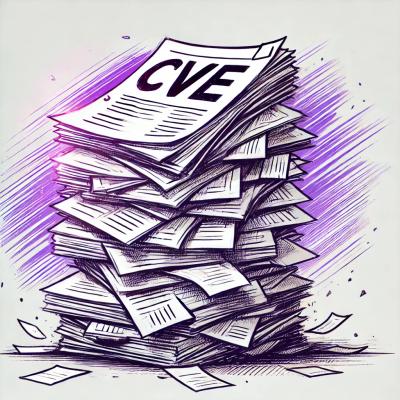
Security News
Node.js EOL Versions CVE Dubbed the "Worst CVE of the Year" by Security Experts
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
@aws-amplify/graphql-api-construct
Advanced tools
AppSync GraphQL Api Construct using Amplify GraphQL Transformer.
This package vends an L3 CDK Construct wrapping the behavior of the Amplify GraphQL Transformer. This enables quick development and interation of AppSync APIs which support the Amplify GraphQL Directives. For more information on schema modeling in GraphQL, please refer to the amplify developer docs.
The primary way to use this construct is to invoke it with a provided schema (either as an inline graphql string, or as one or more appsync.SchemaFile
) objects, and with authorization config provided. There are 5 supported methods for authorization of an AppSync API, all of which are supported by this construct. For more information on authorization rule definitions in Amplify, refer to the authorization docs. Note: currently at least one authorization rule is required, and if multiple are specified, a defaultAuthorizationMode
must be specified on the api as well. Specified authorization modes must be a superset of those configured in the graphql schema.
In this example, we create a single model, which will use user pool
auth in order to allow logged in users to create and manage their own todos
privately.
We create a cdk App and Stack, though you may be deploying this to a custom stack, this is purely illustrative for a concise demo.
We then wire this through to import a user pool which was already deployed (creating and deploying is out of scope for this example).
import { App, Stack } from 'aws-cdk-lib';
import { UserPool } from 'aws-cdk-lib/aws-cognito';
import { AmplifyGraphqlApi, AmplifyGraphqlDefinition } from '@aws-amplify/graphql-api-construct';
const app = new App();
const stack = new Stack(app, 'TodoStack');
new AmplifyGraphqlApi(stack, 'TodoApp', {
definition: AmplifyGraphqlDefinition.fromString(/* GraphQL */ `
type Todo @model @auth(rules: [{ allow: owner }]) {
description: String!
completed: Boolean
}
`),
authorizationModes: {
userPoolConfig: {
userPool: UserPool.fromUserPoolId(stack, 'ImportedUserPool', '<YOUR_USER_POOL_ID>'),
},
},
});
In this example, we create a two related models, which will use which logged in users in the 'Author' and 'Admin' user groups will have full access to, and customers requesting with api key will only have read permissions on.
import { App, Stack } from 'aws-cdk-lib';
import { UserPool } from 'aws-cdk-lib/aws-cognito';
import { AmplifyGraphqlApi, AmplifyGraphqlDefinition } from '@aws-amplify/graphql-api-construct';
const app = new App();
const stack = new Stack(app, 'BlogStack');
new AmplifyGraphqlApi(stack, 'BlogApp', {
definition: AmplifyGraphqlDefinition.fromString(/* GraphQL */ `
type Blog @model @auth(rules: [{ allow: public, operations: [read] }, { allow: groups, groups: ["Author", "Admin"] }]) {
title: String!
description: String
posts: [Post] @hasMany
}
type Post @model @auth(rules: [{ allow: public, operations: [read] }, { allow: groups, groups: ["Author", "Admin"] }]) {
title: String!
content: [String]
blog: Blog @belongsTo
}
`),
authorizationModes: {
defaultAuthorizationMode: 'API_KEY',
apiKeyConfig: {
description: 'Api Key for public access',
expires: cdk.Duration.days(7),
},
userPoolConfig: {
userPool: UserPool.fromUserPoolId(stack, 'ImportedUserPool', '<YOUR_USER_POOL_ID>'),
},
},
});
In this example, we import the schema definition itself from one or more local files, rather than an inline graphql string.
# todo.graphql
type Todo @model @auth(rules: [{ allow: owner }]) {
content: String!
done: Boolean
}
# blog.graphql
type Blog @model @auth(rules: [{ allow: owner }, { allow: public, operations: [read] }]) {
title: String!
description: String
posts: [Post] @hasMany
}
type Post @model @auth(rules: [{ allow: owner }, { allow: public, operations: [read] }]) {
title: String!
content: [String]
blog: Blog @belongsTo
}
// app.ts
import { App, Stack } from 'aws-cdk-lib';
import { UserPool } from 'aws-cdk-lib/aws-cognito';
import { AmplifyGraphqlApi, AmplifyGraphqlDefinition } from '@aws-amplify/graphql-api-construct';
const app = new App();
const stack = new Stack(app, 'MultiFileStack');
new AmplifyGraphqlApi(stack, 'MultiFileDefinition', {
definition: AmplifyGraphqlDefinition.fromFiles(path.join(__dirname, 'todo.graphql'), path.join(__dirname, 'blog.graphql')),
authorizationModes: {
defaultAuthorizationMode: 'API_KEY',
apiKeyConfig: {
description: 'Api Key for public access',
expires: cdk.Duration.days(7),
},
userPoolConfig: {
userPool: UserPool.fromUserPoolId(stack, 'ImportedUserPool', '<YOUR_USER_POOL_ID>'),
},
},
});
L3 Construct which invokes the Amplify Transformer Pattern over an input Graphql Schema.
This can be used to quickly define appsync apis which support full CRUD+List and Subscriptions, relationships, auth, search over data, the ability to inject custom business logic and query/mutation operations, and connect to ML services.
For more information, refer to the docs links below: Data Modeling - https://docs.amplify.aws/cli/graphql/data-modeling/ Authorization - https://docs.amplify.aws/cli/graphql/authorization-rules/ Custom Business Logic - https://docs.amplify.aws/cli/graphql/custom-business-logic/ Search - https://docs.amplify.aws/cli/graphql/search-and-result-aggregations/ ML Services - https://docs.amplify.aws/cli/graphql/connect-to-machine-learning-services/
For a full reference of the supported custom graphql directives - https://docs.amplify.aws/cli/graphql/directives-reference/
The output of this construct is a mapping of L2 or L1 resources generated by the transformer, which generally follow the access pattern
const api = new AmplifyGraphQlApi(this, 'api', { <params> });
// Access L2 resources under `.resources`
api.resources.tables["Todo"].tableArn;
// Access L1 resources under `.resources.cfnResources`
api.resources.cfnResources.cfnGraphqlApi.xrayEnabled = true;
Object.values(api.resources.cfnResources.cfnTables).forEach(table => {
table.pointInTimeRecoverySpecification = { pointInTimeRecoveryEnabled: false };
});
resources.<ResourceType>.<ResourceName>
- you can then perform any CDK action on these resulting resoureces.
import { AmplifyGraphqlApi } from '@aws-amplify/graphql-api-construct'
new AmplifyGraphqlApi(scope: Construct, id: string, props: AmplifyGraphqlApiProps)
Name | Type | Description |
---|---|---|
scope | constructs.Construct | the scope to create this construct within. |
id | string | the id to use for this api. |
props | AmplifyGraphqlApiProps | the properties used to configure the generated api. |
scope
Required the scope to create this construct within.
id
Required the id to use for this api.
props
Required the properties used to configure the generated api.
Name | Description |
---|---|
toString | Returns a string representation of this construct. |
addDynamoDbDataSource | Add a new DynamoDB data source to this API. |
addElasticsearchDataSource | Add a new elasticsearch data source to this API. |
addEventBridgeDataSource | Add an EventBridge data source to this api. |
addFunction | Add an appsync function to the api. |
addHttpDataSource | Add a new http data source to this API. |
addLambdaDataSource | Add a new Lambda data source to this API. |
addNoneDataSource | Add a new dummy data source to this API. |
addOpenSearchDataSource | dd a new OpenSearch data source to this API. |
addRdsDataSource | Add a new Rds data source to this API. |
addResolver | Add a resolver to the api. |
toString
public toString(): string
Returns a string representation of this construct.
addDynamoDbDataSource
public addDynamoDbDataSource(id: string, table: ITable, options?: DataSourceOptions): DynamoDbDataSource
Add a new DynamoDB data source to this API.
This is a proxy method to the L2 GraphqlApi Construct.
id
Required The data source's id.
table
Required The DynamoDB table backing this data source.
options
Optional The optional configuration for this data source.
addElasticsearchDataSource
public addElasticsearchDataSource(id: string, domain: IDomain, options?: DataSourceOptions): ElasticsearchDataSource
Add a new elasticsearch data source to this API.
This is a proxy method to the L2 GraphqlApi Construct.
id
Required The data source's id.
domain
Required The elasticsearch domain for this data source.
options
Optional The optional configuration for this data source.
addEventBridgeDataSource
public addEventBridgeDataSource(id: string, eventBus: IEventBus, options?: DataSourceOptions): EventBridgeDataSource
Add an EventBridge data source to this api.
This is a proxy method to the L2 GraphqlApi Construct.
id
Required The data source's id.
eventBus
Required The EventBridge EventBus on which to put events.
options
Optional The optional configuration for this data source.
addFunction
public addFunction(id: string, props: AddFunctionProps): AppsyncFunction
Add an appsync function to the api.
id
Required the function's id.
props
Required addHttpDataSource
public addHttpDataSource(id: string, endpoint: string, options?: HttpDataSourceOptions): HttpDataSource
Add a new http data source to this API.
