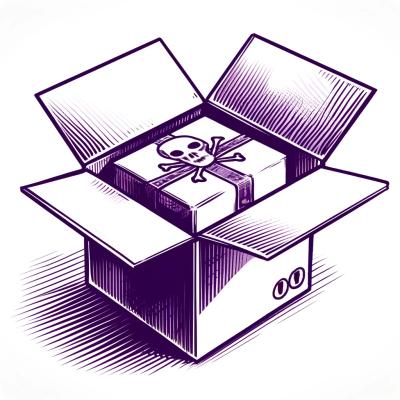
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@aws-sdk/client-redshift-data
Advanced tools
AWS SDK for JavaScript Redshift Data Client for Node.js, Browser and React Native
@aws-sdk/client-redshift-data is an AWS SDK for JavaScript package that allows you to interact with Amazon Redshift Data API. This package provides functionalities to execute SQL commands, manage database connections, and retrieve query results from Amazon Redshift clusters.
Execute SQL Statements
This feature allows you to execute SQL statements on your Amazon Redshift cluster. The code sample demonstrates how to execute a simple SELECT query.
const { RedshiftDataClient, ExecuteStatementCommand } = require('@aws-sdk/client-redshift-data');
const client = new RedshiftDataClient({ region: 'us-west-2' });
const params = {
ClusterIdentifier: 'my-cluster',
Database: 'my-database',
Sql: 'SELECT * FROM my_table',
DbUser: 'my-db-user'
};
const command = new ExecuteStatementCommand(params);
client.send(command).then(
(data) => console.log(data),
(error) => console.error(error)
);
Retrieve Query Results
This feature allows you to retrieve the results of a previously executed SQL statement. The code sample demonstrates how to get the results using the statement ID.
const { RedshiftDataClient, GetStatementResultCommand } = require('@aws-sdk/client-redshift-data');
const client = new RedshiftDataClient({ region: 'us-west-2' });
const params = {
Id: 'statement-id'
};
const command = new GetStatementResultCommand(params);
client.send(command).then(
(data) => console.log(data),
(error) => console.error(error)
);
List Databases
This feature allows you to list all databases in your Amazon Redshift cluster. The code sample demonstrates how to list databases using the cluster identifier and database user.
const { RedshiftDataClient, ListDatabasesCommand } = require('@aws-sdk/client-redshift-data');
const client = new RedshiftDataClient({ region: 'us-west-2' });
const params = {
ClusterIdentifier: 'my-cluster',
DbUser: 'my-db-user'
};
const command = new ListDatabasesCommand(params);
client.send(command).then(
(data) => console.log(data),
(error) => console.error(error)
);
List Tables
This feature allows you to list all tables in a specified database within your Amazon Redshift cluster. The code sample demonstrates how to list tables using the cluster identifier, database name, and database user.
const { RedshiftDataClient, ListTablesCommand } = require('@aws-sdk/client-redshift-data');
const client = new RedshiftDataClient({ region: 'us-west-2' });
const params = {
ClusterIdentifier: 'my-cluster',
Database: 'my-database',
DbUser: 'my-db-user'
};
const command = new ListTablesCommand(params);
client.send(command).then(
(data) => console.log(data),
(error) => console.error(error)
);
The 'pg' package is a PostgreSQL client for Node.js. It allows you to interact with PostgreSQL databases, including Amazon Redshift, by executing SQL queries, managing connections, and retrieving results. Compared to @aws-sdk/client-redshift-data, 'pg' is more general-purpose and not specifically tailored for Redshift Data API.
Sequelize is a promise-based Node.js ORM for various SQL databases, including PostgreSQL. It provides a higher-level abstraction for database operations, including model definitions, associations, and query building. While it can be used with Amazon Redshift, it is not specifically designed for Redshift Data API like @aws-sdk/client-redshift-data.
Knex.js is a SQL query builder for Node.js that supports multiple database types, including PostgreSQL. It provides a flexible and powerful way to build and execute SQL queries. While it can be used with Amazon Redshift, it does not offer the same level of integration with Redshift Data API as @aws-sdk/client-redshift-data.
