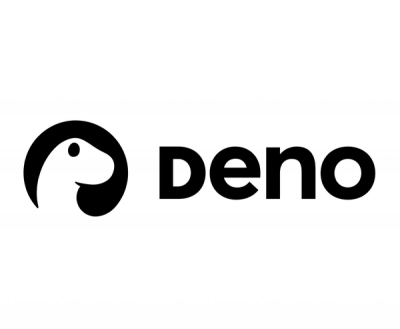
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@appfarm/ttl-cache-redis
Advanced tools
Simple abstraction of redis for storing strings and objects
This is a supersimple abstraction for storing strings and JSON-objects in redis.
Using npm:
$ npm install @appfarm/ttl-cache-redis
const Cache = require('@appfarm/ttl-cache-redis')
const myCache = new Cache({
redisConnectionString: 'redis://localhost:6379',
cacheKeyPrefix: 'MY-CACHE-', // prefix any key with this value
})
// Setting "foo" to MY-CACHE-newkey with a
// lifetime of 10 minutes
myCache
.setStringValue('newkey', 'foo', 600)
.then(() => console.log('Successfully set string value'))
.catch(err => console.error(err))
myCache
.setObjectValue('myobject', { foo: 'bar' }, 300)
.then(() => console.log('Successfully set object value'))
.catch(err => console.error(err))
// Retrieve string value from cache
myCache
.getStringValue(key)
.then(value => {
console.log('Found value:', value)
})
.catch(err => {
console.log('Unable to find value or key has expired')
})
// Retrieve object value from store
myCache
.getObectValue(key)
.then(objectValue => {
console.log('Found object:', objectValue)
})
.catch(err => {
console.log('Unable to find value or key has expired')
})
// Fire and forget - delete value from store immediately
// Returns nothing.
myCache.invalidate('newkey')
FAQs
Simple abstraction of redis for storing strings and objects
The npm package @appfarm/ttl-cache-redis receives a total of 1 weekly downloads. As such, @appfarm/ttl-cache-redis popularity was classified as not popular.
We found that @appfarm/ttl-cache-redis demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.