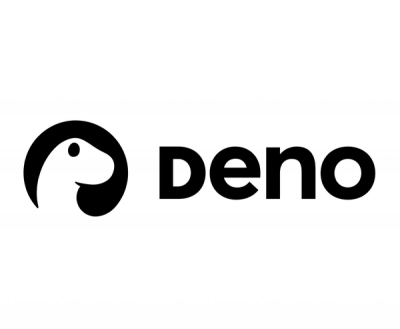
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@babel/helper-builder-binary-assignment-operator-visitor
Advanced tools
Helper function to build binary assignment operator visitors
@babel/helper-builder-binary-assignment-operator-visitor is a Babel helper module that assists in transforming binary assignment operators (like +=, -=, etc.) in JavaScript code. It provides utilities to create visitors for these operators, making it easier to handle and transform them during the Babel plugin development process.
Transforming Binary Assignment Operators
This feature allows you to transform binary assignment operators like += into their expanded form. The code sample demonstrates how to create a visitor that transforms the += operator into a binary expression using the @babel/helper-builder-binary-assignment-operator-visitor package.
const { types: t } = require('@babel/core');
const build = require('@babel/helper-builder-binary-assignment-operator-visitor');
const visitor = build({
operator: "+=",
build: (left, right) => t.binaryExpression(
"+",
left,
right
)
});
const plugin = {
visitor: {
AssignmentExpression(path) {
if (path.node.operator === "+=") {
visitor(path);
}
}
}
};
TODO
FAQs
Helper function to build binary assignment operator visitors
The npm package @babel/helper-builder-binary-assignment-operator-visitor receives a total of 12,502,707 weekly downloads. As such, @babel/helper-builder-binary-assignment-operator-visitor popularity was classified as popular.
We found that @babel/helper-builder-binary-assignment-operator-visitor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.