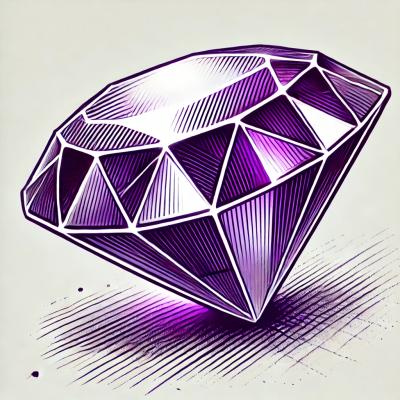
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
@aws-sdk/client-acm
Advanced tools
AWS SDK for JavaScript Acm Client for Node.js, Browser and React Native
@aws-sdk/client-acm is a part of the AWS SDK for JavaScript, which allows developers to interact with the AWS Certificate Manager (ACM) service. This package provides methods to request, manage, and deploy SSL/TLS certificates for use with AWS services and internal connected resources.
Request a Certificate
This feature allows you to request a new SSL/TLS certificate for a specified domain. The code sample demonstrates how to request a certificate for 'example.com' using email validation.
const { ACMClient, RequestCertificateCommand } = require('@aws-sdk/client-acm');
const client = new ACMClient({ region: 'us-west-2' });
const command = new RequestCertificateCommand({
DomainName: 'example.com',
ValidationMethod: 'EMAIL',
});
client.send(command).then(
(data) => console.log(data),
(error) => console.error(error)
);
List Certificates
This feature allows you to list all the certificates in your AWS account. The code sample demonstrates how to retrieve and log the list of certificates.
const { ACMClient, ListCertificatesCommand } = require('@aws-sdk/client-acm');
const client = new ACMClient({ region: 'us-west-2' });
const command = new ListCertificatesCommand({});
client.send(command).then(
(data) => console.log(data),
(error) => console.error(error)
);
Describe Certificate
This feature allows you to get detailed information about a specific certificate. The code sample demonstrates how to describe a certificate using its ARN.
const { ACMClient, DescribeCertificateCommand } = require('@aws-sdk/client-acm');
const client = new ACMClient({ region: 'us-west-2' });
const command = new DescribeCertificateCommand({
CertificateArn: 'arn:aws:acm:us-west-2:123456789012:certificate/abcd1234-5678-90ab-cdef-EXAMPLE11111',
});
client.send(command).then(
(data) => console.log(data),
(error) => console.error(error)
);
Delete Certificate
This feature allows you to delete a specified certificate. The code sample demonstrates how to delete a certificate using its ARN.
const { ACMClient, DeleteCertificateCommand } = require('@aws-sdk/client-acm');
const client = new ACMClient({ region: 'us-west-2' });
const command = new DeleteCertificateCommand({
CertificateArn: 'arn:aws:acm:us-west-2:123456789012:certificate/abcd1234-5678-90ab-cdef-EXAMPLE11111',
});
client.send(command).then(
(data) => console.log(data),
(error) => console.error(error)
);
node-acme is a client library for the ACME protocol, which is used by Let's Encrypt to automate the process of obtaining SSL/TLS certificates. Unlike @aws-sdk/client-acm, which is specific to AWS Certificate Manager, node-acme can be used to interact with any ACME-compliant certificate authority.
acme-client is another library for the ACME protocol, providing a simple and flexible way to interact with ACME servers like Let's Encrypt. It offers similar functionalities to node-acme but with a different API design. It is not tied to AWS services and can be used with any ACME-compliant CA.
FAQs
AWS SDK for JavaScript Acm Client for Node.js, Browser and React Native
We found that @aws-sdk/client-acm demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.