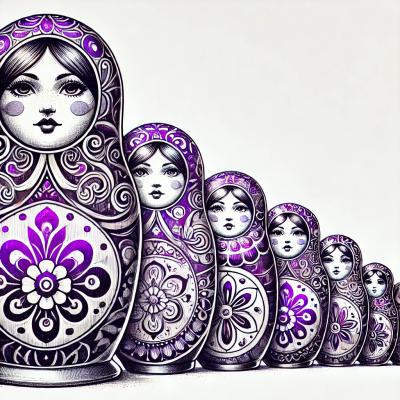
Research
Security News
Threat Actor Exposes Playbook for Exploiting npm to Build Blockchain-Powered Botnets
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
@aws-cdk/aws-dynamodb
Advanced tools
Here is a minimal deployable DynamoDB table definition:
const table = new dynamodb.Table(this, 'Table', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
});
To import an existing table into your CDK application, use the Table.fromTableName
, Table.fromTableArn
or Table.fromTableAttributes
factory method. This method accepts table name or table ARN which describes the properties of an already
existing table:
declare const user: iam.User;
const table = dynamodb.Table.fromTableArn(this, 'ImportedTable', 'arn:aws:dynamodb:us-east-1:111111111:table/my-table');
// now you can just call methods on the table
table.grantReadWriteData(user);
If you intend to use the tableStreamArn
(including indirectly, for example by creating an
@aws-cdk/aws-lambda-event-source.DynamoEventSource
on the imported table), you must use the
Table.fromTableAttributes
method and the tableStreamArn
property must be populated.
When a table is defined, you must define it's schema using the partitionKey
(required) and sortKey
(optional) properties.
DynamoDB supports two billing modes:
const table = new dynamodb.Table(this, 'Table', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
billingMode: dynamodb.BillingMode.PAY_PER_REQUEST,
});
Further reading: https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/HowItWorks.ReadWriteCapacityMode.
DynamoDB supports two table classes:
const table = new dynamodb.Table(this, 'Table', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
tableClass: dynamodb.TableClass.STANDARD_INFREQUENT_ACCESS,
});
Further reading: https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/HowItWorks.TableClasses.html
You can have DynamoDB automatically raise and lower the read and write capacities of your table by setting up autoscaling. You can use this to either keep your tables at a desired utilization level, or by scaling up and down at pre-configured times of the day:
Auto-scaling is only relevant for tables with the billing mode, PROVISIONED.
Example of configuring autoscaling
Further reading: https://docs.aws.amazon.com/amazondynamodb/latest/developerguide/AutoScaling.html https://aws.amazon.com/blogs/database/how-to-use-aws-cloudformation-to-configure-auto-scaling-for-amazon-dynamodb-tables-and-indexes/
You can create DynamoDB Global Tables by setting the replicationRegions
property on a Table
:
const globalTable = new dynamodb.Table(this, 'Table', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
replicationRegions: ['us-east-1', 'us-east-2', 'us-west-2'],
});
When doing so, a CloudFormation Custom Resource will be added to the stack in order to create the replica tables in the selected regions.
The default billing mode for Global Tables is PAY_PER_REQUEST
.
If you want to use PROVISIONED
,
you have to make sure write auto-scaling is enabled for that Table:
const globalTable = new dynamodb.Table(this, 'Table', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
replicationRegions: ['us-east-1', 'us-east-2', 'us-west-2'],
billingMode: dynamodb.BillingMode.PROVISIONED,
});
globalTable.autoScaleWriteCapacity({
minCapacity: 1,
maxCapacity: 10,
}).scaleOnUtilization({ targetUtilizationPercent: 75 });
When adding a replica region for a large table, you might want to increase the timeout for the replication operation:
const globalTable = new dynamodb.Table(this, 'Table', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
replicationRegions: ['us-east-1', 'us-east-2', 'us-west-2'],
replicationTimeout: Duration.hours(2), // defaults to Duration.minutes(30)
});
All user data stored in Amazon DynamoDB is fully encrypted at rest. When creating a new table, you can choose to encrypt using the following customer master keys (CMK) to encrypt your table:
Creating a Table encrypted with a customer managed CMK:
const table = new dynamodb.Table(this, 'MyTable', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
encryption: dynamodb.TableEncryption.CUSTOMER_MANAGED,
});
// You can access the CMK that was added to the stack on your behalf by the Table construct via:
const tableEncryptionKey = table.encryptionKey;
You can also supply your own key:
import * as kms from '@aws-cdk/aws-kms';
const encryptionKey = new kms.Key(this, 'Key', {
enableKeyRotation: true,
});
const table = new dynamodb.Table(this, 'MyTable', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
encryption: dynamodb.TableEncryption.CUSTOMER_MANAGED,
encryptionKey, // This will be exposed as table.encryptionKey
});
In order to use the AWS managed CMK instead, change the code to:
const table = new dynamodb.Table(this, 'MyTable', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
encryption: dynamodb.TableEncryption.AWS_MANAGED,
});
// In this case, the CMK _cannot_ be accessed through table.encryptionKey.
To get the partition key and sort key of the table or indexes you have configured:
declare const table: dynamodb.Table;
const schema = table.schema();
const partitionKey = schema.partitionKey;
const sortKey = schema.sortKey;
// In case you want to get schema details for any secondary index
// const { partitionKey, sortKey } = table.schema(INDEX_NAME);
A Kinesis Data Stream can be configured on the DynamoDB table to capture item-level changes.
import * as kinesis from '@aws-cdk/aws-kinesis';
const stream = new kinesis.Stream(this, 'Stream');
const table = new dynamodb.Table(this, 'Table', {
partitionKey: { name: 'id', type: dynamodb.AttributeType.STRING },
kinesisStream: stream,
});
FAQs
The CDK Construct Library for AWS::DynamoDB
We found that @aws-cdk/aws-dynamodb demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A threat actor's playbook for exploiting the npm ecosystem was exposed on the dark web, detailing how to build a blockchain-powered botnet.
Security News
NVD’s backlog surpasses 20,000 CVEs as analysis slows and NIST announces new system updates to address ongoing delays.
Security News
Research
A malicious npm package disguised as a WhatsApp client is exploiting authentication flows with a remote kill switch to exfiltrate data and destroy files.