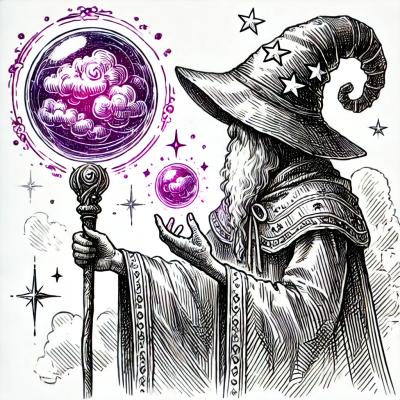
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@aws-crypto/crc32c
Advanced tools
Pure JS implementation of CRC32-C https://en.wikipedia.org/wiki/Cyclic_redundancy_check
The @aws-crypto/crc32c package is designed for calculating CRC32C (Cyclic Redundancy Check) checksums. This is particularly useful for verifying the integrity of data, such as files or network packets, to ensure they have not been altered during transmission or storage. The CRC32C algorithm is known for its efficiency and error-detection capabilities, making it a popular choice for error-checking applications.
Calculate CRC32C checksum from a string
This feature allows you to calculate the CRC32C checksum of a given string. The input string is first converted to a Buffer, and then the `crc32c` function is used to calculate the checksum. The result is a number that represents the checksum, which can be converted to a hexadecimal string for easier reading or comparison.
"use strict";
const { crc32c } = require('@aws-crypto/crc32c');
const input = Buffer.from('Hello World');
const checksum = crc32c(input);
console.log(`CRC32C Checksum: ${checksum.toString(16)}`);
Calculate CRC32C checksum from a file
This feature demonstrates how to calculate the CRC32C checksum of a file. The file is read into a Buffer using Node.js's `fs.readFileSync` method, and then the `crc32c` function is applied to this Buffer to compute the checksum. This is useful for verifying the integrity of files.
"use strict";
const fs = require('fs');
const { crc32c } = require('@aws-crypto/crc32c');
const fileBuffer = fs.readFileSync('path/to/your/file.txt');
const checksum = crc32c(fileBuffer);
console.log(`CRC32C Checksum of the file: ${checksum.toString(16)}`);
The 'fast-crc32c' package is another Node.js module for computing CRC32C checksums. It offers similar functionality to '@aws-crypto/crc32c' but focuses on performance optimizations for Node.js environments. It uses native bindings to Google's Snappy project for faster computation, making it a good alternative for performance-critical applications.
While 'buffer-crc32' computes CRC32 checksums rather than CRC32C, it serves a similar purpose in verifying data integrity. The main difference lies in the algorithm used for the checksum calculation. 'buffer-crc32' might be preferred in scenarios where CRC32 is the required standard, but '@aws-crypto/crc32c' is specifically tailored for CRC32C computations.
Pure JS implementation of CRC32-C https://en.wikipedia.org/wiki/Cyclic_redundancy_check
import { Crc32c } from '@aws-crypto/crc32c';
const crc32Digest = (new Crc32c).update(buffer).digest()
npm test
FAQs
Pure JS implementation of CRC32-C https://en.wikipedia.org/wiki/Cyclic_redundancy_check
The npm package @aws-crypto/crc32c receives a total of 4,898,516 weekly downloads. As such, @aws-crypto/crc32c popularity was classified as popular.
We found that @aws-crypto/crc32c demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.