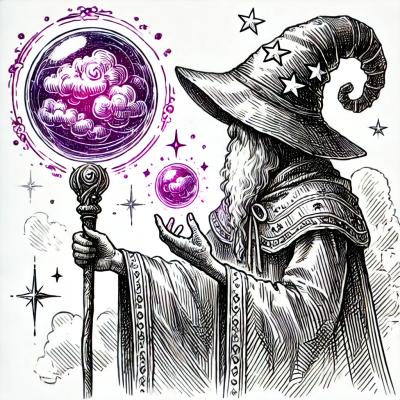
Security News
Cloudflare Adds Security.txt Setup Wizard
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
@aws-sdk/credential-provider-sso
Advanced tools
AWS credential provider that exchanges a resolved SSO login token file for temporary AWS credentials
The @aws-sdk/credential-provider-sso package is designed to facilitate the retrieval of AWS credentials using AWS Single Sign-On (SSO). This is particularly useful for developers and applications that need to authenticate against AWS services using SSO. The package simplifies the process of obtaining temporary credentials for AWS SDK clients.
Obtaining AWS credentials from SSO
This feature allows developers to obtain AWS credentials by specifying the SSO start URL, account ID, region, and role name. The credentials can then be used to authenticate AWS SDK clients.
const { fromSSO } = require('@aws-sdk/credential-provider-sso');
const client = new SomeAwsClient({
credentials: fromSSO({
ssoStartUrl: 'https://my-sso-start-url.example.com',
ssoAccountId: '123456789012',
ssoRegion: 'us-west-2',
ssoRoleName: 'MyRoleName'
})
});
The aws-sdk package is the official AWS SDK for JavaScript. While it supports various methods of authentication, including credentials from environment variables, shared credentials file, and IAM roles for EC2 instances, it does not specifically focus on SSO credential retrieval like @aws-sdk/credential-provider-sso. However, it provides a broader scope of AWS service interactions.
AWS Amplify is a development platform for building secure, scalable mobile and web applications. It includes support for authentication via Amazon Cognito, which can be integrated with SSO, but it's more focused on application development rather than directly providing AWS credentials for SDK clients. Compared to @aws-sdk/credential-provider-sso, AWS Amplify offers a higher-level abstraction for authentication and authorization.
This module provides a function, fromSSO
that will create CredentialProvider
functions that read from AWS SDKs and Tools shared configuration and credentials files(Profile appears
in the credentials file will be given precedence over the profile found in the
config file). This provider will load the resolved access token on local disk,
and then request temporary AWS credentials. For the guidance over AWS Single
Sign-On service, please refer to the service document.
You may customize how credentials are resolved by providing an options hash to
the fromSSO
factory function. The following options are supported:
profile
- The configuration profile to use. If not specified, the provider
will use the value in the AWS_PROFILE
environment variable or default
by
default.filepath
- The path to the shared credentials file. If not specified, the
provider will use the value in the AWS_SHARED_CREDENTIALS_FILE
environment
variable or ~/.aws/credentials
by default.configFilepath
- The path to the shared config file. If not specified, the
provider will use the value in the AWS_CONFIG_FILE
environment variable or
~/.aws/config
by default.ssoClient
- The SSO Client that used to request AWS credentials with the SSO
access token. If not specified, a default SSO client will be created with the
region specified in the profile sso_region
entry.This credential provider relies on AWS CLI to login to an AWS SSO session. Here's a brief walk-through:
$ aws configure sso
...
...
CLI profile name [123456789011_ReadOnly]: my-sso-profile<ENTER>
import { fromSSO } from "@aws-sdk/credential-provider-sso"; // ES6 example
// const { fromSSO } = require(@aws-sdk/credential-provider-sso") // CommonJS example
//...
const client = new FooClient({ credentials: fromSSO({ profile: "my-sso-profile" });
Alternatively, the SSO credential provider is supported in default Node.js credential provider:
import { defaultProvider } from "@aws-sdk/credential-provider-node"; // ES6 example
// const { defaultProvider } = require(@aws-sdk/credential-provider-node") // CommonJS example
//...
const client = new FooClient({ credentials: defaultProvider({ profile: "my-sso-profile" });
$ aws sso logout
Successfully signed out of all SSO profiles.
This credential provider is only applicable if the profile specified in shared configuration and credentials files contain ALL of the following entries:
~/.aws/credentials
[sample-profile]
sso_account_id = 012345678901
sso_region = us-east-1
sso_role_name = SampleRole
sso_start_url = https://d-abc123.awsapps.com/start
~/.aws/config
[profile sample-profile]
sso_account_id = 012345678901
sso_region = us-east-1
sso_role_name = SampleRole
sso_start_url = https://d-abc123.awsapps.com/start
3.18.0 (2021-06-04)
FAQs
AWS credential provider that exchanges a resolved SSO login token file for temporary AWS credentials
The npm package @aws-sdk/credential-provider-sso receives a total of 19,315,405 weekly downloads. As such, @aws-sdk/credential-provider-sso popularity was classified as popular.
We found that @aws-sdk/credential-provider-sso demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Cloudflare has launched a setup wizard allowing users to easily create and manage a security.txt file for vulnerability disclosure on their websites.
Security News
The Socket Research team breaks down a malicious npm package targeting the legitimate DOMPurify library. It uses obfuscated code to hide that it is exfiltrating browser and crypto wallet data.
Security News
ENISA’s 2024 report highlights the EU’s top cybersecurity threats, including rising DDoS attacks, ransomware, supply chain vulnerabilities, and weaponized AI.