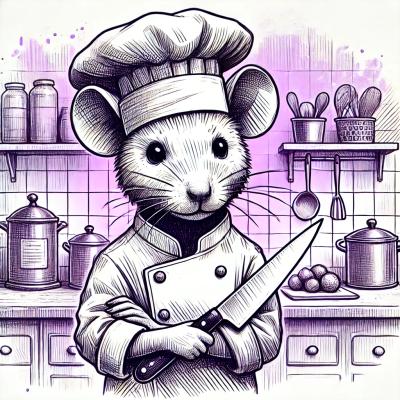
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@backstage/backend-common
Advanced tools
Common functionality library for Backstage backends
@backstage/backend-common is a utility library for building backend services in the Backstage ecosystem. It provides common functionalities such as logging, database connections, and server setup, which are essential for developing robust and scalable backend services.
Logging
Provides a root logger that can be used throughout the application for consistent logging.
const { getRootLogger } = require('@backstage/backend-common');
const logger = getRootLogger();
logger.info('This is an info message');
Database Connection
Helps in setting up and managing database connections using a configuration object.
const { DatabaseManager } = require('@backstage/backend-common');
const config = { /* database configuration */ };
const databaseManager = DatabaseManager.fromConfig(config);
const db = databaseManager.forPlugin('my-plugin');
Server Setup
Simplifies the process of setting up a web server with routing and middleware.
const { createServiceBuilder } = require('@backstage/backend-common');
const service = createServiceBuilder(module)
.setPort(7000)
.addRouter('/api', myApiRouter)
.start();
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for web and mobile applications. Unlike @backstage/backend-common, which is tailored for the Backstage ecosystem, Express is a general-purpose web framework.
Winston is a versatile logging library for Node.js. While @backstage/backend-common includes logging as one of its features, Winston is a dedicated logging library with more advanced features and customization options.
Knex is a SQL query builder for Node.js, designed to be flexible and portable. It provides a similar database management functionality as @backstage/backend-common but is more focused on query building and database migrations.
[!CAUTION] This package is deprecated and will be removed in a near future, so please follow the deprecated instructions for the exports you still use.
Common functionality library for Backstage backends, implementing logging, error handling and similar.
Add the library to your backend package:
# From your Backstage root directory
yarn --cwd packages/backend add @backstage/backend-common
then make use of the handlers and logger as necessary:
import {
errorHandler,
getRootLogger,
notFoundHandler,
requestLoggingHandler,
} from '@backstage/backend-common';
const app = express();
app.use(requestLoggingHandler());
app.use('/home', myHomeRouter);
app.use(notFoundHandler());
app.use(errorHandler());
app.listen(PORT, () => {
getRootLogger().info(`Listening on port ${PORT}`);
});
FAQs
Common functionality library for Backstage backends
We found that @backstage/backend-common demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.