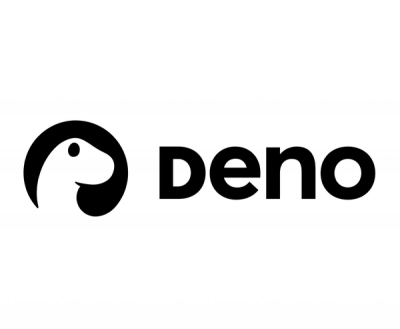
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@aws-sdk/util-hex-encoding
Advanced tools
Converts binary buffers to and from lowercase hexadecimal encoding
@aws-sdk/util-hex-encoding is a utility package from AWS SDK for JavaScript that provides functions to encode and decode hexadecimal strings. This can be particularly useful for handling binary data in a readable format.
Hex Encoding
This feature allows you to convert a Buffer or Uint8Array to a hexadecimal string. The code sample demonstrates encoding the string 'Hello, World!' into its hexadecimal representation.
const { toHex } = require('@aws-sdk/util-hex-encoding');
const buffer = Buffer.from('Hello, World!');
const hexString = toHex(buffer);
console.log(hexString); // Outputs: 48656c6c6f2c20576f726c6421
Hex Decoding
This feature allows you to convert a hexadecimal string back into a Buffer. The code sample demonstrates decoding the hexadecimal string back into the original 'Hello, World!' string.
const { fromHex } = require('@aws-sdk/util-hex-encoding');
const hexString = '48656c6c6f2c20576f726c6421';
const buffer = fromHex(hexString);
console.log(buffer.toString()); // Outputs: Hello, World!
The 'hex' package provides simple functions to encode and decode hexadecimal strings, similar to @aws-sdk/util-hex-encoding. It is lightweight and easy to use but does not offer the additional AWS SDK integrations.
The 'crypto-js' package offers a wide range of cryptographic functions, including hex encoding and decoding. It is more feature-rich compared to @aws-sdk/util-hex-encoding, providing additional cryptographic utilities like hashing and encryption.
The 'buffer' package is a Node.js core module that provides a way to handle binary data. It includes methods for hex encoding and decoding, similar to @aws-sdk/util-hex-encoding, but also offers a broader range of functionalities for binary data manipulation.
FAQs
Converts binary buffers to and from lowercase hexadecimal encoding
The npm package @aws-sdk/util-hex-encoding receives a total of 3,395,069 weekly downloads. As such, @aws-sdk/util-hex-encoding popularity was classified as popular.
We found that @aws-sdk/util-hex-encoding demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 5 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.