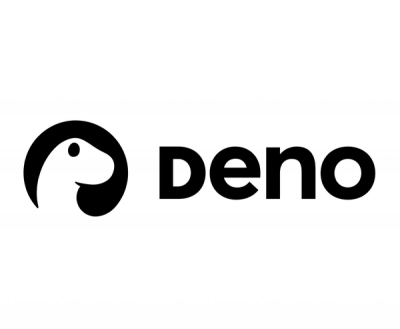
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@autonomys/auto-utils
Advanced tools
The Autonomys Auto SDK Utility (@autonomys/auto-utils
) provides core utility functions for interacting with the Autonomys Network. It offers functionalities for:
This package serves as the foundational layer for building applications within the Autonomys ecosystem.
Install the package via npm or yarn:
# Using npm
npm install @autonomys/auto-utils
# Using yarn
yarn add @autonomys/auto-utils
Below are examples demonstrating how to use the functions provided by @autonomys/auto-utils
.
Activate a wallet using a mnemonic phrase:
// Import necessary functions
import { activateWallet } from '@autonomys/auto-utils'
;(async () => {
// Replace with your mnemonic
const mnemonic = 'your mnemonic phrase here'
// Activate the wallet
const { api, accounts } = await activateWallet({
mnemonic,
networkId: 'gemini-3h', // Optional: specify the network ID
})
const account = accounts[0]
console.log(`Connected with account address: ${account.address}`)
// Perform actions with the account...
// Disconnect when done
await api.disconnect()
})()
Parameters:
mnemonic
(string): The mnemonic phrase of the wallet.networkId
(string, optional): The ID of the network to connect to.Returns:
api
: An instance of ApiPromise
connected to the network.accounts
: An array of accounts derived from the mnemonic.You can also activate a wallet using a URI (e.g., //Alice
for development purposes):
import { activateWallet } from '@autonomys/auto-utils'
;(async () => {
const { api, accounts } = await activateWallet({
uri: '//Alice',
networkId: 'localhost', // Connect to a local network
})
const account = accounts[0]
console.log(`Connected with account address: ${account.address}`)
// Disconnect when done
await api.disconnect()
})()
Create mock wallets for testing purposes:
import { activate, mockWallets, getMockWallet } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'gemini-3h' })
const wallets = await mockWallets({}, api)
const aliceWallet = getMockWallet('Alice', wallets)
const bobWallet = getMockWallet('Bob', wallets)
console.log(`Alice's address: ${aliceWallet.accounts[0].address}`)
console.log(`Bob's address: ${bobWallet.accounts[0].address}`)
// Disconnect when done
await api.disconnect()
})()
List all available networks:
import { networks } from '@autonomys/auto-utils'
networks.forEach((network) => {
console.log(`Network ID: ${network.id}, Name: ${network.name}`)
})
Retrieve details of a specific network:
import { getNetworkDetails } from '@autonomys/auto-utils'
const network = getNetworkDetails({ networkId: 'gemini-3h' })
console.log(`Network Name: ${network.name}`)
console.log(`RPC URLs: ${network.rpcUrls.join(', ')}`)
Retrieve details of a specific domain within a network:
import { getNetworkDomainDetails } from '@autonomys/auto-utils'
const domain = getNetworkDomainDetails({ domainId: '1', networkId: 'gemini-3h' })
console.log(`Domain Name: ${domain.name}`)
console.log(`RPC URLs: ${domain.rpcUrls.join(', ')}`)
Hash a string using BLAKE2b-256:
import { blake2b_256, stringToUint8Array } from '@autonomys/auto-utils'
const data = 'Hello, Autonomys!'
const dataBytes = stringToUint8Array(data)
const hash = blake2b_256(dataBytes)
console.log(`Hash: ${hash}`) // Outputs the hash of the input string
Convert a string to a Uint8Array
:
import { stringToUint8Array } from '@autonomys/auto-utils'
const text = 'Sample text'
const byteArray = stringToUint8Array(text)
console.log(byteArray) // Outputs Uint8Array representation of the string
Concatenate two Uint8Array
instances:
import { stringToUint8Array, concatenateUint8Arrays } from '@autonomys/auto-utils'
const array1 = stringToUint8Array('First part ')
const array2 = stringToUint8Array('Second part')
const concatenated = concatenateUint8Arrays(array1, array2)
console.log(`Concatenated Result: ${new TextDecoder().decode(concatenated)}`)
// Outputs: "First part Second part"
Connect to the Autonomys Network:
import { activate } from '@autonomys/auto-utils'
;(async () => {
const api = await activate({ networkId: 'gemini-3h' })
console.log('API connected')
// Perform API calls...
// Disconnect when done
await api.disconnect()
})()
Connect to a specific domain within the network:
import { activateDomain } from '@autonomys/auto-utils'
;(async () => {
const api = await activateDomain({ domainId: '1', networkId: 'gemini-3h' })
console.log('Domain API connected')
// Perform domain-specific API calls...
// Disconnect when done
await api.disconnect()
})()
Save data to local storage or the file system and read it back:
import { save, read } from '@autonomys/auto-utils'
const key = 'myData'
const value = { message: 'Hello, Autonomys!' }
// Save data
save(key, value)
// Read data
const retrievedValue = read(key)
console.log(retrievedValue) // Outputs: { message: 'Hello, Autonomys!' }
Convert an address to a standardized format and decode it:
import { address, decode } from '@autonomys/auto-utils'
const originalAddress = '5GmS1wtCfR4tK5SSgnZbVT4kYw5W8NmxmijcsxCQE6oLW6A8'
const standardizedAddress = address(originalAddress)
const decodedAddress = decode(originalAddress)
console.log(`Standardized Address: ${standardizedAddress}`)
console.log(`Decoded Address:`, decodedAddress)
setupWallet(params: MnemonicOrURI): KeyringPair
: Initializes a wallet using a mnemonic or URI.