This is a proxy method to the L2 GraphqlApi Construct.
id
Required The data source's id.
endpoint
Required The http endpoint.
options
Optional The optional configuration for this data source.
addLambdaDataSource
public addLambdaDataSource(id: string, lambdaFunction: IFunction, options?: DataSourceOptions): LambdaDataSource
Add a new Lambda data source to this API.
This is a proxy method to the L2 GraphqlApi Construct.
id
Required The data source's id.
lambdaFunction
Required The Lambda function to call to interact with this data source.
options
Optional The optional configuration for this data source.
addNoneDataSource
public addNoneDataSource(id: string, options?: DataSourceOptions): NoneDataSource
Add a new dummy data source to this API.
This is a proxy method to the L2 GraphqlApi Construct. Useful for pipeline resolvers and for backend changes that don't require a data source.
id
Required The data source's id.
options
Optional The optional configuration for this data source.
addOpenSearchDataSource
public addOpenSearchDataSource(id: string, domain: IDomain, options?: DataSourceOptions): OpenSearchDataSource
dd a new OpenSearch data source to this API.
This is a proxy method to the L2 GraphqlApi Construct.
id
Required The data source's id.
domain
Required The OpenSearch domain for this data source.
options
Optional The optional configuration for this data source.
addRdsDataSource
public addRdsDataSource(id: string, serverlessCluster: IServerlessCluster, secretStore: ISecret, databaseName?: string, options?: DataSourceOptions): RdsDataSource
Add a new Rds data source to this API.
This is a proxy method to the L2 GraphqlApi Construct.
id
Required The data source's id.
serverlessCluster
Required The serverless cluster to interact with this data source.
secretStore
Required The secret store that contains the username and password for the serverless cluster.
databaseName
Optional The optional name of the database to use within the cluster.
options
Optional The optional configuration for this data source.
addResolver
public addResolver(id: string, props: ExtendedResolverProps): Resolver
Add a resolver to the api.
This is a proxy method to the L2 GraphqlApi Construct.
id
Required The resolver's id.
props
Required the resolver properties.
Name | Description |
---|---|
isConstruct | Checks if x is a construct. |
isConstruct
import { AmplifyGraphqlApi } from '@aws-amplify/graphql-api-construct'
AmplifyGraphqlApi.isConstruct(x: any)
Checks if x
is a construct.
x
Required Any object.
Name | Type | Description |
---|---|---|
node | constructs.Node | The tree node. |
apiId | string | Generated Api Id. |
generatedFunctionSlots | MutationFunctionSlot | QueryFunctionSlot | SubscriptionFunctionSlot[] | Resolvers generated by the transform process, persisted on the side in order to facilitate pulling a manifest for the purposes of inspecting and producing overrides. |
graphqlUrl | string | Graphql URL For the generated API. |
realtimeUrl | string | Realtime URL For the generated API. |
resources | AmplifyGraphqlApiResources | Generated L1 and L2 CDK resources. |
apiKey | string | Generated Api Key if generated. |
node
Required public readonly node: Node;
The tree node.
apiId
Required public readonly apiId: string;
Generated Api Id.
May be a CDK Token.
generatedFunctionSlots
Required public readonly generatedFunctionSlots: MutationFunctionSlot | QueryFunctionSlot | SubscriptionFunctionSlot[];
Resolvers generated by the transform process, persisted on the side in order to facilitate pulling a manifest for the purposes of inspecting and producing overrides.
graphqlUrl
Required public readonly graphqlUrl: string;
Graphql URL For the generated API.
May be a CDK Token.
realtimeUrl
Required public readonly realtimeUrl: string;
Realtime URL For the generated API.
May be a CDK Token.
resources
Required public readonly resources: AmplifyGraphqlApiResources;
Generated L1 and L2 CDK resources.
apiKey
Optional public readonly apiKey: string;
Generated Api Key if generated.
May be a CDK Token.
Input type properties when adding a new appsync.AppsyncFunction to the generated API. This is equivalent to the Omit<appsync.AppsyncFunctionProps, 'api'>.
import { AddFunctionProps } from '@aws-amplify/graphql-api-construct'
const addFunctionProps: AddFunctionProps = { ... }
Name | Type | Description |
---|---|---|
dataSource | aws-cdk-lib.aws_appsync.BaseDataSource | the data source linked to this AppSync Function. |
name | string | the name of the AppSync Function. |
code | aws-cdk-lib.aws_appsync.Code | The function code. |
description | string | the description for this AppSync Function. |
requestMappingTemplate | aws-cdk-lib.aws_appsync.MappingTemplate | the request mapping template for the AppSync Function. |
responseMappingTemplate | aws-cdk-lib.aws_appsync.MappingTemplate | the response mapping template for the AppSync Function. |
runtime | aws-cdk-lib.aws_appsync.FunctionRuntime | The functions runtime. |
dataSource
Required public readonly dataSource: BaseDataSource;
the data source linked to this AppSync Function.
name
Required public readonly name: string;
the name of the AppSync Function.
code
Optional public readonly code: Code;
The function code.
description
Optional public readonly description: string;
the description for this AppSync Function.
requestMappingTemplate
Optional public readonly requestMappingTemplate: MappingTemplate;
the request mapping template for the AppSync Function.
responseMappingTemplate
Optional public readonly responseMappingTemplate: MappingTemplate;
the response mapping template for the AppSync Function.
runtime
Optional public readonly runtime: FunctionRuntime;
The functions runtime.
Use custom resource type 'Custom::AmplifyDynamoDBTable' to provision table.
import { AmplifyDynamoDbModelDataSourceStrategy } from '@aws-amplify/graphql-api-construct'
const amplifyDynamoDbModelDataSourceStrategy: AmplifyDynamoDbModelDataSourceStrategy = { ... }
Name | Type | Description |
---|---|---|
dbType | string | No description. |
provisionStrategy | string | No description. |
dbType
Required public readonly dbType: string;
provisionStrategy
Required public readonly provisionStrategy: string;
L1 CDK resources from the Api which were generated as part of the transform.
These are potentially stored under nested stacks, but presented organized by type instead.
import { AmplifyGraphqlApiCfnResources } from '@aws-amplify/graphql-api-construct'
const amplifyGraphqlApiCfnResources: AmplifyGraphqlApiCfnResources = { ... }
Name | Type | Description |
---|---|---|
additionalCfnResources | {[ key: string ]: aws-cdk-lib.CfnResource} | Remaining L1 resources generated, keyed by logicalId. |
amplifyDynamoDbTables | {[ key: string ]: AmplifyDynamoDbTableWrapper} | The Generated Amplify DynamoDb Table L1 resource wrapper, keyed by model type name. |
cfnDataSources | {[ key: string ]: aws-cdk-lib.aws_appsync.CfnDataSource} | The Generated AppSync DataSource L1 Resources, keyed by logicalId. |
cfnFunctionConfigurations | {[ key: string ]: aws-cdk-lib.aws_appsync.CfnFunctionConfiguration} | The Generated AppSync Function L1 Resources, keyed by logicalId. |
cfnFunctions | {[ key: string ]: aws-cdk-lib.aws_lambda.CfnFunction} | The Generated Lambda Function L1 Resources, keyed by function name. |
cfnGraphqlApi | aws-cdk-lib.aws_appsync.CfnGraphQLApi | The Generated AppSync Api L1 Resource. |
cfnGraphqlSchema | aws-cdk-lib.aws_appsync.CfnGraphQLSchema | The Generated AppSync Schema L1 Resource. |
cfnResolvers | {[ key: string ]: aws-cdk-lib.aws_appsync.CfnResolver} | The Generated AppSync Resolver L1 Resources, keyed by logicalId. |
cfnRoles | {[ key: string ]: aws-cdk-lib.aws_iam.CfnRole} | The Generated IAM Role L1 Resources, keyed by logicalId. |
cfnTables | {[ key: string ]: aws-cdk-lib.aws_dynamodb.CfnTable} | The Generated DynamoDB Table L1 Resources, keyed by logicalId. |
cfnApiKey | aws-cdk-lib.aws_appsync.CfnApiKey | The Generated AppSync Api Key L1 Resource. |
additionalCfnResources
Required public readonly additionalCfnResources: {[ key: string ]: CfnResource};
Remaining L1 resources generated, keyed by logicalId.
amplifyDynamoDbTables
Required public readonly amplifyDynamoDbTables: {[ key: string ]: AmplifyDynamoDbTableWrapper};
The Generated Amplify DynamoDb Table L1 resource wrapper, keyed by model type name.
cfnDataSources
Required public readonly cfnDataSources: {[ key: string ]: CfnDataSource};
The Generated AppSync DataSource L1 Resources, keyed by logicalId.
cfnFunctionConfigurations
Required public readonly cfnFunctionConfigurations: {[ key: string ]: CfnFunctionConfiguration};
The Generated AppSync Function L1 Resources, keyed by logicalId.
cfnFunctions
Required public readonly cfnFunctions: {[ key: string ]: CfnFunction};
The Generated Lambda Function L1 Resources, keyed by function name.
cfnGraphqlApi
Required public readonly cfnGraphqlApi: CfnGraphQLApi;
The Generated AppSync Api L1 Resource.
cfnGraphqlSchema
Required public readonly cfnGraphqlSchema: CfnGraphQLSchema;
The Generated AppSync Schema L1 Resource.
cfnResolvers
Required public readonly cfnResolvers: {[ key: string ]: CfnResolver};
The Generated AppSync Resolver L1 Resources, keyed by logicalId.
cfnRoles
Required public readonly cfnRoles: {[ key: string ]: CfnRole};
The Generated IAM Role L1 Resources, keyed by logicalId.
cfnTables
Required public readonly cfnTables: {[ key: string ]: CfnTable};
The Generated DynamoDB Table L1 Resources, keyed by logicalId.
cfnApiKey
Optional public readonly cfnApiKey: CfnApiKey;
The Generated AppSync Api Key L1 Resource.