AWS SDK for JavaScript RedshiftData Client for Node.js, Browser and React Native.
You can use the Amazon Redshift Data API to run queries on Amazon Redshift tables. You can run SQL statements, which are committed if the statement succeeds.
For more information about the Amazon Redshift Data API and CLI usage examples, see Using the Amazon Redshift Data API in the Amazon Redshift Management Guide.
To install this package, simply type add or install @aws-sdk/client-redshift-data using your favorite package manager:
npm install @aws-sdk/client-redshift-data
yarn add @aws-sdk/client-redshift-data
pnpm add @aws-sdk/client-redshift-data
The AWS SDK is modulized by clients and commands.
To send a request, you only need to import the RedshiftDataClient
and
the commands you need, for example ListSchemasCommand
:
// ES5 example
const { RedshiftDataClient, ListSchemasCommand } = require("@aws-sdk/client-redshift-data");
// ES6+ example
import { RedshiftDataClient, ListSchemasCommand } from "@aws-sdk/client-redshift-data";
To send a request, you:
send
operation on client with command object as input.destroy()
to close open connections.// a client can be shared by different commands.
const client = new RedshiftDataClient({ region: "REGION" });
const params = {
/** input parameters */
};
const command = new ListSchemasCommand(params);
We recommend using await operator to wait for the promise returned by send operation as follows:
// async/await.
try {
const data = await client.send(command);
// process data.
} catch (error) {
// error handling.
} finally {
// finally.
}
Async-await is clean, concise, intuitive, easy to debug and has better error handling as compared to using Promise chains or callbacks.
You can also use Promise chaining to execute send operation.
client.send(command).then(
(data) => {
// process data.
},
(error) => {
// error handling.
}
);
Promises can also be called using .catch()
and .finally()
as follows:
client
.send(command)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
})
.finally(() => {
// finally.
});
We do not recommend using callbacks because of callback hell, but they are supported by the send operation.
// callbacks.
client.send(command, (err, data) => {
// process err and data.
});
The client can also send requests using v2 compatible style. However, it results in a bigger bundle size and may be dropped in next major version. More details in the blog post on modular packages in AWS SDK for JavaScript
import * as AWS from "@aws-sdk/client-redshift-data";
const client = new AWS.RedshiftData({ region: "REGION" });
// async/await.
try {
const data = await client.listSchemas(params);
// process data.
} catch (error) {
// error handling.
}
// Promises.
client
.listSchemas(params)
.then((data) => {
// process data.
})
.catch((error) => {
// error handling.
});
// callbacks.
client.listSchemas(params, (err, data) => {
// process err and data.
});
When the service returns an exception, the error will include the exception information, as well as response metadata (e.g. request id).
try {
const data = await client.send(command);
// process data.
} catch (error) {
const { requestId, cfId, extendedRequestId } = error.$metadata;
console.log({ requestId, cfId, extendedRequestId });
/**
* The keys within exceptions are also parsed.
* You can access them by specifying exception names:
* if (error.name === 'SomeServiceException') {
* const value = error.specialKeyInException;
* }
*/
}
Please use these community resources for getting help. We use the GitHub issues for tracking bugs and feature requests, but have limited bandwidth to address them.
aws-sdk-js
on AWS Developer Blog.aws-sdk-js
.To test your universal JavaScript code in Node.js, browser and react-native environments, visit our code samples repo.
This client code is generated automatically. Any modifications will be overwritten the next time the @aws-sdk/client-redshift-data
package is updated.
To contribute to client you can check our generate clients scripts.
This SDK is distributed under the Apache License, Version 2.0, see LICENSE for more information.
3.709.0 (2024-12-10)
FAQs
AWS SDK for JavaScript Redshift Data Client for Node.js, Browser and React Native
The npm package @aws-sdk/client-redshift-data receives a total of 107,663 weekly downloads. As such, @aws-sdk/client-redshift-data popularity was classified as popular.
We found that @aws-sdk/client-redshift-data demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.