance.
import { client } from './specific-apollo-client';
class SpecificClientElement
extends ApolloClientMixin(client, ApolloQueryMixin(HTMLElement)) {
// ... do stuff with your client
}
Allows users to set the element's query (or mutation, or subscription) and variables using HTML.
import { ApolloQueryMixin, GraphQLScriptChildMixin } from '@apollo-elements/mixins';
class HelloQueryElement extends ApolloQueryMixin(HTMLElement) { /* ... */ }
customElements.define('hello-query', HelloQueryElement);
<hello-query>
<script type="application/graphql">
query HelloQuery($user: ID, $greeting: String) {
helloWorld(user: $user) {
name
greeting
}
}
</script>
<script type="application/json">
{
"greeting": "shalom",
"user": "haver"
}
</script>
</hello-query>
Optional mixin which prevents queries from automatically subscribing until their non-nullable variables are defined.
Optional mixin which lets you declare type policies for a component's query.
Different kind of mixin. These are JavaScript class mixins, which are essentially function composition.
Looking for other libraries? Want to use Apollo with your favourite custom-elements library? Check out our docs site
apollo-elements
is a community project maintained by Benny Powers.
FAQs
👩🚀🌛 Custom Element class mixins for Apollo GraphQL 🚀👨🚀
The npm package @apollo-elements/mixins receives a total of 128 weekly downloads. As such, @apollo-elements/mixins popularity was classified as not popular.
We found that @apollo-elements/mixins demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.