Input props for the AmplifyGraphqlApi construct.
Specifies what the input to transform into an Api, and configurations for the transformation process.
import { AmplifyGraphqlApiProps } from '@aws-amplify/graphql-api-construct'
const amplifyGraphqlApiProps: AmplifyGraphqlApiProps = { ... }
Name | Type | Description |
---|---|---|
authorizationModes | AuthorizationModes | Required auth modes for the Api. |
definition | IAmplifyGraphqlDefinition | The definition to transform in a full Api. |
apiName | string | Name to be used for the AppSync Api. |
conflictResolution | ConflictResolution | Configure conflict resolution on the Api, which is required to enable DataStore Api functionality. |
dataStoreConfiguration | DataStoreConfiguration | Configure DataStore conflict resolution on the Api. |
disableOutputStorage | boolean | Disables storing construct output. |
functionNameMap | {[ key: string ]: aws-cdk-lib.aws_lambda.IFunction} | Lambda functions referenced in the definitions's. |
functionSlots | MutationFunctionSlot | QueryFunctionSlot | SubscriptionFunctionSlot[] | Overrides for a given slot in the generated resolver pipelines. |
outputStorageStrategy | IBackendOutputStorageStrategy | Strategy to store construct outputs. |
predictionsBucket | aws-cdk-lib.aws_s3.IBucket | If using predictions, a bucket must be provided which will be used to search for assets. |
stackMappings | {[ key: string ]: string} | StackMappings override the assigned nested stack on a per-resource basis. |
transformerPlugins | any[] | Provide a list of additional custom transformers which are injected into the transform process. |
translationBehavior | PartialTranslationBehavior | This replaces feature flags from the Api construct, for general information on what these parameters do, refer to https://docs.amplify.aws/cli/reference/feature-flags/#graphQLTransformer. |
authorizationModes
Required public readonly authorizationModes: AuthorizationModes;
Required auth modes for the Api.
This object must be a superset of the configured auth providers in the Api definition. For more information, refer to https://docs.amplify.aws/cli/graphql/authorization-rules/
definition
Required public readonly definition: IAmplifyGraphqlDefinition;
The definition to transform in a full Api.
Can be constructed via the AmplifyGraphqlDefinition class.
apiName
Optional public readonly apiName: string;
Name to be used for the AppSync Api.
Default: construct id.
conflictResolution
public readonly conflictResolution: ConflictResolution;
Configure conflict resolution on the Api, which is required to enable DataStore Api functionality.
For more information, refer to https://docs.amplify.aws/lib/datastore/getting-started/q/platform/js/
dataStoreConfiguration
Optional public readonly dataStoreConfiguration: DataStoreConfiguration;
Configure DataStore conflict resolution on the Api.
Conflict resolution is required to enable DataStore Api functionality. For more information, refer to https://docs.amplify.aws/lib/datastore/getting-started/q/platform/js/
disableOutputStorage
Optional public readonly disableOutputStorage: boolean;
Disables storing construct output.
Output storage should be disabled when creating multiple GraphQL APIs in a single CDK synthesis. outputStorageStrategy will be ignored if this is set to true.
functionNameMap
Optional public readonly functionNameMap: {[ key: string ]: IFunction};
Lambda functions referenced in the definitions's.
functionSlots
Optional public readonly functionSlots: MutationFunctionSlot | QueryFunctionSlot | SubscriptionFunctionSlot[];
Overrides for a given slot in the generated resolver pipelines.
For more information about what slots are available, refer to https://docs.amplify.aws/cli/graphql/custom-business-logic/#override-amplify-generated-resolvers.
outputStorageStrategy
Optional public readonly outputStorageStrategy: IBackendOutputStorageStrategy;
Strategy to store construct outputs.
If no outputStorageStrategey is provided a default strategy will be used.
predictionsBucket
Optional public readonly predictionsBucket: IBucket;
If using predictions, a bucket must be provided which will be used to search for assets.
stackMappings
Optional public readonly stackMappings: {[ key: string ]: string};
StackMappings override the assigned nested stack on a per-resource basis.
Only applies to resolvers, and takes the form { : } It is not recommended to use this parameter unless you are encountering stack resource count limits, and worth noting that after initial deployment AppSync resolvers cannot be moved between nested stacks, they will need to be removed from the app, then re-added from a new stack.
transformerPlugins
Optional public readonly transformerPlugins: any[];
Provide a list of additional custom transformers which are injected into the transform process.
These custom transformers must be implemented with aws-cdk-lib >=2.80.0, and
translationBehavior
Optional public readonly translationBehavior: PartialTranslationBehavior;
This replaces feature flags from the Api construct, for general information on what these parameters do, refer to https://docs.amplify.aws/cli/reference/feature-flags/#graphQLTransformer.
Accessible resources from the Api which were generated as part of the transform.
These are potentially stored under nested stacks, but presented organized by type instead.
import { AmplifyGraphqlApiResources } from '@aws-amplify/graphql-api-construct'
const amplifyGraphqlApiResources: AmplifyGraphqlApiResources = { ... }
Name | Type | Description |
---|---|---|
cfnResources | AmplifyGraphqlApiCfnResources | L1 Cfn Resources, for when dipping down a level of abstraction is desirable. |
functions | {[ key: string ]: aws-cdk-lib.aws_lambda.IFunction} | The Generated Lambda Function L1 Resources, keyed by function name. |
graphqlApi | aws-cdk-lib.aws_appsync.IGraphqlApi | The Generated AppSync Api L2 Resource, includes the Schema. |
nestedStacks | {[ key: string ]: aws-cdk-lib.NestedStack} | Nested Stacks generated by the Api Construct. |
roles | {[ key: string ]: aws-cdk-lib.aws_iam.IRole} | The Generated IAM Role L2 Resources, keyed by logicalId. |
tables | {[ key: string ]: aws-cdk-lib.aws_dynamodb.ITable} | The Generated DynamoDB Table L2 Resources, keyed by logicalId. |
cfnResources
Required public readonly cfnResources: AmplifyGraphqlApiCfnResources;
L1 Cfn Resources, for when dipping down a level of abstraction is desirable.
functions
Required public readonly functions: {[ key: string ]: IFunction};
The Generated Lambda Function L1 Resources, keyed by function name.
graphqlApi
Required public readonly graphqlApi: IGraphqlApi;
The Generated AppSync Api L2 Resource, includes the Schema.
nestedStacks
Required public readonly nestedStacks: {[ key: string ]: NestedStack};
Nested Stacks generated by the Api Construct.
roles
Required public readonly roles: {[ key: string ]: IRole};
The Generated IAM Role L2 Resources, keyed by logicalId.
tables
Required public readonly tables: {[ key: string ]: ITable};
The Generated DynamoDB Table L2 Resources, keyed by logicalId.
Configuration for Api Keys on the Graphql Api.
import { ApiKeyAuthorizationConfig } from '@aws-amplify/graphql-api-construct'
const apiKeyAuthorizationConfig: ApiKeyAuthorizationConfig = { ... }
Name | Type | Description |
---|---|---|
expires | aws-cdk-lib.Duration | A duration representing the time from Cloudformation deploy until expiry. |
description | string | Optional description for the Api Key to attach to the Api. |
expires
Required public readonly expires: Duration;
A duration representing the time from Cloudformation deploy until expiry.
description
Optional public readonly description: string;
Optional description for the Api Key to attach to the Api.
Authorization Modes to apply to the Api.
At least one modes must be provided, and if more than one are provided a defaultAuthorizationMode must be specified. For more information on Amplify Api auth, refer to https://docs.amplify.aws/cli/graphql/authorization-rules/#authorization-strategies
import { AuthorizationModes } from '@aws-amplify/graphql-api-construct'
const authorizationModes: AuthorizationModes = { ... }
Name | Type | Description |
---|---|---|
adminRoles | aws-cdk-lib.aws_iam.IRole[] | A list of roles granted full R/W access to the Api. |
apiKeyConfig | ApiKeyAuthorizationConfig | AppSync Api Key config, required if a 'apiKey' auth provider is specified in the Api. |
defaultAuthorizationMode | string | Default auth mode to provide to the Api, required if more than one config type is specified. |
iamConfig | IAMAuthorizationConfig | IAM Auth config, required if an 'iam' auth provider is specified in the Api. |
lambdaConfig | LambdaAuthorizationConfig | Lambda config, required if a 'function' auth provider is specified in the Api. |
oidcConfig | OIDCAuthorizationConfig | Cognito OIDC config, required if a 'oidc' auth provider is specified in the Api. |
userPoolConfig | UserPoolAuthorizationConfig | Cognito UserPool config, required if a 'userPools' auth provider is specified in the Api. |
adminRoles
public readonly adminRoles: IRole[];
A list of roles granted full R/W access to the Api.
apiKeyConfig
Optional public readonly apiKeyConfig: ApiKeyAuthorizationConfig;
AppSync Api Key config, required if a 'apiKey' auth provider is specified in the Api.
Applies to 'public' auth strategy.
defaultAuthorizationMode
Optional public readonly defaultAuthorizationMode: string;
Default auth mode to provide to the Api, required if more than one config type is specified.
iamConfig
Optional public readonly iamConfig: IAMAuthorizationConfig;
IAM Auth config, required if an 'iam' auth provider is specified in the Api.
Applies to 'public' and 'private' auth strategies.
lambdaConfig
Optional public readonly lambdaConfig: LambdaAuthorizationConfig;
Lambda config, required if a 'function' auth provider is specified in the Api.
