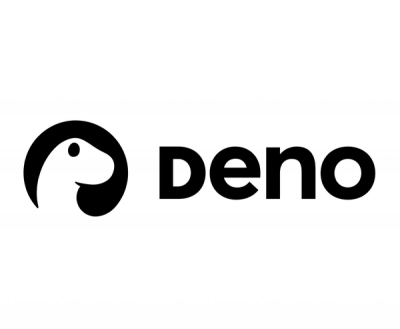
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@ayana/bento
Advanced tools
Bento is a robust NodeJS application framework designed to make creating and maintaing complex projects a simple and fast process.
Bento indroduces a concept of components. Components are logical chunks of code that all work together to provide your application to the world.
All components recieve their own ComponentAPI instance. The Component API is consistent across all components and provides a way for components to speak to eachother. As well as many other "Quality of life" features. Such as: variable injection and management, dependency management and injection, component event subscriptions, and more!
As a rule of thumb, components should not take on more then required. (IE: instead of having one component for connecting to Discord and processing messages. Have two, one for the connection and emitting the message events, and one that handles messages)
Here is a very basic example of a Bento component:
import { Component, ComponentAPI } from '@ayana/bento';
export class ExampleComponent {
// this property becomes available after onLoad see ComponentAPI for more info
public api: ComponentAPI;
// required for all components, must be unique
public name: string = 'ExampleComponent';
// Optionally define other components we depend upon
// Some decorators auto append to this array such as @SubscribeEvent
public dependencies: Array<Component> = [];
// Lifecycle event, called right before component fully loaded
public async onLoad() {
console.log('Hello world!');
}
// Lifecycle event, called right before component is unloaded
public async onUnload() {
console.log('Goodbye world!');
}
}
A runnable version of this example is available on Gitlab
Using Bento is pretty simple. First import and initilize Bento and any plugins you wish to use. Then simply add the plugins to Bento. The below example assumes you have a directory called "components" in the same directory (relative) to it.
import { Bento, FSComponentLoader } from '@ayana/bento';
// Create a Bento instance
const bento = new Bento();
// Anonymous async function so we can use await
(async () => {
// Create FSComponentLoader
// NOTE: Keep in mind all FSComponentLoader does is find components in a path
// Instantiates them and calls Bento.addComponent
// Behind the scenes
const fsloader = new FSComponentLoader();
await fsloader.addDirectory(__dirname, 'components');
// Apply plugin to Bento.
await bento.addPlugin(fsloader);
// Verify that Application looks good to continue
await bento.verify();
})().catch(e => {
console.error(`Error while starting Bento:\n${e}`);
process.exit(1);
});
More examples available here
FAQs
Modular runtime framework designed to solve complex tasks
The npm package @ayana/bento receives a total of 53 weekly downloads. As such, @ayana/bento popularity was classified as not popular.
We found that @ayana/bento demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.