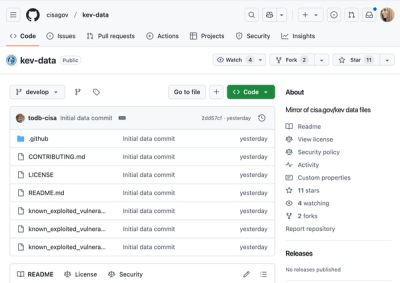
Security News
CISA Brings KEV Data to GitHub
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
@aws-cdk/aws-scheduler-targets-alpha
Advanced tools
The CDK Construct Library for Amazon Scheduler Targets
The APIs of higher level constructs in this module are in developer preview before they become stable. We will only make breaking changes to address unforeseen API issues. Therefore, these APIs are not subject to Semantic Versioning, and breaking changes will be announced in release notes. This means that while you may use them, you may need to update your source code when upgrading to a newer version of this package.
Amazon EventBridge Scheduler is a feature from Amazon EventBridge that allows you to create, run, and manage scheduled tasks at scale. With EventBridge Scheduler, you can schedule millions of one-time or recurring tasks across various AWS services without provisioning or managing underlying infrastructure.
This library contains integration classes for Amazon EventBridge Scheduler to call any number of supported AWS Services.
The following targets are supported:
targets.LambdaInvoke
: Invoke an AWS Lambda functiontargets.StepFunctionsStartExecution
: Start an AWS Step Functiontargets.CodeBuildStartBuild
: Start a CodeBuild jobtargets.SqsSendMessage
: Send a Message to an Amazon SQS Queuetargets.SnsPublish
: Publish messages to an Amazon SNS topictargets.EventBridgePutEvents
: Put Events on EventBridgetargets.InspectorStartAssessmentRun
: Start an Amazon Inspector assessment runtargets.KinesisStreamPutRecord
: Put a record to an Amazon Kinesis Data Streamtargets.KinesisDataFirehosePutRecord
: Put a record to a Kinesis Data Firehosetargets.CodePipelineStartPipelineExecution
: Start a CodePipeline executiontargets.SageMakerStartPipelineExecution
: Start a SageMaker pipeline executiontargets.Universal
: Invoke a wider set of AWS APIUse the LambdaInvoke
target to invoke a lambda function.
The code snippet below creates an event rule with a Lambda function as a target called every hour by EventBridge Scheduler with a custom payload. You can optionally attach a dead letter queue.
import * as lambda from 'aws-cdk-lib/aws-lambda';
const fn = new lambda.Function(this, 'MyFunc', {
runtime: lambda.Runtime.NODEJS_LATEST,
handler: 'index.handler',
code: lambda.Code.fromInline(`exports.handler = handler.toString()`),
});
const dlq = new sqs.Queue(this, "DLQ", {
queueName: 'MyDLQ',
});
const target = new targets.LambdaInvoke(fn, {
deadLetterQueue: dlq,
maxEventAge: Duration.minutes(1),
retryAttempts: 3,
input: ScheduleTargetInput.fromObject({
'payload': 'useful'
}),
});
const schedule = new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.hours(1)),
target
});
Use the StepFunctionsStartExecution
target to start a new execution on a StepFunction.
The code snippet below creates an event rule with a Step Function as a target called every hour by EventBridge Scheduler with a custom payload.
import * as sfn from 'aws-cdk-lib/aws-stepfunctions';
import * as tasks from 'aws-cdk-lib/aws-stepfunctions-tasks';
const payload = {
Name: "MyParameter",
Value: '🌥️',
};
const putParameterStep = new tasks.CallAwsService(this, 'PutParameter', {
service: 'ssm',
action: 'putParameter',
iamResources: ['*'],
parameters: {
"Name.$": '$.Name',
"Value.$": '$.Value',
Type: 'String',
Overwrite: true,
},
});
const stateMachine = new sfn.StateMachine(this, 'StateMachine', {
definitionBody: sfn.DefinitionBody.fromChainable(putParameterStep)
});
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.hours(1)),
target: new targets.StepFunctionsStartExecution(stateMachine, {
input: ScheduleTargetInput.fromObject(payload),
}),
});
Use the CodeBuildStartBuild
target to start a new build run on a CodeBuild project.
The code snippet below creates an event rule with a CodeBuild project as target which is called every hour by EventBridge Scheduler.
import * as codebuild from 'aws-cdk-lib/aws-codebuild';
declare const project: codebuild.Project;
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.minutes(60)),
target: new targets.CodeBuildStartBuild(project),
});
Use the SqsSendMessage
target to send a message to an SQS Queue.
The code snippet below creates an event rule with an SQS Queue as a target called every hour by EventBridge Scheduler with a custom payload.
Contains the messageGroupId
to use when the target is a FIFO queue. If you specify
a FIFO queue as a target, the queue must have content-based deduplication enabled.
const payload = 'test';
const messageGroupId = 'id';
const queue = new sqs.Queue(this, 'MyQueue', {
fifo: true,
contentBasedDeduplication: true,
});
const target = new targets.SqsSendMessage(queue, {
input: ScheduleTargetInput.fromText(payload),
messageGroupId,
});
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.minutes(1)),
target
});
Use the SnsPublish
target to publish messages to an Amazon SNS topic.