Applies to 'custom' auth strategy.
oidcConfig
Optional public readonly oidcConfig: OIDCAuthorizationConfig;
Cognito OIDC config, required if a 'oidc' auth provider is specified in the Api.
Applies to 'owner', 'private', and 'group' auth strategies.
userPoolConfig
Optional public readonly userPoolConfig: UserPoolAuthorizationConfig;
Cognito UserPool config, required if a 'userPools' auth provider is specified in the Api.
Applies to 'owner', 'private', and 'group' auth strategies.
Enable optimistic concurrency on the project.
import { AutomergeConflictResolutionStrategy } from '@aws-amplify/graphql-api-construct'
const automergeConflictResolutionStrategy: AutomergeConflictResolutionStrategy = { ... }
Name | Type | Description |
---|---|---|
detectionType | string | The conflict detection type used for resolution. |
handlerType | string | This conflict resolution strategy executes an auto-merge. |
detectionType
Required public readonly detectionType: string;
The conflict detection type used for resolution.
handlerType
Required public readonly handlerType: string;
This conflict resolution strategy executes an auto-merge.
For more information, refer to https://docs.aws.amazon.com/appsync/latest/devguide/conflict-detection-and-sync.html#conflict-detection-and-resolution
Project level configuration for conflict resolution.
import { ConflictResolution } from '@aws-amplify/graphql-api-construct'
const conflictResolution: ConflictResolution = { ... }
Name | Type | Description |
---|---|---|
models | {[ key: string ]: AutomergeConflictResolutionStrategy | OptimisticConflictResolutionStrategy | CustomConflictResolutionStrategy} | Model-specific conflict resolution overrides. |
project | AutomergeConflictResolutionStrategy | OptimisticConflictResolutionStrategy | CustomConflictResolutionStrategy | Project-wide config for conflict resolution. |
models
public readonly models: {[ key: string ]: AutomergeConflictResolutionStrategy | OptimisticConflictResolutionStrategy | CustomConflictResolutionStrategy};
Model-specific conflict resolution overrides.
project
public readonly project: AutomergeConflictResolutionStrategy | OptimisticConflictResolutionStrategy | CustomConflictResolutionStrategy;
Project-wide config for conflict resolution.
Applies to all non-overridden models.
Common parameters for conflict resolution.
import { ConflictResolutionStrategyBase } from '@aws-amplify/graphql-api-construct'
const conflictResolutionStrategyBase: ConflictResolutionStrategyBase = { ... }
Name | Type | Description |
---|---|---|
detectionType | string | The conflict detection type used for resolution. |
detectionType
Required public readonly detectionType: string;
The conflict detection type used for resolution.
Enable custom sync on the project, powered by a lambda.
import { CustomConflictResolutionStrategy } from '@aws-amplify/graphql-api-construct'
const customConflictResolutionStrategy: CustomConflictResolutionStrategy = { ... }
Name | Type | Description |
---|---|---|
detectionType | string | The conflict detection type used for resolution. |
conflictHandler | aws-cdk-lib.aws_lambda.IFunction | The function which will be invoked for conflict resolution. |
handlerType | string | This conflict resolution strategy uses a lambda handler type. |
detectionType
Required public readonly detectionType: string;
The conflict detection type used for resolution.
conflictHandler
Required public readonly conflictHandler: IFunction;
The function which will be invoked for conflict resolution.
handlerType
Required public readonly handlerType: string;
This conflict resolution strategy uses a lambda handler type.
For more information, refer to https://docs.aws.amazon.com/appsync/latest/devguide/conflict-detection-and-sync.html#conflict-detection-and-resolution
The input type for defining a ModelDataSourceStrategy used to resolve a field annotated with a @sql
directive.
Although this is a
public type, you should rarely need to use this. The AmplifyGraphqlDefinition factory methods (e.g., fromString
,
fromFilesAndStrategy
) will automatically construct this structure for you.
import { CustomSqlDataSourceStrategy } from '@aws-amplify/graphql-api-construct'
const customSqlDataSourceStrategy: CustomSqlDataSourceStrategy = { ... }
Name | Type | Description |
---|---|---|
fieldName | string | The field name with which the custom SQL is associated. |
strategy | SQLLambdaModelDataSourceStrategy | The strategy used to create the datasource that will resolve the custom SQL statement. |
typeName | string | The built-in type (either "Query" or "Mutation") with which the custom SQL is associated. |
fieldName
Required public readonly fieldName: string;
The field name with which the custom SQL is associated.
strategy
Required public readonly strategy: SQLLambdaModelDataSourceStrategy;
The strategy used to create the datasource that will resolve the custom SQL statement.
typeName
Required public readonly typeName: string;
The built-in type (either "Query" or "Mutation") with which the custom SQL is associated.
Project level configuration for DataStore.
import { DataStoreConfiguration } from '@aws-amplify/graphql-api-construct'
const dataStoreConfiguration: DataStoreConfiguration = { ... }
Name | Type | Description |
---|---|---|
models | {[ key: string ]: AutomergeConflictResolutionStrategy | OptimisticConflictResolutionStrategy | CustomConflictResolutionStrategy} | Model-specific conflict resolution overrides. |
project | AutomergeConflictResolutionStrategy | OptimisticConflictResolutionStrategy | CustomConflictResolutionStrategy | Project-wide config for conflict resolution. |
models
Optional public readonly models: {[ key: string ]: AutomergeConflictResolutionStrategy | OptimisticConflictResolutionStrategy | CustomConflictResolutionStrategy};
Model-specific conflict resolution overrides.
project
Optional public readonly project: AutomergeConflictResolutionStrategy | OptimisticConflictResolutionStrategy | CustomConflictResolutionStrategy;
Project-wide config for conflict resolution.
Applies to all non-overridden models.
Use default CloudFormation type 'AWS::DynamoDB::Table' to provision table.
import { DefaultDynamoDbModelDataSourceStrategy } from '@aws-amplify/graphql-api-construct'
const defaultDynamoDbModelDataSourceStrategy: DefaultDynamoDbModelDataSourceStrategy = { ... }
Name | Type | Description |
---|---|---|
dbType | string | No description. |
provisionStrategy | string | No description. |
dbType
Required public readonly dbType: string;
provisionStrategy
Required public readonly provisionStrategy: string;
Common slot parameters.
import { FunctionSlotBase } from '@aws-amplify/graphql-api-construct'
const functionSlotBase: FunctionSlotBase = { ... }
Name | Type | Description |
---|---|---|
fieldName | string | The field to attach this function to on the Api definition. |
function | FunctionSlotOverride | The overridden behavior for this slot. |
slotIndex | number | The slot index to use to inject this into the execution pipeline. |
fieldName
Required public readonly fieldName: string;
The field to attach this function to on the Api definition.
function
Required public readonly function: FunctionSlotOverride;
The overridden behavior for this slot.
slotIndex
Required public readonly slotIndex: number;
The slot index to use to inject this into the execution pipeline.
For more information on slotting, refer to https://docs.amplify.aws/cli/graphql/custom-business-logic/#extend-amplify-generated-resolvers
Params exposed to support configuring and overriding pipelined slots.
This allows configuration of the underlying function, including the request and response mapping templates.
import { FunctionSlotOverride } from '@aws-amplify/graphql-api-construct'
const functionSlotOverride: FunctionSlotOverride = { ... }
Name | Type | Description |
---|---|---|
requestMappingTemplate | aws-cdk-lib.aws_appsync.MappingTemplate | Override request mapping template for the function slot. |
responseMappingTemplate | aws-cdk-lib.aws_appsync.MappingTemplate | Override response mapping template for the function slot. |
requestMappingTemplate
Optional public readonly requestMappingTemplate: MappingTemplate;
Override request mapping template for the function slot.
Executed before the datasource is invoked.
responseMappingTemplate
Optional public readonly responseMappingTemplate: MappingTemplate;
Override response mapping template for the function slot.
Executed after the datasource is invoked.
Configuration for IAM Authorization on the Graphql Api.
import { IAMAuthorizationConfig } from '@aws-amplify/graphql-api-construct'
const iAMAuthorizationConfig: IAMAuthorizationConfig = { ... }
Name | Type | Description |
---|---|---|
authenticatedUserRole | aws-cdk-lib.aws_iam.IRole | Authenticated user role, applies to { provider: iam, allow: private } access. |
identityPoolId | string | ID for the Cognito Identity Pool vending auth and unauth roles. |
unauthenticatedUserRole | aws-cdk-lib.aws_iam.IRole | Unauthenticated user role, applies to { provider: iam, allow: public } access. |
allowListedRoles | string | aws-cdk-lib.aws_iam.IRole[] | A list of IAM roles which will be granted full read/write access to the generated model if IAM auth is enabled. |
authenticatedUserRole
Required public readonly authenticatedUserRole: IRole;
Authenticated user role, applies to { provider: iam, allow: private } access.
identityPoolId
Required public readonly identityPoolId: string;
ID for the Cognito Identity Pool vending auth and unauth roles.
Format: <region>:<id string>
unauthenticatedUserRole
Required public readonly unauthenticatedUserRole: IRole;
Unauthenticated user role, applies to { provider: iam, allow: public } access.
allowListedRoles
Optional public readonly allowListedRoles: string | IRole[];
A list of IAM roles which will be granted full read/write access to the generated model if IAM auth is enabled.
If an IRole is provided, the role name
will be used for matching.
If a string is provided, the raw value will be used for matching.
