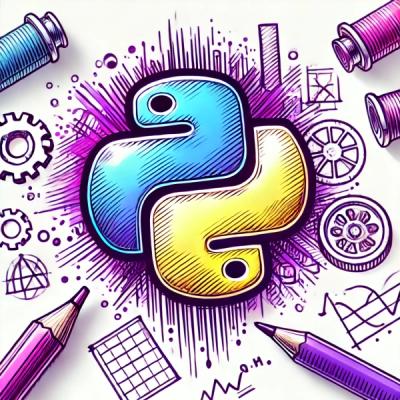
Security News
New Python Packaging Proposal Aims to Solve Phantom Dependency Problem with SBOMs
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
@aws-lambda-powertools/idempotency
Advanced tools
The idempotency package for the Powertools for AWS Lambda (TypeScript) library. It provides options to make your Lambda functions idempotent and safe to retry.
Powertools for AWS Lambda (TypeScript) is a developer toolkit to implement Serverless best practices and increase developer velocity.
You can use the package in both TypeScript and JavaScript code bases.
This package provides a utility to implement idempotency in your Lambda functions. You can either use it to wrap a function, or as Middy middleware to make your AWS Lambda handler idempotent.
The current implementation provides a persistence layer for Amazon DynamoDB, which offers a variety of configuration options. You can also bring your own persistence layer by extending the BasePersistenceLayer
class.
To get started, install the library by running:
npm i @aws-lambda-powertools/idempotency @aws-sdk/client-dynamodb @aws-sdk/lib-dynamodb
Next, review the IAM permissions attached to your AWS Lambda function and make sure you allow the actions detailed in the documentation of the utility.
You can make any function idempotent, and safe to retry, by wrapping it using the makeIdempotent
higher-order function.
The function wrapper takes a reference to the function to be made idempotent as first argument, and an object with options as second argument.
When you wrap your Lambda handler function, the utility uses the content of the event
parameter to handle the idempotency logic.
import { makeIdempotent } from '@aws-lambda-powertools/idempotency';
import { DynamoDBPersistenceLayer } from '@aws-lambda-powertools/idempotency/dynamodb';
import type { Context, APIGatewayProxyEvent } from 'aws-lambda';
const persistenceStore = new DynamoDBPersistenceLayer({
tableName: 'idempotencyTableName',
});
const myHandler = async (
event: APIGatewayProxyEvent,
_context: Context
): Promise<void> => {
// your code goes here here
};
export const handler = makeIdempotent(myHandler, {
persistenceStore,
});
You can also use the makeIdempotent
function to wrap any other arbitrary function, not just Lambda handlers.
import { makeIdempotent } from '@aws-lambda-powertools/idempotency';
import { DynamoDBPersistenceLayer } from '@aws-lambda-powertools/idempotency/dynamodb';
import type { Context, SQSEvent, SQSRecord } from 'aws-lambda';
const persistenceStore = new DynamoDBPersistenceLayer({
tableName: 'idempotencyTableName',
});
const processingFunction = async (payload: SQSRecord): Promise<void> => {
// your code goes here here
};
const processIdempotently = makeIdempotent(processingFunction, {
persistenceStore,
});
export const handler = async (
event: SQSEvent,
_context: Context
): Promise<void> => {
for (const record of event.Records) {
await processIdempotently(record);
}
};
If your function has multiple arguments, you can use the dataIndexArgument
option to specify which argument should be used as the idempotency key.
import { makeIdempotent } from '@aws-lambda-powertools/idempotency';
import { DynamoDBPersistenceLayer } from '@aws-lambda-powertools/idempotency/dynamodb';
import type { Context, SQSEvent, SQSRecord } from 'aws-lambda';
const persistenceStore = new DynamoDBPersistenceLayer({
tableName: 'idempotencyTableName',
});
const processingFunction = async (payload: SQSRecord, customerId: string): Promise<void> => {
// your code goes here here
};
const processIdempotently = makeIdempotent(processingFunction, {
persistenceStore,
// this tells the utility to use the second argument (`customerId`) as the idempotency key
dataIndexArgument: 1,
});
export const handler = async (
event: SQSEvent,
_context: Context
): Promise<void> => {
for (const record of event.Records) {
await processIdempotently(record, 'customer-123');
}
};
Note that you can also specify a JMESPath expression in the Idempotency config object to select a subset of the event payload as the idempotency key. This is useful when dealing with payloads that contain timestamps or request ids.