The code snippets below create an event rule with a Amazon SNS topic as a target. It's called every hour by Amazon EventBridge Scheduler with a custom payload.
import * as sns from 'aws-cdk-lib/aws-sns';
const topic = new sns.Topic(this, 'Topic');
const payload = {
message: 'Hello scheduler!',
};
const target = new targets.SnsPublish(topic, {
input: ScheduleTargetInput.fromObject(payload),
});
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.hours(1)),
target,
});
Use the EventBridgePutEvents
target to send events to an EventBridge event bus.
The code snippet below creates an event rule with an EventBridge event bus as a target called every hour by EventBridge Scheduler with a custom event payload.
import * as events from 'aws-cdk-lib/aws-events';
const eventBus = new events.EventBus(this, 'EventBus', {
eventBusName: 'DomainEvents',
});
const eventEntry: targets.EventBridgePutEventsEntry = {
eventBus,
source: 'PetService',
detail: ScheduleTargetInput.fromObject({ Name: 'Fluffy' }),
detailType: '🐶',
};
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.hours(1)),
target: new targets.EventBridgePutEvents(eventEntry),
});
Use the InspectorStartAssessmentRun
target to start an Inspector assessment run.
The code snippet below creates an event rule with an assessment template as the target which is called every hour by EventBridge Scheduler.
import * as inspector from 'aws-cdk-lib/aws-inspector';
declare const assessmentTemplate: inspector.CfnAssessmentTemplate;
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.minutes(60)),
target: new targets.InspectorStartAssessmentRun(assessmentTemplate),
});
Use the KinesisStreamPutRecord
target to put a record to an Amazon Kinesis Data Stream.
The code snippet below creates an event rule with a stream as the target which is called every hour by EventBridge Scheduler.
import * as kinesis from 'aws-cdk-lib/aws-kinesis';
const stream = new kinesis.Stream(this, 'MyStream');
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.minutes(60)),
target: new targets.KinesisStreamPutRecord(stream, {
partitionKey: 'key',
}),
});
Use the KinesisDataFirehosePutRecord
target to put a record to a Kinesis Data Firehose delivery stream.
The code snippet below creates an event rule with a delivery stream as a target called every hour by EventBridge Scheduler with a custom payload.
import * as firehose from '@aws-cdk/aws-kinesisfirehose-alpha';
declare const deliveryStream: firehose.IDeliveryStream;
const payload = {
Data: "record",
};
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.minutes(60)),
target: new targets.KinesisDataFirehosePutRecord(deliveryStream, {
input: ScheduleTargetInput.fromObject(payload),
}),
});
Use the CodePipelineStartPipelineExecution
target to start a new execution for a CodePipeline pipeline.
The code snippet below creates an event rule with a CodePipeline pipeline as the target which is called every hour by EventBridge Scheduler.
import * as codepipeline from 'aws-cdk-lib/aws-codepipeline';
declare const pipeline: codepipeline.Pipeline;
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.minutes(60)),
target: new targets.CodePipelineStartPipelineExecution(pipeline),
});
Use the SageMakerStartPipelineExecution
target to start a new execution for a SageMaker pipeline.
The code snippet below creates an event rule with a SageMaker pipeline as the target which is called every hour by EventBridge Scheduler.
import * as sagemaker from 'aws-cdk-lib/aws-sagemaker';
declare const pipeline: sagemaker.IPipeline;
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.minutes(60)),
target: new targets.SageMakerStartPipelineExecution(pipeline, {
pipelineParameterList: [{
name: 'parameter-name',
value: 'parameter-value',
}],
}),
});
Use the Universal
target to invoke AWS API. See https://docs.aws.amazon.com/scheduler/latest/UserGuide/managing-targets-universal.html
The code snippet below creates an event rule with AWS API as the target which is called at midnight every day by EventBridge Scheduler.
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.cron({
minute: '0',
hour: '0',
}),
target: new targets.Universal({
service: 'rds',
action: 'stopDBCluster',
input: ScheduleTargetInput.fromObject({
DbClusterIdentifier: 'my-db',
}),
}),
});
The service
must be in lowercase and the action
must be in camelCase.
By default, an IAM policy for the Scheduler is extracted from the API call. The action in the policy is constructed using the service
and action
prop.
Re-using the example above, the action will be rds:stopDBCluster
. Note that not all IAM actions follow the same pattern. In such scenario, please use the
policyStatements
prop to override the policy:
new Schedule(this, 'Schedule', {
schedule: ScheduleExpression.rate(Duration.minutes(60)),
target: new targets.Universal({
service: 'sqs',
action: 'sendMessage',
policyStatements: [
new iam.PolicyStatement({
actions: ['sqs:SendMessage'],
resources: ['arn:aws:sqs:us-east-1:123456789012:my_queue'],
}),
new iam.PolicyStatement({
actions: ['kms:Decrypt', 'kms:GenerateDataKey*'],
resources: ['arn:aws:kms:us-east-1:123456789012:key/0987dcba-09fe-87dc-65ba-ab0987654321'],
}),
],
}),
});
Note: The default policy uses
*
in the resources field as CDK does not have a straight forward way to auto-discover the resources permission required. It is recommended that you scope the field down to specific resources to have a better security posture.
FAQs
The CDK Construct Library for Amazon Scheduler Targets
The npm package @aws-cdk/aws-scheduler-targets-alpha receives a total of 23,335 weekly downloads. As such, @aws-cdk/aws-scheduler-targets-alpha popularity was classified as popular.
We found that @aws-cdk/aws-scheduler-targets-alpha demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA's KEV data is now on GitHub, offering easier access, API integration, commit history tracking, and automated updates for security teams and researchers.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.