Configuration for Custom Lambda authorization on the Graphql Api.
import { LambdaAuthorizationConfig } from '@aws-amplify/graphql-api-construct'
const lambdaAuthorizationConfig: LambdaAuthorizationConfig = { ... }
Name | Type | Description |
---|---|---|
function | aws-cdk-lib.aws_lambda.IFunction | The authorizer lambda function. |
ttl | aws-cdk-lib.Duration | How long the results are cached. |
function
Required public readonly function: IFunction;
The authorizer lambda function.
ttl
Required public readonly ttl: Duration;
How long the results are cached.
Slot types for Mutation Resolvers.
import { MutationFunctionSlot } from '@aws-amplify/graphql-api-construct'
const mutationFunctionSlot: MutationFunctionSlot = { ... }
Name | Type | Description |
---|---|---|
fieldName | string | The field to attach this function to on the Api definition. |
function | FunctionSlotOverride | The overridden behavior for this slot. |
slotIndex | number | The slot index to use to inject this into the execution pipeline. |
slotName | string | The slot name to inject this behavior into. |
typeName | string | This slot type applies to the Mutation type on the Api definition. |
fieldName
Required public readonly fieldName: string;
The field to attach this function to on the Api definition.
function
Required public readonly function: FunctionSlotOverride;
The overridden behavior for this slot.
slotIndex
Required public readonly slotIndex: number;
The slot index to use to inject this into the execution pipeline.
For more information on slotting, refer to https://docs.amplify.aws/cli/graphql/custom-business-logic/#extend-amplify-generated-resolvers
slotName
Required public readonly slotName: string;
The slot name to inject this behavior into.
For more information on slotting, refer to https://docs.amplify.aws/cli/graphql/custom-business-logic/#extend-amplify-generated-resolvers
typeName
Required public readonly typeName: string;
This slot type applies to the Mutation type on the Api definition.
Configuration for OpenId Connect Authorization on the Graphql Api.
import { OIDCAuthorizationConfig } from '@aws-amplify/graphql-api-construct'
const oIDCAuthorizationConfig: OIDCAuthorizationConfig = { ... }
Name | Type | Description |
---|---|---|
oidcIssuerUrl | string | Url for the OIDC token issuer. |
oidcProviderName | string | The issuer for the OIDC configuration. |
tokenExpiryFromAuth | aws-cdk-lib.Duration | The duration an OIDC token is valid after being authenticated by OIDC provider. |
tokenExpiryFromIssue | aws-cdk-lib.Duration | The duration an OIDC token is valid after being issued to a user. |
clientId | string | The client identifier of the Relying party at the OpenID identity provider. |
oidcIssuerUrl
Required public readonly oidcIssuerUrl: string;
Url for the OIDC token issuer.
oidcProviderName
Required public readonly oidcProviderName: string;
The issuer for the OIDC configuration.
tokenExpiryFromAuth
Required public readonly tokenExpiryFromAuth: Duration;
The duration an OIDC token is valid after being authenticated by OIDC provider.
auth_time claim in OIDC token is required for this validation to work.
tokenExpiryFromIssue
Required public readonly tokenExpiryFromIssue: Duration;
The duration an OIDC token is valid after being issued to a user.
This validation uses iat claim of OIDC token.
clientId
Optional public readonly clientId: string;
The client identifier of the Relying party at the OpenID identity provider.
A regular expression can be specified so AppSync can validate against multiple client identifiers at a time. Example
Enable automerge on the project.
import { OptimisticConflictResolutionStrategy } from '@aws-amplify/graphql-api-construct'
const optimisticConflictResolutionStrategy: OptimisticConflictResolutionStrategy = { ... }
Name | Type | Description |
---|---|---|
detectionType | string | The conflict detection type used for resolution. |
handlerType | string | This conflict resolution strategy the _version to perform optimistic concurrency. |
detectionType
Required public readonly detectionType: string;
The conflict detection type used for resolution.
handlerType
Required public readonly handlerType: string;
This conflict resolution strategy the _version to perform optimistic concurrency.
For more information, refer to https://docs.aws.amazon.com/appsync/latest/devguide/conflict-detection-and-sync.html#conflict-detection-and-resolution
A utility interface equivalent to Partial.
import { PartialTranslationBehavior } from '@aws-amplify/graphql-api-construct'
const partialTranslationBehavior: PartialTranslationBehavior = { ... }
Name | Type | Description |
---|---|---|
allowDestructiveGraphqlSchemaUpdates | boolean | The following schema updates require replacement of the underlying DynamoDB table:. |
disableResolverDeduping | boolean | Disable resolver deduping, this can sometimes cause problems because dedupe ordering isn't stable today, which can lead to circular dependencies across stacks if models are reordered. |
enableAutoIndexQueryNames | boolean | Automate generation of query names, and as a result attaching all indexes as queries to the generated Api. |
enableSearchNodeToNodeEncryption | boolean | If enabled, set nodeToNodeEncryption on the searchable domain (if one exists). |
enableTransformerCfnOutputs | boolean | When enabled, internal cfn outputs which existed in Amplify-generated apps will continue to be emitted. |
populateOwnerFieldForStaticGroupAuth | boolean | Ensure that the owner field is still populated even if a static iam or group authorization applies. |
replaceTableUponGsiUpdate | boolean | This behavior will only come into effect when both "allowDestructiveGraphqlSchemaUpdates" and this value are set to true. |
respectPrimaryKeyAttributesOnConnectionField | boolean | Enable custom primary key support, there's no good reason to disable this unless trying not to update a legacy app. |
sandboxModeEnabled | boolean | Enabling sandbox mode will enable api key auth on all models in the transformed schema. |
secondaryKeyAsGSI | boolean | If disabled, generated. |
shouldDeepMergeDirectiveConfigDefaults | boolean | Restore parity w/ GQLv1. |
suppressApiKeyGeneration | boolean | If enabled, disable api key resource generation even if specified as an auth rule on the construct. |
useSubUsernameForDefaultIdentityClaim | boolean | Ensure that oidc and userPool auth use the sub field in the for the username field, which disallows new users with the same id to access data from a deleted user in the pool. |
allowDestructiveGraphqlSchemaUpdates
Optional public readonly allowDestructiveGraphqlSchemaUpdates: boolean;
The following schema updates require replacement of the underlying DynamoDB table:.
Removing or renaming a model
ALL DATA WILL BE LOST when the table replacement happens. When enabled, destructive updates are allowed. This will only affect DynamoDB tables with provision strategy "AMPLIFY_TABLE".
disableResolverDeduping
Optional public readonly disableResolverDeduping: boolean;
Disable resolver deduping, this can sometimes cause problems because dedupe ordering isn't stable today, which can lead to circular dependencies across stacks if models are reordered.
enableAutoIndexQueryNames
Optional public readonly enableAutoIndexQueryNames: boolean;
Automate generation of query names, and as a result attaching all indexes as queries to the generated Api.
If enabled,
enableSearchNodeToNodeEncryption
Optional public readonly enableSearchNodeToNodeEncryption: boolean;
If enabled, set nodeToNodeEncryption on the searchable domain (if one exists).
Not recommended for use, prefer to use `Object.values(resources.additionalResources['AWS::Elasticsearch::Domain']).forEach((domain: CfnDomain) => { domain.NodeToNodeEncryptionOptions = { Enabled: True }; });
enableTransformerCfnOutputs
Optional public readonly enableTransformerCfnOutputs: boolean;
When enabled, internal cfn outputs which existed in Amplify-generated apps will continue to be emitted.
populateOwnerFieldForStaticGroupAuth
Optional public readonly populateOwnerFieldForStaticGroupAuth: boolean;
Ensure that the owner field is still populated even if a static iam or group authorization applies.
replaceTableUponGsiUpdate
Optional public readonly replaceTableUponGsiUpdate: boolean;
This behavior will only come into effect when both "allowDestructiveGraphqlSchemaUpdates" and this value are set to true.
When enabled, any global secondary index update operation will replace the table instead of iterative deployment, which will WIPE ALL EXISTING DATA but cost much less time for deployment This will only affect DynamoDB tables with provision strategy "AMPLIFY_TABLE".
respectPrimaryKeyAttributesOnConnectionField
Optional public readonly respectPrimaryKeyAttributesOnConnectionField: boolean;
Enable custom primary key support, there's no good reason to disable this unless trying not to update a legacy app.
sandboxModeEnabled
Optional public readonly sandboxModeEnabled: boolean;
Enabling sandbox mode will enable api key auth on all models in the transformed schema.
secondaryKeyAsGSI
Optional public readonly secondaryKeyAsGSI: boolean;
If disabled, generated.
shouldDeepMergeDirectiveConfigDefaults
Optional public readonly shouldDeepMergeDirectiveConfigDefaults: boolean;
Restore parity w/ GQLv1.
suppressApiKeyGeneration
Optional public readonly suppressApiKeyGeneration: boolean;
If enabled, disable api key resource generation even if specified as an auth rule on the construct.
This is a legacy parameter from the Graphql Transformer existing in Amplify CLI, not recommended to change.
useSubUsernameForDefaultIdentityClaim
Optional public readonly useSubUsernameForDefaultIdentityClaim: boolean;
Ensure that oidc and userPool auth use the sub
field in the for the username field, which disallows new users with the same id to access data from a deleted user in the pool.
The configuration for the provisioned concurrency of the Lambda.
import { ProvisionedConcurrencyConfig } from '@aws-amplify/graphql-api-construct'
const provisionedConcurrencyConfig: ProvisionedConcurrencyConfig = { ... }
Name | Type | Description |
---|---|---|
provisionedConcurrentExecutions | number | The amount of provisioned concurrency to allocate. |
provisionedConcurrentExecutions
Required public readonly provisionedConcurrentExecutions: number;
The amount of provisioned concurrency to allocate.
