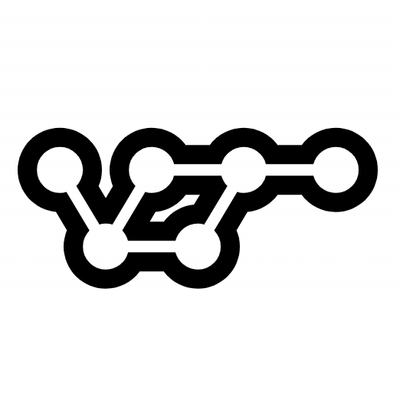
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
@appbaseio/analytics
Advanced tools
A universal analytics library that allows you to register click, conversion and custom events for appbase.io apps.
The analytics library can be either loaded via jsDelivr CDN or directly bundled with your application.
You can use the UMD build in browsers by following the below snippet:
<script defer src="https://cdn.jsdelivr.net/npm/@appbaseio/analytics@1.0.0-alpha.1/dist/@appbaseio/analytics.umd.min.js"
></script>
const aaInstance = aa({
index: 'APP_NAME',
credentials: 'APP_CREDENTIALS'
});
Install the library by following the below command:
npm install @appbaseio/analytics
# or
yarn add @appbaseio/analytics
const aa = require('@appbaseio/analytics');
const aaInstance = aa({
index: 'APP_NAME',
credentials: 'APP_CREDENTIALS'
});
The analytics library provides the utility methods to implement the appbase.io search analytics, the common use-cases are to record the clicks, conversions and apply the search headers to create a search session.
To register the click events you just need to create a search session and get the search id, a search session can be initialized bypassing the x-search-query
header in the _msearch
or _search
request.
We recommend to use getAnalyticsHeaders
method to attach the analytics headers in search requests. For example:
const aa = require('@appbaseio/analytics');
const aaInstance = aa({
index: 'APP_NAME',
credentials: 'APP_CREDENTIALS'
});
const analyticsHeaders = aaInstance
.setSearchQuery('harry')
.getAnalyticsHeaders();
fetch(`https://scalr.api.appbse.io/${APP_NAME}/_search`, {
headers: analyticsHeaders
})
.then(res => {
const searchID = res.headers.get('X-Search-Id');
// Set the search id back to the analytics instance
if (searchID) {
aaInstance.setSearchID(searchID);
}
})
.catch(err => {
console.error(err);
});
Once you get the searchID
back you need to use it to record the analytics events.
Click events can be registered with the help of registerClick
method which has the following signature:
registerClick(
clickPosition: number, // position of the item in the list
isSuggestion?: boolean // whether a click is of type suggestion or results
): Promise<any>
aa()
.setIndex('APP_NAME') // If index is not set during initialization
.setCredentials('APP_CREDENTIALS') // If credentials is not set during initialization
.setSearchID('SEARCH_ID') // SEARCH_ID must be present otherwise the function will throw an error
.registerClick(`CLICK_POSITION`);
aa()
.setIndex('APP_NAME') // If index is not set during initialization
.setCredentials('APP_CREDENTIALS') // If credentials is not set during initialization
.setSearchID('SEARCH_ID') // SEARCH_ID must be present otherwise the function will throw an error
.registerClick(`CLICK_POSITION`, true);
aa()
.setIndex('APP_NAME') // If index is not set during initialization
.setCredentials('APP_CREDENTIALS') // If credentials is not set during initialization
.setSearchID('SEARCH_ID') // SEARCH_ID must be present otherwise the function will throw an error
.registerConversion();
aa().setUserID('harry');
aa().setCustomEvents({
key1: 'value1',
key2: 'value2'
});
aa().setSearchState({
BookSensor: {
dataField: 'original_title',
value: 'harry',
queryFormat: 'or'
}
});
Although this library supports chaining i.e you can set and clear anything whenever you want but you can initialize it at once with some common properties to avoid the duplication.
