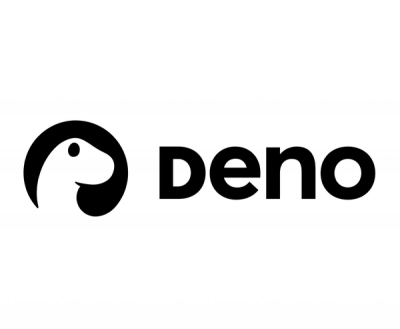
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@aws-sdk/config-resolver
Advanced tools
[](https://www.npmjs.com/package/@aws-sdk/config-resolver) [](https://www.npmjs.com/package/@aws-sd
@aws-sdk/config-resolver is a package within the AWS SDK for JavaScript that provides utilities for resolving configuration settings for AWS clients. It helps in managing and resolving configurations such as region, credentials, and other client-specific settings.
Region Resolution
This feature allows you to resolve the region configuration for an AWS client. The code sample demonstrates how to use the `resolveRegionConfig` function to resolve the region setting from a client configuration object.
const { resolveRegionConfig } = require('@aws-sdk/config-resolver');
const clientConfig = { region: 'us-west-2' };
const resolvedConfig = resolveRegionConfig(clientConfig);
console.log(resolvedConfig);
Credentials Resolution
This feature allows you to resolve the credentials configuration for an AWS client. The code sample demonstrates how to use the `resolveCredentialsConfig` function to resolve the credentials setting from a client configuration object.
const { resolveCredentialsConfig } = require('@aws-sdk/config-resolver');
const clientConfig = { credentials: { accessKeyId: 'AKIA...', secretAccessKey: 'SECRET...' } };
const resolvedConfig = resolveCredentialsConfig(clientConfig);
console.log(resolvedConfig);
Endpoint Resolution
This feature allows you to resolve the endpoint configuration for an AWS client. The code sample demonstrates how to use the `resolveEndpointConfig` function to resolve the endpoint setting from a client configuration object.
const { resolveEndpointConfig } = require('@aws-sdk/config-resolver');
const clientConfig = { endpoint: 'https://dynamodb.us-west-2.amazonaws.com' };
const resolvedConfig = resolveEndpointConfig(clientConfig);
console.log(resolvedConfig);
The `aws-sdk` package is the official AWS SDK for JavaScript. It provides a comprehensive set of tools for interacting with AWS services, including configuration management. Unlike `@aws-sdk/config-resolver`, which is a modular package focused on configuration resolution, `aws-sdk` is a monolithic package that includes all AWS service clients and utilities.
The `aws-sdk-mock` package is used for mocking AWS SDK calls in unit tests. While it does not directly compete with `@aws-sdk/config-resolver`, it provides functionalities for testing AWS SDK configurations and responses, which can be useful in a development environment where configuration resolution needs to be tested.
The `aws-config` package is a lightweight utility for loading AWS configuration settings from various sources such as environment variables, JSON files, and command-line arguments. It offers similar configuration resolution capabilities but is more focused on loading configurations from different sources rather than resolving them for AWS clients.
3.18.0 (2021-06-04)
FAQs
[](https://www.npmjs.com/package/@aws-sdk/config-resolver) [](https://www.npmjs.com/package/@aws-sd
The npm package @aws-sdk/config-resolver receives a total of 2,684,557 weekly downloads. As such, @aws-sdk/config-resolver popularity was classified as popular.
We found that @aws-sdk/config-resolver demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.