Wrapper for provisioned throughput config in DDB.
import { ProvisionedThroughput } from '@aws-amplify/graphql-api-construct'
const provisionedThroughput: ProvisionedThroughput = { ... }
Name | Type | Description |
---|---|---|
readCapacityUnits | number | The read capacity units on the table or index. |
writeCapacityUnits | number | The write capacity units on the table or index. |
readCapacityUnits
Required public readonly readCapacityUnits: number;
The read capacity units on the table or index.
writeCapacityUnits
Required public readonly writeCapacityUnits: number;
The write capacity units on the table or index.
Slot types for Query Resolvers.
import { QueryFunctionSlot } from '@aws-amplify/graphql-api-construct'
const queryFunctionSlot: QueryFunctionSlot = { ... }
Name | Type | Description |
---|---|---|
fieldName | string | The field to attach this function to on the Api definition. |
function | FunctionSlotOverride | The overridden behavior for this slot. |
slotIndex | number | The slot index to use to inject this into the execution pipeline. |
slotName | string | The slot name to inject this behavior into. |
typeName | string | This slot type applies to the Query type on the Api definition. |
fieldName
Required public readonly fieldName: string;
The field to attach this function to on the Api definition.
function
Required public readonly function: FunctionSlotOverride;
The overridden behavior for this slot.
slotIndex
Required public readonly slotIndex: number;
The slot index to use to inject this into the execution pipeline.
For more information on slotting, refer to https://docs.amplify.aws/cli/graphql/custom-business-logic/#extend-amplify-generated-resolvers
slotName
Required public readonly slotName: string;
The slot name to inject this behavior into.
For more information on slotting, refer to https://docs.amplify.aws/cli/graphql/custom-business-logic/#extend-amplify-generated-resolvers
typeName
Required public readonly typeName: string;
This slot type applies to the Query type on the Api definition.
A strategy that creates a Lambda to connect to a pre-existing SQL table to resolve model data.
import { SQLLambdaModelDataSourceStrategy } from '@aws-amplify/graphql-api-construct'
const sQLLambdaModelDataSourceStrategy: SQLLambdaModelDataSourceStrategy = { ... }
Name | Type | Description |
---|---|---|
dbConnectionConfig | SqlModelDataSourceSecretsManagerDbConnectionConfig | SqlModelDataSourceSsmDbConnectionConfig | The parameters the Lambda data source will use to connect to the database. |
dbType | string | The type of the SQL database used to process model operations for this definition. |
name | string | The name of the strategy. |
customSqlStatements | {[ key: string ]: string} | Custom SQL statements. |
sqlLambdaProvisionedConcurrencyConfig | ProvisionedConcurrencyConfig | The configuration for the provisioned concurrency of the Lambda. |
vpcConfiguration | VpcConfig | The configuration of the VPC into which to install the Lambda. |
dbConnectionConfig
Required public readonly dbConnectionConfig: SqlModelDataSourceSecretsManagerDbConnectionConfig | SqlModelDataSourceSsmDbConnectionConfig;
The parameters the Lambda data source will use to connect to the database.
dbType
Required public readonly dbType: string;
The type of the SQL database used to process model operations for this definition.
name
Required public readonly name: string;
The name of the strategy.
This will be used to name the AppSync DataSource itself, plus any associated resources like resolver Lambdas. This name must be unique across all schema definitions in a GraphQL API.
customSqlStatements
Optional public readonly customSqlStatements: {[ key: string ]: string};
Custom SQL statements.
The key is the value of the references
attribute of the @sql
directive in the schema
; the value is the SQL
to be executed.
sqlLambdaProvisionedConcurrencyConfig
Optional public readonly sqlLambdaProvisionedConcurrencyConfig: ProvisionedConcurrencyConfig;
The configuration for the provisioned concurrency of the Lambda.
vpcConfiguration
Optional public readonly vpcConfiguration: VpcConfig;
The configuration of the VPC into which to install the Lambda.
The credentials stored in Secrets Manager that the lambda data source will use to connect to the database.
The managed secret should be in the same region as the lambda.
import { SqlModelDataSourceSecretsManagerDbConnectionConfig } from '@aws-amplify/graphql-api-construct'
const sqlModelDataSourceSecretsManagerDbConnectionConfig: SqlModelDataSourceSecretsManagerDbConnectionConfig = { ... }
Name | Type | Description |
---|---|---|
databaseName | string | The database name. |
hostname | string | The hostame of the database. |
port | number | The port number of the database proxy, cluster, or instance. |
secretArn | string | The ARN of the managed secret with username, password, and hostname to use when connecting to the database. |
keyArn | string | The ARN of the customer managed encryption key for the secret. |
databaseName
Required public readonly databaseName: string;
The database name.
hostname
Required public readonly hostname: string;
The hostame of the database.
port
Required public readonly port: number;
The port number of the database proxy, cluster, or instance.
secretArn
Required public readonly secretArn: string;
The ARN of the managed secret with username, password, and hostname to use when connecting to the database.
keyArn
Optional public readonly keyArn: string;
The ARN of the customer managed encryption key for the secret.
If not supplied, the secret is expected to be encrypted with the default AWS-managed key. *
The Secure Systems Manager parameter paths the Lambda data source will use to connect to the database.
These parameters are retrieved from Secure Systems Manager in the same region as the Lambda.
import { SqlModelDataSourceSsmDbConnectionConfig } from '@aws-amplify/graphql-api-construct'
const sqlModelDataSourceSsmDbConnectionConfig: SqlModelDataSourceSsmDbConnectionConfig = { ... }
Name | Type | Description |
---|---|---|
databaseNameSsmPath | string | The Secure Systems Manager parameter containing the database name. |
hostnameSsmPath | string | The Secure Systems Manager parameter containing the hostname of the database. |
passwordSsmPath | string | The Secure Systems Manager parameter containing the password to use when connecting to the database. |
portSsmPath | string | The Secure Systems Manager parameter containing the port number of the database proxy, cluster, or instance. |
usernameSsmPath | string | The Secure Systems Manager parameter containing the username to use when connecting to the database. |
databaseNameSsmPath
Required public readonly databaseNameSsmPath: string;
The Secure Systems Manager parameter containing the database name.
hostnameSsmPath
Required public readonly hostnameSsmPath: string;
The Secure Systems Manager parameter containing the hostname of the database.
For RDS-based SQL data sources, this can be the hostname of a database proxy, cluster, or instance.
passwordSsmPath
Required public readonly passwordSsmPath: string;
The Secure Systems Manager parameter containing the password to use when connecting to the database.
portSsmPath
Required public readonly portSsmPath: string;
The Secure Systems Manager parameter containing the port number of the database proxy, cluster, or instance.
usernameSsmPath
Required public readonly usernameSsmPath: string;
The Secure Systems Manager parameter containing the username to use when connecting to the database.
Represents the settings used to enable server-side encryption.
import { SSESpecification } from '@aws-amplify/graphql-api-construct'
const sSESpecification: SSESpecification = { ... }
Name | Type | Description |
---|---|---|
sseEnabled | boolean | Indicates whether server-side encryption is done using an AWS managed key or an AWS owned key. |
kmsMasterKeyId | string | The AWS KMS key that should be used for the AWS KMS encryption. |
sseType | SSEType | Server-side encryption type. |
sseEnabled
Required public readonly sseEnabled: boolean;
Indicates whether server-side encryption is done using an AWS managed key or an AWS owned key.
If enabled (true), server-side encryption type is set to KMS
and an AWS managed key is used ( AWS KMS charges apply).
If disabled (false) or not specified, server-side encryption is set to AWS owned key.
kmsMasterKeyId
Optional public readonly kmsMasterKeyId: string;
The AWS KMS key that should be used for the AWS KMS encryption.
To specify a key, use its key ID, Amazon Resource Name (ARN), alias name, or alias ARN. Note that you should only provide
this parameter if the key is different from the default DynamoDB key alias/aws/dynamodb
.
sseType
Optional public readonly sseType: SSEType;
Server-side encryption type.
The only supported value is:
KMS
Server-side encryption that uses AWS Key Management Service.
The key is stored in your account and is managed by AWS KMS ( AWS KMS charges apply).
Represents the DynamoDB Streams configuration for a table in DynamoDB.
import { StreamSpecification } from '@aws-amplify/graphql-api-construct'
const streamSpecification: StreamSpecification = { ... }
Name | Type | Description |
---|---|---|
streamViewType | aws-cdk-lib.aws_dynamodb.StreamViewType | When an item in the table is modified, StreamViewType determines what information is written to the stream for this table. |
streamViewType
Required public readonly streamViewType: StreamViewType;
When an item in the table is modified, StreamViewType
determines what information is written to the stream for this table.
Valid values for StreamViewType
are:
KEYS_ONLY
- Only the key attributes of the modified item are written to the stream.NEW_IMAGE
- The entire item, as it appears after it was modified, is written to the stream.OLD_IMAGE
- The entire item, as it appeared before it was modified, is written to the stream.NEW_AND_OLD_IMAGES
- Both the new and the old item images of the item are written to the stream.Subnet configuration for VPC endpoints used by a Lambda resolver for a SQL-based data source.
Although it is possible to create multiple subnets in a single availability zone, VPC service endpoints may only be deployed to a single subnet in a given availability zone. This structure ensures that the Lambda function and VPC service endpoints are mutually consistent.
import { SubnetAvailabilityZone } from '@aws-amplify/graphql-api-construct'
const subnetAvailabilityZone: SubnetAvailabilityZone = { ... }
Name | Type | Description |
---|---|---|
availabilityZone | string | The availability zone of the subnet. |
subnetId | string | The subnet ID to install the Lambda data source in. |
availabilityZone
Required public readonly availabilityZone: string;
The availability zone of the subnet.
subnetId
Required public readonly subnetId: string;
The subnet ID to install the Lambda data source in.