const aa = require('@appbaseio/analytics');
const aaInstance = aa({
index: 'APP_NAME',
credentials: 'APP_CREDENTIALS'
});
Optional configuration options:
Option | Type | Default | Description |
---|---|---|---|
index | string | None (required) | App name for appbase.io apps or index name in case of Arc or clusters . |
credentials | string | None (required) | API key for appbase.io hosted application. |
url | string | https://scalr.api.appbase.io | Not needed for appbase.io apps but in case of Arc you need to define the arc URL. |
userID | string | null | Sets the userID to be recorded. |
customEvents | object | null | To set the custom events |
headers | object | null | To set the custom headers |
emptyQuery | boolean | true | To define whether to record the empty queries or not |
setIndex(index: string)
To set the index or app name.
setCredentials(credentials: string)
To set the application auth credentials.
setURL(url: string)
To set the URL, required when you use the appbase.io clusters or Arc
.
setHeaders(headers: Object)
It allows you to set the custom headers in analytics endpoints.
setSearchQuery(searchQuery: string)
Sets the search query which is needed to retrieve the search headers.
clearSearchQuery();
Clears the search query
setSearchID(searchID: string)
Sets the search ID which is required to register the analytics events.
clearSearchID();
Clears the searchID
setSearchState(searchState: string)
Sets the search state which will be used to retrieve the search headers.
clearSearchState();
Clears the saved search state.
setUserID(userID: string)
Sets the user ID which will be used to retrieve the search headers.
clearUserID();
Clears the userID
setCustomEvents(customEvents: Object)
Sets the custom events which will override the existing custom events and will be used further in the analytics headers. For example:
aa().setCustomEvents({
platform: 'mac',
user_segment: 'free'
});
clearCustomEvents();
Clears the existing custom events
addCustomEvent(customEvent: Object)
Add a particular custom event in the existing custom events.
aa().addCustomEvent({
platform: 'mac'
});
removeCustomEvent(eventKey: string)
Remove a custom event by key
aa().removeCustomEvent('platform');
setFilters(filters: Object)
Set the filters which will be used to retrieve the search headers.
aa().setFilters({
brand: 'Adidas',
category: 'shoes'
});
clearFilters();
Clear the filters
addFilter(filter: Object)
Adds a filter in the existing filters.
removeFilter(filterKey: string)
Removes a filter by key
enableEmptyQuery();
Enables the recording of empty queries i.e create a search session even if the search query is empty.
disableEmptyQuery();
Disables the recording of empty queries.
registerClick(
clickPosition: number,
isSuggestion?: boolean
): Promise<any>
Registers a click event
registerConversion(): Promise<any>
Registers a search conversion
getAnalyticsHeaders(): Object
Returns a list of search headers based on the analytics state which needs to be applied to the _search
& _msearch
request to create a search session.
Fork the repo and run the following command to run & test locally.
yarn && yarn test
Arc API Gateway for ElasticSearch (Out of the box Security, Rate Limit Features, Record Analytics and Request Logs).
ReactiveSearch ReactiveSearch is an Elasticsearch UI components library for React, React Native and Vue. It has 25+ components consisting of Lists, Ranges, Search UIs, Result displays and a way to bring any existing UI component into the library.
searchbox A lightweight and performance focused searchbox UI libraries to query and display results from your ElasticSearch app (aka index).
Dejavu allows viewing raw data within an appbase.io (or Elasticsearch) app. Soon to be released feature: An ability to import custom data from CSV and JSON files, along with a guided walkthrough on applying data mappings.
Mirage ReactiveSearch components can be extended using custom Elasticsearch queries. For those new to Elasticsearch, Mirage provides an intuitive GUI for composing queries.
ReactiveMaps is a similar project to Reactive Search that allows building realtime maps easily.
appbase-js While building search UIs is dandy with Reactive Search, you might also need to add some input forms. appbase-js comes in handy there.
This library is Apache licensed.
FAQs
Universal analytics library for appbase.io apps
The npm package @appbaseio/analytics receives a total of 2,644 weekly downloads. As such, @appbaseio/analytics popularity was classified as popular.
We found that @appbaseio/analytics demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.