import { makeIdempotent, IdempotencyConfig } from '@aws-lambda-powertools/idempotency';
import { DynamoDBPersistenceLayer } from '@aws-lambda-powertools/idempotency/dynamodb';
import type { Context, APIGatewayProxyEvent } from 'aws-lambda';
const persistenceStore = new DynamoDBPersistenceLayer({
tableName: 'idempotencyTableName',
});
const myHandler = async (
event: APIGatewayProxyEvent,
_context: Context
): Promise<void> => {
// your code goes here here
};
export const handler = makeIdempotent(myHandler, {
persistenceStore,
config: new IdempotencyConfig({
eventKeyJmespath: 'requestContext.identity.user',
}),
});
Check the docs for more examples.
If instead you use Middy, you can use the makeHandlerIdempotent
middleware. When using the middleware your Lambda handler becomes idempotent.
By default, the Idempotency utility will use the full event payload to create an hash and determine if a request is idempotent, and therefore it should not be retried. When dealing with a more elaborate payload, where parts of the payload always change you should use the IdempotencyConfig
object to instruct the utility to only use a portion of your payload. This is useful when dealing with payloads that contain timestamps or request ids.
import { IdempotencyConfig } from '@aws-lambda-powertools/idempotency';
import { makeHandlerIdempotent } from '@aws-lambda-powertools/idempotency/middleware';
import { DynamoDBPersistenceLayer } from '@aws-lambda-powertools/idempotency/dynamodb';
import middy from '@middy/core';
import type { Context, APIGatewayProxyEvent } from 'aws-lambda';
const persistenceStore = new DynamoDBPersistenceLayer({
tableName: 'idempotencyTableName',
});
const config = new IdempotencyConfig({
hashFunction: 'md5',
useLocalCache: false,
expiresAfterSeconds: 3600,
throwOnNoIdempotencyKey: false,
eventKeyJmesPath: 'headers.idempotency-key',
});
export const handler = middy(
async (_event: APIGatewayProxyEvent, _context: Context): Promise<void> => {
// your code goes here here
}
).use(
makeHandlerIdempotent({
config,
persistenceStore,
})
);
Check the docs for more examples.
You can use a DynamoDB Table to store the idempotency information. This enables you to keep track of the hash key, payload, status for progress, expiration, and much more.
You can customize most of the configuration options of the table, i.e the names of the attributes. See the API documentation for more details.
If you are interested in contributing to this project, please refer to our Contributing Guidelines.
The roadmap of Powertools for AWS Lambda (TypeScript) is driven by customers’ demand.
Help us prioritize upcoming functionalities or utilities by upvoting existing RFCs and feature requests, or creating new ones, in this GitHub repository.
#typescript
- Invite linkKnowing which companies are using this library is important to help prioritize the project internally. If your company is using Powertools for AWS Lambda (TypeScript), you can request to have your name and logo added to the README file by raising a Support Powertools for AWS Lambda (TypeScript) (become a reference) issue.
The following companies, among others, use Powertools:
Share what you did with Powertools for AWS Lambda (TypeScript) 💞💞. Blog post, workshops, presentation, sample apps and others. Check out what the community has already shared about Powertools for AWS Lambda (TypeScript) here.
This helps us understand who uses Powertools for AWS Lambda (TypeScript) in a non-intrusive way, and helps us gain future investments for other Powertools for AWS Lambda languages. When using Layers, you can add Powertools as a dev dependency (or as part of your virtual env) to not impact the development process.
Credits for the Lambda Powertools for AWS Lambda (TypeScript) idea go to DAZN and their DAZN Lambda Powertools.
This library is licensed under the MIT-0 License. See the LICENSE file.
FAQs
The idempotency package for the Powertools for AWS Lambda (TypeScript) library. It provides options to make your Lambda functions idempotent and safe to retry.
The npm package @aws-lambda-powertools/idempotency receives a total of 4,793 weekly downloads. As such, @aws-lambda-powertools/idempotency popularity was classified as popular.
We found that @aws-lambda-powertools/idempotency demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.
Security News
Socket CEO Feross Aboukhadijeh discusses open source security challenges, including zero-day attacks and supply chain risks, on the Cyber Security Council podcast.
Security News
Research
Socket researchers uncover how threat actors weaponize Out-of-Band Application Security Testing (OAST) techniques across the npm, PyPI, and RubyGems ecosystems to exfiltrate sensitive data.