Slot types for Subscription Resolvers.
import { SubscriptionFunctionSlot } from '@aws-amplify/graphql-api-construct'
const subscriptionFunctionSlot: SubscriptionFunctionSlot = { ... }
Name | Type | Description |
---|---|---|
fieldName | string | The field to attach this function to on the Api definition. |
function | FunctionSlotOverride | The overridden behavior for this slot. |
slotIndex | number | The slot index to use to inject this into the execution pipeline. |
slotName | string | The slot name to inject this behavior into. |
typeName | string | This slot type applies to the Subscription type on the Api definition. |
fieldName
Required public readonly fieldName: string;
The field to attach this function to on the Api definition.
function
Required public readonly function: FunctionSlotOverride;
The overridden behavior for this slot.
slotIndex
Required public readonly slotIndex: number;
The slot index to use to inject this into the execution pipeline.
For more information on slotting, refer to https://docs.amplify.aws/cli/graphql/custom-business-logic/#extend-amplify-generated-resolvers
slotName
Required public readonly slotName: string;
The slot name to inject this behavior into.
For more information on slotting, refer to https://docs.amplify.aws/cli/graphql/custom-business-logic/#extend-amplify-generated-resolvers
typeName
Required public readonly typeName: string;
This slot type applies to the Subscription type on the Api definition.
Shape for TTL config.
import { TimeToLiveSpecification } from '@aws-amplify/graphql-api-construct'
const timeToLiveSpecification: TimeToLiveSpecification = { ... }
Name | Type | Description |
---|---|---|
enabled | boolean | Boolean determining if the ttl is enabled or not. |
attributeName | string | Attribute name to apply to the ttl spec. |
enabled
Required public readonly enabled: boolean;
Boolean determining if the ttl is enabled or not.
attributeName
Optional public readonly attributeName: string;
Attribute name to apply to the ttl spec.
Strongly typed set of shared parameters for all transformers, and core layer.
This is intended to replace feature flags, to ensure param coercion happens in a single location, and isn't spread around the transformers, where they can have different default behaviors.
import { TranslationBehavior } from '@aws-amplify/graphql-api-construct'
const translationBehavior: TranslationBehavior = { ... }
Name | Type | Description |
---|---|---|
allowDestructiveGraphqlSchemaUpdates | boolean | The following schema updates require replacement of the underlying DynamoDB table:. |
disableResolverDeduping | boolean | Disable resolver deduping, this can sometimes cause problems because dedupe ordering isn't stable today, which can lead to circular dependencies across stacks if models are reordered. |
enableAutoIndexQueryNames | boolean | Automate generation of query names, and as a result attaching all indexes as queries to the generated Api. |
enableSearchNodeToNodeEncryption | boolean | No description. |
enableTransformerCfnOutputs | boolean | When enabled, internal cfn outputs which existed in Amplify-generated apps will continue to be emitted. |
populateOwnerFieldForStaticGroupAuth | boolean | Ensure that the owner field is still populated even if a static iam or group authorization applies. |
replaceTableUponGsiUpdate | boolean | This behavior will only come into effect when both "allowDestructiveGraphqlSchemaUpdates" and this value are set to true. |
respectPrimaryKeyAttributesOnConnectionField | boolean | Enable custom primary key support, there's no good reason to disable this unless trying not to update a legacy app. |
sandboxModeEnabled | boolean | Enabling sandbox mode will enable api key auth on all models in the transformed schema. |
secondaryKeyAsGSI | boolean | If disabled, generated. |
shouldDeepMergeDirectiveConfigDefaults | boolean | Restore parity w/ GQLv1. |
suppressApiKeyGeneration | boolean | If enabled, disable api key resource generation even if specified as an auth rule on the construct. |
useSubUsernameForDefaultIdentityClaim | boolean | Ensure that oidc and userPool auth use the sub field in the for the username field, which disallows new users with the same id to access data from a deleted user in the pool. |
allowDestructiveGraphqlSchemaUpdates
Required public readonly allowDestructiveGraphqlSchemaUpdates: boolean;
The following schema updates require replacement of the underlying DynamoDB table:.
Removing or renaming a model
ALL DATA WILL BE LOST when the table replacement happens. When enabled, destructive updates are allowed. This will only affect DynamoDB tables with provision strategy "AMPLIFY_TABLE".
disableResolverDeduping
Required public readonly disableResolverDeduping: boolean;
Disable resolver deduping, this can sometimes cause problems because dedupe ordering isn't stable today, which can lead to circular dependencies across stacks if models are reordered.
enableAutoIndexQueryNames
Required public readonly enableAutoIndexQueryNames: boolean;
Automate generation of query names, and as a result attaching all indexes as queries to the generated Api.
If enabled,
enableSearchNodeToNodeEncryption
Required public readonly enableSearchNodeToNodeEncryption: boolean;
enableTransformerCfnOutputs
Required public readonly enableTransformerCfnOutputs: boolean;
When enabled, internal cfn outputs which existed in Amplify-generated apps will continue to be emitted.
populateOwnerFieldForStaticGroupAuth
Required public readonly populateOwnerFieldForStaticGroupAuth: boolean;
Ensure that the owner field is still populated even if a static iam or group authorization applies.
replaceTableUponGsiUpdate
Required public readonly replaceTableUponGsiUpdate: boolean;
This behavior will only come into effect when both "allowDestructiveGraphqlSchemaUpdates" and this value are set to true.
When enabled, any GSI update operation will replace the table instead of iterative deployment, which will WIPE ALL EXISTING DATA but cost much less time for deployment This will only affect DynamoDB tables with provision strategy "AMPLIFY_TABLE".
respectPrimaryKeyAttributesOnConnectionField
Required public readonly respectPrimaryKeyAttributesOnConnectionField: boolean;
Enable custom primary key support, there's no good reason to disable this unless trying not to update a legacy app.
sandboxModeEnabled
Required public readonly sandboxModeEnabled: boolean;
Enabling sandbox mode will enable api key auth on all models in the transformed schema.
secondaryKeyAsGSI
Required public readonly secondaryKeyAsGSI: boolean;
If disabled, generated.
shouldDeepMergeDirectiveConfigDefaults
Required public readonly shouldDeepMergeDirectiveConfigDefaults: boolean;
Restore parity w/ GQLv1.
suppressApiKeyGeneration
Required public readonly suppressApiKeyGeneration: boolean;
If enabled, disable api key resource generation even if specified as an auth rule on the construct.
This is a legacy parameter from the Graphql Transformer existing in Amplify CLI, not recommended to change.
useSubUsernameForDefaultIdentityClaim
Required public readonly useSubUsernameForDefaultIdentityClaim: boolean;
Ensure that oidc and userPool auth use the sub
field in the for the username field, which disallows new users with the same id to access data from a deleted user in the pool.
Configuration for Cognito UserPool Authorization on the Graphql Api.
import { UserPoolAuthorizationConfig } from '@aws-amplify/graphql-api-construct'
const userPoolAuthorizationConfig: UserPoolAuthorizationConfig = { ... }
Name | Type | Description |
---|---|---|
userPool | aws-cdk-lib.aws_cognito.IUserPool | The Cognito User Pool which is used to authenticated JWT tokens, and vends group and user information. |
userPool
Required public readonly userPool: IUserPool;
The Cognito User Pool which is used to authenticated JWT tokens, and vends group and user information.
Configuration of the VPC in which to install a Lambda to resolve queries against a SQL-based data source.
The SQL Lambda will be deployed into the specified VPC, subnets, and security groups. The specified subnets and security groups must be in the same VPC. The VPC must have at least one subnet. The construct will also create VPC service endpoints in the specified subnets, as well as inbound security rules, to allow traffic on port 443 within each security group. This allows the Lambda to read database connection information from Secure Systems Manager.
import { VpcConfig } from '@aws-amplify/graphql-api-construct'
const vpcConfig: VpcConfig = { ... }
Name | Type | Description |
---|---|---|
securityGroupIds | string[] | The security groups to install the Lambda data source in. |
subnetAvailabilityZoneConfig | SubnetAvailabilityZone[] | The subnets to install the Lambda data source in, one per availability zone. |
vpcId | string | The VPC to install the Lambda data source in. |
securityGroupIds
Required public readonly securityGroupIds: string[];
The security groups to install the Lambda data source in.
subnetAvailabilityZoneConfig
Required public readonly subnetAvailabilityZoneConfig: SubnetAvailabilityZone[];
The subnets to install the Lambda data source in, one per availability zone.
vpcId
Required public readonly vpcId: string;
The VPC to install the Lambda data source in.
Wrapper class around Custom::AmplifyDynamoDBTable custom resource, to simplify the override experience a bit.
This is NOT a construct, just an easier way to access
the generated construct.
This is a wrapper intended to mimic the aws_cdk_lib.aws_dynamodb.Table
functionality more-or-less.
Notable differences is the addition of TKTK properties, to account for the fact that they're constructor props
in the CDK construct, as well as the removal of all from*, grant*, and metric* methods implemented by Table.
import { AmplifyDynamoDbTableWrapper } from '@aws-amplify/graphql-api-construct'
new AmplifyDynamoDbTableWrapper(resource: CfnResource)
Name | Type | Description |
---|---|---|
resource | aws-cdk-lib.CfnResource | the Cfn resource. |
resource
Required the Cfn resource.
