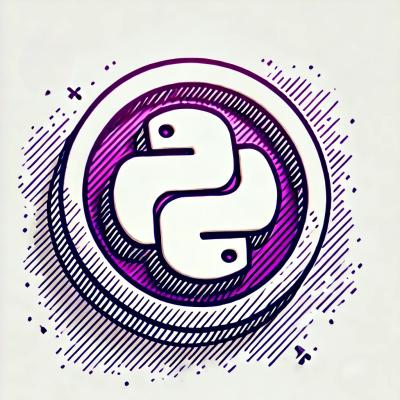
Security News
PyPI Now Supports iOS and Android Wheels for Mobile Python Development
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
@auth0/angular-jwt
Advanced tools
@auth0/angular-jwt is an Angular library that provides utilities for handling JSON Web Tokens (JWT). It helps in managing token-based authentication in Angular applications by providing features such as token decoding, token expiration checking, and HTTP request interception to automatically add JWTs to requests.
Token Decoding
This feature allows you to decode a JWT to access its payload. The JwtHelperService provides a method decodeToken that takes a raw JWT string and returns its decoded payload.
import { JwtHelperService } from '@auth0/angular-jwt';
const helper = new JwtHelperService();
const decodedToken = helper.decodeToken(myRawToken);
console.log(decodedToken);
Token Expiration Checking
This feature allows you to check if a JWT has expired. The JwtHelperService provides a method isTokenExpired that takes a raw JWT string and returns a boolean indicating whether the token is expired.
import { JwtHelperService } from '@auth0/angular-jwt';
const helper = new JwtHelperService();
const isExpired = helper.isTokenExpired(myRawToken);
console.log(isExpired);
HTTP Request Interception
This feature allows you to automatically add JWTs to HTTP requests. By configuring the JwtModule and providing a tokenGetter function, you can intercept HTTP requests and add the JWT to the Authorization header.
import { HttpClientModule, HTTP_INTERCEPTORS } from '@angular/common/http';
import { JwtModule } from '@auth0/angular-jwt';
export function tokenGetter() {
return localStorage.getItem('access_token');
}
@NgModule({
imports: [
HttpClientModule,
JwtModule.forRoot({
config: {
tokenGetter: tokenGetter,
allowedDomains: ['example.com'],
disallowedRoutes: ['example.com/examplebadroute/'],
},
}),
],
providers: [
{ provide: HTTP_INTERCEPTORS, useClass: JwtInterceptor, multi: true },
],
})
export class AppModule {}
angular2-jwt is another Angular library for handling JWTs. It provides similar functionalities such as token decoding, token expiration checking, and HTTP request interception. However, @auth0/angular-jwt is more actively maintained and has better integration with Auth0 services.
ngx-auth is a comprehensive authentication library for Angular that supports JWT. It provides features for token management, HTTP request interception, and route guarding. Compared to @auth0/angular-jwt, ngx-auth offers a more extensive set of features for handling authentication flows.
ngx-jwt-auth is another Angular library focused on JWT authentication. It provides utilities for token storage, token expiration checking, and HTTP request interception. While it offers similar functionalities to @auth0/angular-jwt, it is less popular and has fewer community contributions.
:books: Documentation - :rocket: Getting Started - :computer: API Reference - :speech_balloon: Feedback
This library provides an HttpInterceptor
which automatically attaches a JSON Web Token to HttpClient
requests.
This library does not have any functionality for (or opinion about) implementing user authentication and retrieving JWTs to begin with. Those details will vary depending on your setup, but in most cases, you will use a regular HTTP request to authenticate your users and then save their JWTs in local storage or in a cookie if successful.
This project only supports the actively supported versions of Angular as stated in the Angular documentation. Whilst other versions might be compatible they are not actively supported
# installation with npm
npm install @auth0/angular-jwt
# installation with yarn
yarn add @auth0/angular-jwt
Import the JwtModule
module and add it to your imports list. Call the forRoot
method and provide a tokenGetter
function. You must also add any domains to the allowedDomains
, that you want to make requests to by specifying an allowedDomains
array.
Be sure to import the HttpClientModule
as well.
import { JwtModule } from "@auth0/angular-jwt";
import { HttpClientModule } from "@angular/common/http";
export function tokenGetter() {
return localStorage.getItem("access_token");
}
@NgModule({
bootstrap: [AppComponent],
imports: [
// ...
HttpClientModule,
JwtModule.forRoot({
config: {
tokenGetter: tokenGetter,
allowedDomains: ["example.com"],
disallowedRoutes: ["http://example.com/examplebadroute/"],
},
}),
],
})
export class AppModule {}
Any requests sent using Angular's HttpClient
will automatically have a token attached as an Authorization
header.
import { HttpClient } from "@angular/common/http";
export class AppComponent {
constructor(public http: HttpClient) {}
ping() {
this.http.get("http://example.com/api/things").subscribe(
(data) => console.log(data),
(err) => console.log(err)
);
}
}
If you are using bootstrapApplication
to bootstrap your application using a standalone component, you will need a slightly different way to integrate our SDK:
import { JwtModule } from "@auth0/angular-jwt";
import { provideHttpClient, withInterceptorsFromDi } from "@angular/common/http";
export function tokenGetter() {
return localStorage.getItem("access_token");
}
bootstrapApplication(AppComponent, {
providers: [
// ...
importProvidersFrom(
JwtModule.forRoot({
config: {
tokenGetter: tokenGetter,
allowedDomains: ["example.com"],
disallowedRoutes: ["http://example.com/examplebadroute/"],
},
}),
),
provideHttpClient(
withInterceptorsFromDi()
),
],
});
As you can see, the differences are that:
importProvidersFrom
.provideHttpClient
needs to be called with withInterceptorsFromDi
.Read our API reference to get a better understanding on how to use this SDK.
We appreciate feedback and contribution to this repo! Before you get started, please see the following:
To provide feedback or report a bug, please raise an issue on our issue tracker.
Please do not report security vulnerabilities on the public GitHub issue tracker. The Responsible Disclosure Program details the procedure for disclosing security issues.
Auth0 is an easy to implement, adaptable authentication and authorization platform. To learn more checkout Why Auth0?
This project is licensed under the MIT license. See the LICENSE file for more info.
FAQs
JSON Web Token helper library for Angular
The npm package @auth0/angular-jwt receives a total of 178,894 weekly downloads. As such, @auth0/angular-jwt popularity was classified as popular.
We found that @auth0/angular-jwt demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 44 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PyPI now supports iOS and Android wheels, making it easier for Python developers to distribute mobile packages.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.