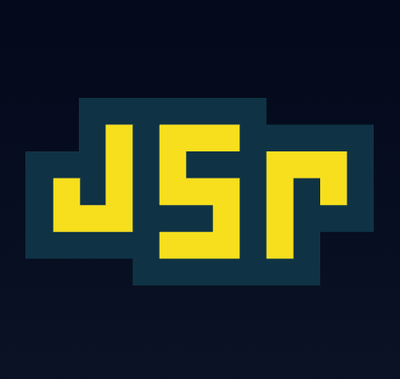
Security News
JSR Working Group Kicks Off with Ambitious Roadmap and Plans for Open Governance
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
@babel/plugin-proposal-decorators
Advanced tools
The @babel/plugin-proposal-decorators package is a Babel plugin that enables the use of decorators, which are a stage 2 proposal for JavaScript. Decorators provide a way to add annotations and a meta-programming syntax for class declarations and members. Decorators can be used to modify classes, properties, methods, and accessors.
Class Decorators
Class decorators are applied to the class and can be used to observe, modify, or replace a class definition. They can be used for logging, auto-binding, injecting dependencies, etc.
@decorator
class MyClass {}
function decorator(Class) {
// Modify or wrap the class
return modifiedClass;
}
Method Decorators
Method decorators are applied to methods of a class and can be used to observe, modify, or replace method definitions. This can be useful for debouncing, throttling, memoization, etc.
class MyClass {
@decorator
myMethod() {}
}
function decorator(target, name, descriptor) {
// Modify or wrap the method
return modifiedDescriptor;
}
Property Decorators
Property decorators are applied to class properties and can be used to modify the behavior of a property, such as by adding getters and setters, or by initializing with a default value.
class MyClass {
@decorator
myProperty;
}
function decorator(target, name) {
// Modify or define property behavior
}
Accessor Decorators
Accessor decorators are applied to getters and setters and can be used to observe, modify, or replace accessors. This can be useful for validation, logging, etc.
class MyClass {
_myValue;
@decorator
get myValue() {
return this._myValue;
}
set myValue(value) {
this._myValue = value;
}
}
function decorator(target, name, descriptor) {
// Modify or wrap the accessor
return modifiedDescriptor;
}
core-decorators is a library of decorators inspired by languages that include them from the start. It offers decorators like @readonly, @debounce, and @autobind, among others. Unlike @babel/plugin-proposal-decorators, which is a Babel plugin to transpile decorator syntax, core-decorators provides ready-to-use decorators for existing JavaScript code.
TypeScript is a superset of JavaScript that adds static types and has built-in support for decorators. TypeScript decorators are similar in concept to those in @babel/plugin-proposal-decorators, but they are built into the language and can be used without a separate Babel plugin. TypeScript decorators can be used for class, method, property, and accessor decoration, similar to @babel/plugin-proposal-decorators.
mobx is a state management library that makes use of decorators for observable properties, computed values, and reactions. The decorators provided by mobx, such as @observable and @computed, are specific to the library's reactivity system and are used to enhance the reactive programming experience. While @babel/plugin-proposal-decorators enables the decorator syntax, mobx provides specific decorators for its own ecosystem.
Compile class and object decorators to ES5
See our website @babel/plugin-proposal-decorators for more information.
Using npm:
npm install --save-dev @babel/plugin-proposal-decorators
or using yarn:
yarn add @babel/plugin-proposal-decorators --dev
v7.15.8 (2021-10-06)
babel-helper-module-transforms
, babel-plugin-transform-modules-amd
, babel-plugin-transform-modules-commonjs
, babel-plugin-transform-modules-umd
babel-parser
babel-generator
babel-generator
, babel-parser
, babel-plugin-proposal-pipeline-operator
babel-plugin-transform-typescript
babel-core
babel-node
FAQs
Compile class and object decorators to ES5
The npm package @babel/plugin-proposal-decorators receives a total of 8,560,663 weekly downloads. As such, @babel/plugin-proposal-decorators popularity was classified as popular.
We found that @babel/plugin-proposal-decorators demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
At its inaugural meeting, the JSR Working Group outlined plans for an open governance model and a roadmap to enhance JavaScript package management.
Security News
Research
An advanced npm supply chain attack is leveraging Ethereum smart contracts for decentralized, persistent malware control, evading traditional defenses.
Security News
Research
Attackers are impersonating Sindre Sorhus on npm with a fake 'chalk-node' package containing a malicious backdoor to compromise developers' projects.