Name | Description |
---|---|
applyRemovalPolicy | Set the deletion policy of the resource based on the removal policy specified. |
setGlobalSecondaryIndexProvisionedThroughput | Set the provisionedThroughtput for a specified GSI by name. |
applyRemovalPolicy
public applyRemovalPolicy(policy: RemovalPolicy): void
Set the deletion policy of the resource based on the removal policy specified.
policy
Required removal policy to set.
setGlobalSecondaryIndexProvisionedThroughput
public setGlobalSecondaryIndexProvisionedThroughput(indexName: string, provisionedThroughput: ProvisionedThroughput): void
Set the provisionedThroughtput for a specified GSI by name.
indexName
Required the index to specify a provisionedThroughput config for.
provisionedThroughput
Required the config to set.
Name | Description |
---|---|
isAmplifyDynamoDbTableResource | Return true and perform type narrowing if a given input appears to be capable of. |
isAmplifyDynamoDbTableResource
import { AmplifyDynamoDbTableWrapper } from '@aws-amplify/graphql-api-construct'
AmplifyDynamoDbTableWrapper.isAmplifyDynamoDbTableResource(x: any)
Return true and perform type narrowing if a given input appears to be capable of.
x
Required the object to check.
Name | Type | Description |
---|---|---|
billingMode | aws-cdk-lib.aws_dynamodb.BillingMode | Specify how you are charged for read and write throughput and how you manage capacity. |
deletionProtectionEnabled | boolean | Set table deletion protection. |
pointInTimeRecoveryEnabled | boolean | Whether point-in-time recovery is enabled. |
provisionedThroughput | ProvisionedThroughput | Update the provisioned throughput for the base table. |
sseSpecification | SSESpecification | Set the ddb server-side encryption specification on the table. |
streamSpecification | StreamSpecification | Set the ddb stream specification on the table. |
timeToLiveAttribute | TimeToLiveSpecification | The name of TTL attribute. |
billingMode
Required public readonly billingMode: BillingMode;
Specify how you are charged for read and write throughput and how you manage capacity.
deletionProtectionEnabled
Required public readonly deletionProtectionEnabled: boolean;
Set table deletion protection.
pointInTimeRecoveryEnabled
Required public readonly pointInTimeRecoveryEnabled: boolean;
Whether point-in-time recovery is enabled.
provisionedThroughput
Required public readonly provisionedThroughput: ProvisionedThroughput;
Update the provisioned throughput for the base table.
sseSpecification
Required public readonly sseSpecification: SSESpecification;
Set the ddb server-side encryption specification on the table.
streamSpecification
Required public readonly streamSpecification: StreamSpecification;
Set the ddb stream specification on the table.
timeToLiveAttribute
Required public readonly timeToLiveAttribute: TimeToLiveSpecification;
The name of TTL attribute.
Class exposing utilities to produce IAmplifyGraphqlDefinition objects given various inputs.
import { AmplifyGraphqlDefinition } from '@aws-amplify/graphql-api-construct'
new AmplifyGraphqlDefinition()
Name | Type | Description |
---|
Name | Description |
---|---|
combine | Combines multiple IAmplifyGraphqlDefinitions into a single definition. |
fromFiles | Convert one or more appsync SchemaFile objects into an Amplify Graphql Schema, binding them to a DynamoDB data source. |
fromFilesAndStrategy | Convert one or more appsync SchemaFile objects into an Amplify Graphql Schema. |
fromString | Produce a schema definition from a string input. |
combine
import { AmplifyGraphqlDefinition } from '@aws-amplify/graphql-api-construct'
AmplifyGraphqlDefinition.combine(definitions: IAmplifyGraphqlDefinition[])
Combines multiple IAmplifyGraphqlDefinitions into a single definition.
definitions
Required the definitions to combine.
fromFiles
import { AmplifyGraphqlDefinition } from '@aws-amplify/graphql-api-construct'
AmplifyGraphqlDefinition.fromFiles(filePaths: string)
Convert one or more appsync SchemaFile objects into an Amplify Graphql Schema, binding them to a DynamoDB data source.
filePaths
Required one or more paths to the graphql files to process.
fromFilesAndStrategy
import { AmplifyGraphqlDefinition } from '@aws-amplify/graphql-api-construct'
AmplifyGraphqlDefinition.fromFilesAndStrategy(filePaths: string | string[], dataSourceStrategy?: DefaultDynamoDbModelDataSourceStrategy | AmplifyDynamoDbModelDataSourceStrategy | SQLLambdaModelDataSourceStrategy)
Convert one or more appsync SchemaFile objects into an Amplify Graphql Schema.
filePaths
Required one or more paths to the graphql files to process.
dataSourceStrategy
Optional the provisioning definition for datasources that resolve @model
s in this schema.
The DynamoDB from CloudFormation will be used by default.
fromString
import { AmplifyGraphqlDefinition } from '@aws-amplify/graphql-api-construct'
AmplifyGraphqlDefinition.fromString(schema: string, dataSourceStrategy?: DefaultDynamoDbModelDataSourceStrategy | AmplifyDynamoDbModelDataSourceStrategy | SQLLambdaModelDataSourceStrategy)
Produce a schema definition from a string input.
schema
Required the graphql input as a string.
dataSourceStrategy
Optional the provisioning definition for datasources that resolve @model
s and custom SQL statements in this schema.
The DynamoDB from CloudFormation will be used by default.
Class exposing utilities to produce SQLLambdaModelDataSourceStrategy objects given various inputs.
import { SQLLambdaModelDataSourceStrategyFactory } from '@aws-amplify/graphql-api-construct'
new SQLLambdaModelDataSourceStrategyFactory()
Name | Type | Description |
---|
Name | Description |
---|---|
fromCustomSqlFiles | Creates a SQLLambdaModelDataSourceStrategy where the binding's customSqlStatements are populated from sqlFiles . |
fromCustomSqlFiles
import { SQLLambdaModelDataSourceStrategyFactory } from '@aws-amplify/graphql-api-construct'
SQLLambdaModelDataSourceStrategyFactory.fromCustomSqlFiles(sqlFiles: string[], options: SQLLambdaModelDataSourceStrategy)
Creates a SQLLambdaModelDataSourceStrategy where the binding's customSqlStatements
are populated from sqlFiles
.
The key
of the customSqlStatements
record is the file's base name (that is, the name of the file minus the directory and extension).
sqlFiles
Required the list of files to load SQL statements from.
options
Required the remaining SQLLambdaModelDataSourceStrategy options.
Graphql Api definition, which can be implemented in multiple ways.
Name | Type | Description |
---|---|---|
dataSourceStrategies | {[ key: string ]: DefaultDynamoDbModelDataSourceStrategy | AmplifyDynamoDbModelDataSourceStrategy | SQLLambdaModelDataSourceStrategy} | Retrieve the datasource strategy mapping. |
functionSlots | MutationFunctionSlot | QueryFunctionSlot | SubscriptionFunctionSlot[] | Retrieve any function slots defined explicitly in the Api definition. |
schema | string | Return the schema definition as a graphql string, with amplify directives allowed. |
customSqlDataSourceStrategies | CustomSqlDataSourceStrategy[] | An array of custom Query or Mutation SQL commands to the data sources that resolves them. |
referencedLambdaFunctions | {[ key: string ]: aws-cdk-lib.aws_lambda.IFunction} | Retrieve the references to any lambda functions used in the definition. |
dataSourceStrategies
Required public readonly dataSourceStrategies: {[ key: string ]: DefaultDynamoDbModelDataSourceStrategy | AmplifyDynamoDbModelDataSourceStrategy | SQLLambdaModelDataSourceStrategy};
Retrieve the datasource strategy mapping.
The default strategy is to use DynamoDB from CloudFormation.
functionSlots
Required public readonly functionSlots: MutationFunctionSlot | QueryFunctionSlot | SubscriptionFunctionSlot[];
Retrieve any function slots defined explicitly in the Api definition.
schema
Required public readonly schema: string;
Return the schema definition as a graphql string, with amplify directives allowed.
customSqlDataSourceStrategies
Optional public readonly customSqlDataSourceStrategies: CustomSqlDataSourceStrategy[];
An array of custom Query or Mutation SQL commands to the data sources that resolves them.
referencedLambdaFunctions
Optional public readonly referencedLambdaFunctions: {[ key: string ]: IFunction};
Retrieve the references to any lambda functions used in the definition.
Useful for wiring through aws_lambda.Function constructs into the definition directly, and generated references to invoke them.
Entry representing the required output from the backend for codegen generate commands to work.
Name | Type | Description |
---|---|---|
payload | {[ key: string ]: string} | The string-map payload of generated config values. |
version | string | The protocol version for this backend output. |
payload
Required public readonly payload: {[ key: string ]: string};
The string-map payload of generated config values.
version
Required public readonly version: string;
The protocol version for this backend output.
Backend output strategy used to write config required for codegen tasks.
Name | Description |
---|---|
addBackendOutputEntry | Add an entry to backend output. |
addBackendOutputEntry
public addBackendOutputEntry(keyName: string, backendOutputEntry: IBackendOutputEntry): void
Add an entry to backend output.
keyName
Required the key.
backendOutputEntry
Required the record to store in the backend output.
Server Side Encryption Type Values - KMS
- Server-side encryption that uses AWS KMS.
The key is stored in your account and is managed by KMS (AWS KMS charges apply).
Name | Description |
---|---|
KMS | No description. |
KMS
FAQs
AppSync GraphQL Api Construct using Amplify GraphQL Transformer.
We found that @aws-amplify/graphql-api-construct demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.
Security News
Bun 1.2 enhances its JavaScript runtime with 90% Node.js compatibility, built-in S3 and Postgres support, HTML Imports, and faster, cloud-first performance.