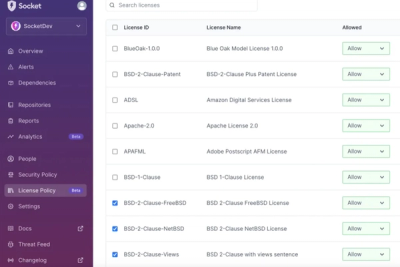
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
@aws-sdk/client-cloudformation
Advanced tools
The @aws-sdk/client-cloudformation npm package is a client library for AWS CloudFormation service. It allows developers to interact with the AWS CloudFormation service programmatically, enabling them to create, manage, and deploy AWS CloudFormation stacks and resources directly from their JavaScript or TypeScript applications.
Managing Stacks
This feature allows you to manage AWS CloudFormation stacks, including creating, updating, and deleting stacks.
const { CloudFormationClient, CreateStackCommand } = require('@aws-sdk/client-cloudformation');
const client = new CloudFormationClient({ region: 'us-west-2' });
const createStackParams = {
StackName: 'MyStack',
TemplateBody: 'JSON or YAML template',
};
const command = new CreateStackCommand(createStackParams);
client.send(command).then((data) => {
console.log('Stack creation initiated:', data);
}).catch((error) => {
console.error('Error creating stack:', error);
});
Describing Stack Resources
This feature allows you to retrieve information about the resources within a specific stack.
const { CloudFormationClient, DescribeStackResourcesCommand } = require('@aws-sdk/client-cloudformation');
const client = new CloudFormationClient({ region: 'us-west-2' });
const describeStackResourcesParams = {
StackName: 'MyStack'
};
const command = new DescribeStackResourcesCommand(describeStackResourcesParams);
client.send(command).then((data) => {
console.log('Stack resources:', data);
}).catch((error) => {
console.error('Error describing stack resources:', error);
});
Listing Stacks
This feature allows you to list all stacks and their statuses in your AWS account.
const { CloudFormationClient, ListStacksCommand } = require('@aws-sdk/client-cloudformation');
const client = new CloudFormationClient({ region: 'us-west-2' });
const command = new ListStacksCommand({});
client.send(command).then((data) => {
console.log('List of stacks:', data);
}).catch((error) => {
console.error('Error listing stacks:', error);
});
The 'aws-sdk' package is the predecessor of the '@aws-sdk/client-cloudformation' and provides a comprehensive AWS SDK for JavaScript. It supports CloudFormation operations but is not modular, meaning it includes the entire AWS SDK rather than just the CloudFormation service.
The 'serverless' package is a framework for building serverless applications using AWS Lambda and other cloud providers. It includes functionality for managing infrastructure as code, similar to CloudFormation, but it is more opinionated and provides a higher-level abstraction for deploying serverless applications.
The 'pulumi' package is a modern infrastructure as code SDK that allows you to define cloud resources using general-purpose programming languages. It is similar to CloudFormation in that it manages cloud resources but offers a different approach by using familiar programming languages and real code to define infrastructure.
FAQs
AWS SDK for JavaScript Cloudformation Client for Node.js, Browser and React Native
The npm package @aws-sdk/client-cloudformation receives a total of 981,209 weekly downloads. As such, @aws-sdk/client-cloudformation popularity was classified as popular.
We found that @aws-sdk/client-cloudformation demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.