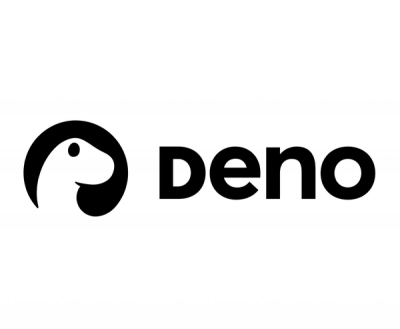
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@arrows/dispatch
Advanced tools
The library contains a collection of dispatch functions. All functions with the arity (number of arguments) greater than one are automatically curried, so a partial application is possible.
The library has built-in type definitions, which provide an excellent IDE support.
Via NPM:
npm i @arrows/dispatch
Via Yarn:
yarn add @arrows/dispatch
All modules can be imported independently (to reduce bundle size), here are some import methods (you can use either CommonJS or ES modules):
import dispatch from '@arrows/dispatch'
import { getType } from '@arrows/dispatch'
import getType from '@arrows/dispatch/getType'
Retrieves the type of a value (using the internal [[Class]]
property).
A more useful alternative for the typeof
operator.
It does not return custom prototypes - if you need that, use the is
function instead.
See: types for the list of the most common values.
See: MDN - Object.prototype.toString.call
value
- Any value(value) => underlying_class_name
getType(1) // -> "Number"
getType(/abc/) // -> "RegExp"
getType([1, 2, 3]) // -> "Array"
Standard identity function - useful as a default dispatch or a placeholder.
value
- Any value(value) => value
identity('foo') // -> "foo"
identity([1, 2, 3]) // -> [1, 2, 3]
Checks if a value is an instance of a prototype/class.
prototype
- A prototype/class with which you want to check the value.value
- Any valuetrue
is a value is an instance of a prototype/class, false
otherwise.(prototype, value) => boolean
class Cat {}
class Dog {}
const cat = new Cat()
const dog = new Dog()
is(Cat, cat) // -> true
is(Dog, cat) // -> false
is(Cat)(cat) // -> true
is(Dog)(cat) // -> false
Checks if a value is inside an array/set.
list
- An array or a set of valuesvalue
- Any valuetrue
is a value is inside an array/set, false
otherwise.(array_or_set, value) => boolean
const names = ['Alice', 'Joe', 'John']
isIn(names, 'Alice') // -> true
isIn(names, 'Bob') // -> false
isIn(names)('Alice') // -> true
isIn(names)('Bob') // -> false
const names = new Set(['Alice', 'Joe', 'John'])
isIn(names, 'Alice') // -> true
isIn(names, 'Bob') // -> false
isIn(names)('Alice') // -> true
isIn(names)('Bob') // -> false
An object that contains a list of the most common types to use with the getType function. You can use it instead of using raw strings (which is error-prone).
getType([1, 2, 3]) === types.Array // -> true
getType(() => null) === types.Function // -> true
getType(Promise.resolve()) === types.Promise // -> true
getType('1') === types.Number // -> false
Project is under open, non-restrictive ISC license.
FAQs
Functional dispatch library
We found that @arrows/dispatch demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.