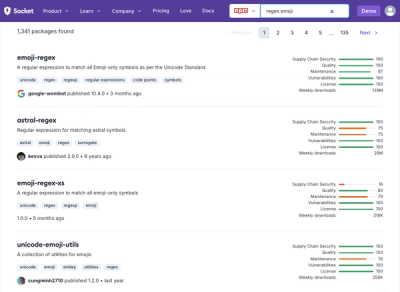
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
@arifzyn/tokopay-client
Advanced tools
A TypeScript client for interacting with the Tokopay payment gateway API. This client provides easy-to-use methods for creating orders, checking order status, and managing your merchant account.
npm install tokopay-ts
# or
yarn add tokopay-ts
import Tokopay from "tokopay-ts";
const tokopay = new Tokopay("YOUR_MERCHANT_ID", "YOUR_SECRET");
const merchantInfo = await tokopay.info();
console.log("Current Balance:", merchantInfo.balance);
Use this method for quick order creation with minimal parameters:
// Simple order creation
const simpleOrder = await tokopay.simpleOrder(
"REF123", // Your unique reference ID
"BRIVA", // Payment method code
25000, // Amount in IDR
);
console.log("Payment URL:", simpleOrder.pay_url);
console.log("Total Amount:", simpleOrder.total_bayar);
Use this method when you need more control over the order details:
// Complete order with all parameters
const orderItems = [
{
product_code: "PROD-001",
name: "Gaming Mouse",
price: 250000,
product_url: "https://yourstore.com/gaming-mouse",
image_url: "https://yourstore.com/images/gaming-mouse.jpg",
},
];
const order = await tokopay.createOrder(
"BRIVA", // Payment channel code
"ORDER-123", // Your unique reference ID
250000, // Amount in IDR
"John Doe", // Customer name
"johndoe@example.com", // Customer email
"081234567890", // Customer phone
"https://yourstore.com/return", // Return URL (optional)
0, // Expiry time (0 for 24h default)
orderItems, // Order items (optional)
);
console.log("Virtual Account:", order.data.nomor_va);
console.log("Payment URL:", order.data.pay_url);
console.log("Amount:", order.data.total_bayar);
Monitor the payment status of your orders:
const orderStatus = await tokopay.checkOrderStatus(
"ORDER-123", // Your reference ID
"BRIVA", // Payment method used
250000, // Order amount
);
console.log("Payment Status:", orderStatus.data.status);
console.log("Payment Instructions:", orderStatus.data.panduan_pembayaran);
console.log("Transaction ID:", orderStatus.data.trx_id);
// Check if payment is completed
if (orderStatus.data.status === "Paid") {
console.log("Payment has been received!");
}
Withdraw funds from your merchant account:
const withdrawal = await tokopay.tarikSaldo(1000000); // Amount in IDR
console.log("Withdrawal Status:", withdrawal.status);
Common payment method codes you can use:
BRIVA
- BRI Virtual AccountBNIVA
- BNI Virtual AccountBSIVA
- BSI Virtual AccountQRIS
- QRIS PaymentDANA
- DANAOVO
- OVOSHOPEEPAY
- ShopeePaySee Docs For Detail Payment Method Tokopay Documentation.
interface OrderStatusResponse {
data: {
other: string;
panduan_pembayaran: string; // Payment instructions
pay_url: string; // Payment page URL
qr_link: string; // QR code image URL (if applicable)
qr_string: string; // QR code string (if applicable)
status: "Unpaid" | "Paid" | "Expired" | "Failed";
total_bayar: number; // Total amount to pay
total_diterima: number; // Amount received after fees
trx_id: string; // Transaction ID
};
status: string;
}
interface OrderResponse {
data: {
nomor_va: string; // Virtual account number
panduan_pembayaran: string; // Payment instructions
pay_url: string; // Payment page URL
total_bayar: number; // Total amount to pay
total_diterima: number; // Amount received after fees
trx_id: string; // Transaction ID
};
status: string;
}
The client includes proper error handling. Here's how to handle potential errors:
try {
const order = await tokopay.createOrder(/* ... */);
// Process successful order
} catch (error) {
console.error("Failed to create order:", error.message);
// Handle error appropriately
}
npm run build
# or
yarn build
npm test
# or
yarn test
For support, please contact support@tokopay.id or visit Tokopay Documentation.
Remember to replace 'YOUR_MERCHANT_ID'
and 'YOUR_SECRET'
with your actual Tokopay credentials.
This project is licensed under the MIT License - see the LICENSE file for details.
FAQs
Simple Tokopay Client Wrapper
We found that @arifzyn/tokopay-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.