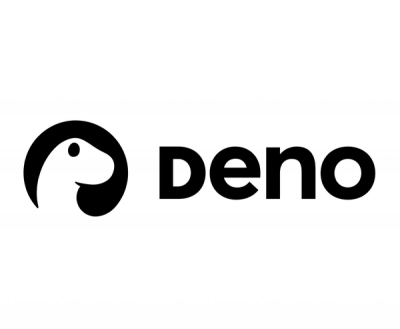
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@apollo/client
Advanced tools
The @apollo/client npm package is a comprehensive state management library for JavaScript that enables you to manage both local and remote data with GraphQL. It is designed to help you develop faster and more secure apps with less boilerplate. It integrates seamlessly with any JavaScript front-end, allowing for reactive data fetching, caching, and data management.
Fetching data with useQuery
This feature allows you to fetch data from a GraphQL server. The useQuery hook is used to execute a GraphQL query and handle loading, error, and result states.
import { useQuery, gql } from '@apollo/client';
const GET_LAUNCHES = gql`
query GetLaunches {
launches {
id
mission_name
}
}
`;
function Launches() {
const { loading, error, data } = useQuery(GET_LAUNCHES);
if (loading) return <p>Loading...</p>;
if (error) return <p>Error :(</p>;
return data.launches.map(({ id, mission_name }) => (
<div key={id}>
<p>{mission_name}</p>
</div>
));
}
Updating data with useMutation
This feature enables you to update data on a GraphQL server. The useMutation hook is used to execute a mutation, allowing you to create, update, or delete data.
import { useMutation, gql } from '@apollo/client';
const ADD_TODO = gql`
mutation AddTodo($type: String!) {
addTodo(type: $type) {
id
type
}
}
`;
function AddTodo() {
let input;
const [addTodo, { data }] = useMutation(ADD_TODO);
return (
<div>
<form
onSubmit={e => {
e.preventDefault();
addTodo({ variables: { type: input.value } });
input.value = '';
}}
>
<input
ref={node => {
input = node;
}}
/>
<button type="submit">Add Todo</button>
</form>
</div>
);
}
Managing local state
This feature allows you to manage local state using Apollo Client. You can read and write to the cache as if it were a local database, enabling you to manage client-side state alongside remote data.
import { gql, useApolloClient } from '@apollo/client';
const GET_LOCAL_STATE = gql`
query GetLocalState {
isLoggedIn @client
}
`;
function LoginButton() {
const client = useApolloClient();
const data = client.readQuery({ query: GET_LOCAL_STATE });
const isLoggedIn = data.isLoggedIn;
return (
<button onClick={() => {
client.writeQuery({
query: GET_LOCAL_STATE,
data: { isLoggedIn: !isLoggedIn }
});
}}>
{isLoggedIn ? 'Log out' : 'Log in'}
</button>
);
}
Relay is a JavaScript framework for building data-driven React applications with GraphQL. It is similar to @apollo/client in that it provides a powerful and efficient way to fetch and manage GraphQL data. Relay focuses on performance optimizations and static query generation, which can make it more suitable for complex, large-scale applications with high performance requirements.
urql is a highly customizable and versatile GraphQL client for React, Vue, Svelte, and other JavaScript frameworks. It offers a simpler and more flexible approach compared to @apollo/client, with features like document caching and subscriptions. urql's extensibility and plugin system make it a good choice for developers looking for a lightweight and adaptable GraphQL client.
Apollo Client is a fully-featured caching GraphQL client with integrations for React, Angular, and more. It allows you to easily build UI components that fetch data via GraphQL.
All Apollo Client documentation, including React integration articles and helpful recipes, can be found at:
https://www.apollographql.com/docs/react/
The Apollo Client API reference can be found at:
https://www.apollographql.com/docs/react/api/apollo-client/
FAQs
A fully-featured caching GraphQL client.
The npm package @apollo/client receives a total of 2,166,922 weekly downloads. As such, @apollo/client popularity was classified as popular.
We found that @apollo/client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.