NuxtHub
The Nuxt Toolkit to create full-stack applications on the Edge.
Features
- Session management with
useAuth(event)
- Query an SQLite database with
useDatabase()
- Access key-value storage with
useKV()
- Store files with
useBlob()
Blob
Upload a blob
const blob = await useBlob().put('my-file.txt', 'Hello World!', {
contentType: 'text/plain'
addRandomSuffix: true
})
Usage with file upload
export default eventHandler(async (event) => {
const form = await readFormData(event)
const file = form.get('file')
return useBlob().put(file.name, file)
})
List blobs
const { blobs, cursor, hasMore } = await useBlob().list({
limit: 10,
prefix: 'my-dir',
})
const blob = useBlob()
let blobs = []
let hasMore = true
let cursor
while (hasMore) {
const result = await blob.list({
cursor,
})
blobs.push(...result.blobs)
hasMore = result.hasMore
cursor = result.cursor
}
Get blob metadata
const blob = await useBlob().head('my-file.txt')
Delete a blob
await useBlob().delete('my-file.txt')
It returns a void response. A delete action is always successful if the blob url exists. A delete action won't throw if the blob url doesn't exists.
Serve a blob
export default eventHandler(event => {
const pathname = event.context.params.pathname
return useBlob().serve(event, pathname)
})
Key-Value Storage
Get a value
const value = await useKV().get('my-key')
Live demos
https://github.com/Atinux/nuxt-todos-edge/assets/904724/5f3bee55-dbae-4329-8057-7d0e16e92f81
Setup
Make sure to install the dependencies using pnpm:
pnpm i
Create a GitHub Oauth Application with:
- Homepage url:
http://localhost:3000
- Callback url:
http://localhost:3000/api/auth/github
Add the variables in the .env
file:
NUXT_OAUTH_GITHUB_CLIENT_ID="my-github-oauth-app-id"
NUXT_OAUTH_GITHUB_CLIENT_SECRET="my-github-oauth-app-secret"
To create sealed sessions, you also need to add NUXT_SESSION_SECRET
in the .env
with at least 32 characters:
NUXT_SESSION_SECRET=your-super-long-secret-for-session-encryption
Development
Start the development server on http://localhost:3000
npm run dev
In the Nuxt DevTools, you can see your tables by clicking on the Drizzle Studio tab:
https://github.com/Atinux/nuxt-todos-edge/assets/904724/7ece3f10-aa6f-43d8-a941-7ca549bc208b
Deploy on CloudFlare Pages
Create a CF pages deployment linked to your GitHub repository. Make sure to select Version 2 (Beta) as the build system version.
Environment variables
NUXT_OAUTH_GITHUB_CLIENT_ID=...
NUXT_OAUTH_GITHUB_CLIENT_SECRET=...
NUXT_SESSION_PASSWORD=...
Build command
Set the build command to:
npm run build
And the output directory to dist/
D1 Database
Lastly, in the project settings -> Functions, add the binding between your D1 database and the DB
variable:
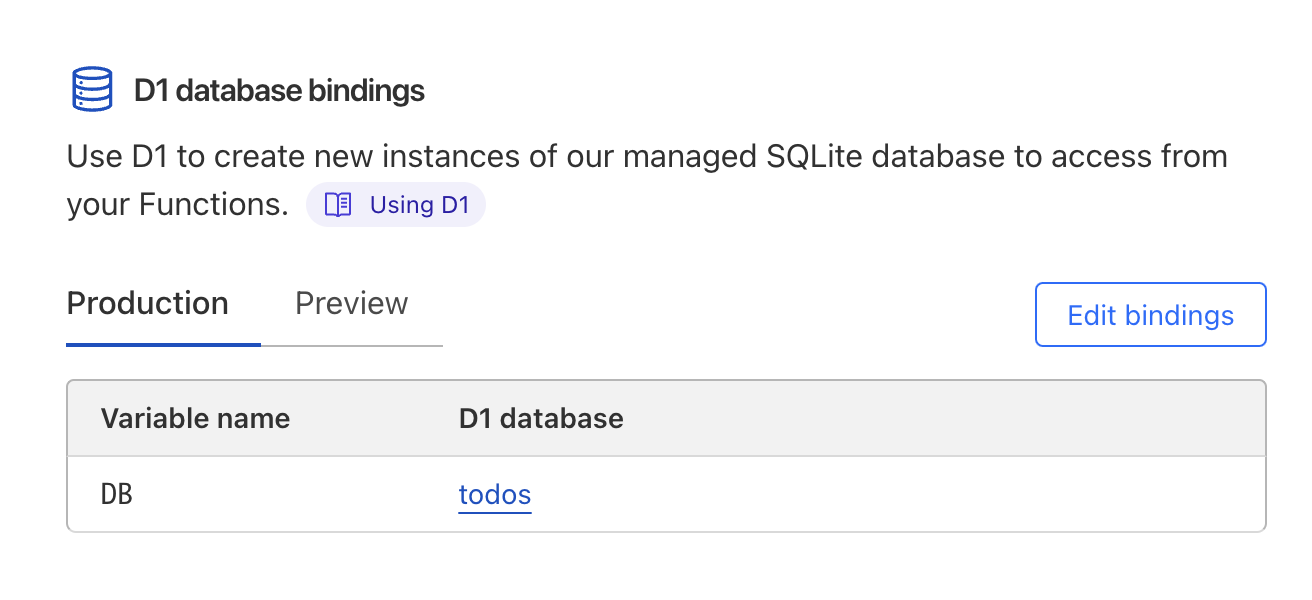
Copy the contents from server/database/migrations/0000_heavy_xorn.sql
into the D1 console to seed the database.
Turso Database
You can also use Turso database instead of CloudFlare D1 by creating a database and adding the following env variables:
TURSO_DB_URL=...
TURSO_DB_TOKEN=...
You can see a live demo using Turso on https://nuxt-todos-turso.pages.dev
License
MIT License