Parameters:
params
(object):
mnemonic
(string, optional): The mnemonic phrase.uri
(string, optional): The derivation path or URI.Returns: KeyringPair
- The initialized wallet key pair.
activateWallet(params: ActivateWalletParams): Promise<WalletActivated>
: Activates a wallet and returns API and accounts.
Parameters:
params
(object):
mnemonic
or uri
(string): Wallet credentials.networkId
(string, optional): The network ID to connect to.Returns:
api
: Connected ApiPromise
instance.accounts
: Array of derived accounts.mockWallets(network: NetworkParams | DomainParams, api: ApiPromise): Promise<WalletActivated[]>
: Creates mock wallets for testing.
Parameters:
network
(object): Network parameters.api
(ApiPromise
): Connected API instance.Returns: Array of WalletActivated
- Mock wallets.
getMockWallet(name: string, wallets: WalletActivated[]): WalletActivated
: Retrieves a mock wallet by name.
Parameters:
name
(string): Name of the mock wallet (e.g., 'Alice', 'Bob').wallets
(array): Array of WalletActivated
.Returns: A single WalletActivated
matching the name.
getNetworkDetails(input?: NetworkParams): Network
: Gets network details.
Parameters:
input
(object, optional): Contains networkId
.Returns: Network
- Network configuration object.
getNetworkRpcUrls(input?: NetworkParams): string[]
: Gets network RPC URLs.
Parameters:
input
(object, optional): Contains networkId
.Returns: Array of RPC URL strings.
getNetworkDomainDetails(params: DomainParams): Domain
: Gets domain details.
Parameters:
params
(object): Contains domainId
and networkId
.Returns: Domain
- Domain configuration object.
getNetworkDomainRpcUrls(params: DomainParams): string[]
: Gets domain RPC URLs.
Parameters:
params
(object): Contains domainId
and networkId
.Returns: Array of domain RPC URL strings.
save(key: string, value: any): void
: Saves data to local storage or file system.
Parameters:
key
(string): Unique identifier for the data.value
(any): Data to be stored.Returns: void
read(key: string): any
: Reads data from local storage or file system.
Parameters:
key
(string): Unique identifier for the data.Returns: The retrieved data.
blake2b_256(data: Uint8Array): string
: Hashes data with BLAKE2b-256.
Parameters:
data
(Uint8Array
): Data to be hashed.Returns: Hex string representation of the hash.
stringToUint8Array(text: string): Uint8Array
: Converts a string to a Uint8Array
.
Parameters:
text
(string): The input string.Returns: Uint8Array
representation of the string.
concatenateUint8Arrays(array1: Uint8Array, array2: Uint8Array): Uint8Array
: Concatenates two Uint8Array
instances.
Parameters:
array1
(Uint8Array
)array2
(Uint8Array
)Returns: New Uint8Array
resulting from concatenation.
activate(params?: ActivateParams<NetworkParams>): Promise<ApiPromise>
: Activates the API for a network.
Parameters:
params
(object, optional): Network activation parameters.Returns: ApiPromise
instance connected to the network.
activateDomain(params: ActivateParams<DomainParams>): Promise<ApiPromise>
: Activates the API for a domain.
Parameters:
params
(object): Domain activation parameters.Returns: ApiPromise
instance connected to the domain.
disconnect(api: ApiPromise): Promise<void>
: Disconnects the API.
Parameters:
api
(ApiPromise
): The API instance to disconnect.Returns: void
address(address: string | Uint8Array): string
: Converts an address to a standardized format.
Parameters:
address
(string | Uint8Array
): The original address.Returns: Standardized address string.
decode(address: string): Uint8Array
: Decodes an address into a Uint8Array
.
Parameters:
address
(string): The address to decode.Returns: Uint8Array
representation of the address.
networks
: Array of network configurations.
import { networks } from '@autonomys/auto-utils'
// Example usage
networks.forEach((network) => {
console.log(network.id, network.name)
})
defaultNetwork
: Default network configuration.
import { defaultNetwork } from '@autonomys/auto-utils'
console.log(`Default Network: ${defaultNetwork.name}`)
mockURIs
: Array of mock URIs.
import { mockURIs } from '@autonomys/auto-utils'
console.log(`Available mock URIs: ${mockURIs.join(', ')}`)
Network
, Domain
, Explorer
, NetworkParams
, DomainParams
Mnemonic
, URI
, AppName
, MnemonicOrURI
These types are available for TypeScript users to ensure type safety and better development experience.
When using @autonomys/auto-utils
, it's important to handle potential errors, especially when dealing with asynchronous operations like network connections or wallet activations. Make use of try/catch
blocks or handle promise rejections appropriately.
Example:
import { activateWallet } from '@autonomys/auto-utils'
;(async () => {
try {
const { api, accounts } = await activateWallet({
mnemonic: 'your mnemonic',
})
// Proceed with using the api and accounts
} catch (error) {
console.error('Error activating wallet:', error)
}
})()
We welcome community contributions! If you wish to contribute to @autonomys/auto-utils
, please follow these guidelines:
Fork the repository on GitHub.
Clone your fork locally:
git clone https://github.com/your-username/auto-sdk.git
cd auto-sdk/packages/auto-utils
Install dependencies:
yarn install
Make your changes and ensure all tests pass:
yarn test
Commit your changes with clear and descriptive messages.
Push to your fork and create a pull request against the main
branch of the original repository.
yarn lint
to ensure code style consistency.This project is licensed under the MIT License. See the LICENSE file for details.
If you have any questions or need support, feel free to reach out:
We appreciate your feedback and contributions!
FAQs
Unknown package
We found that @autonomys/auto-utils demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.