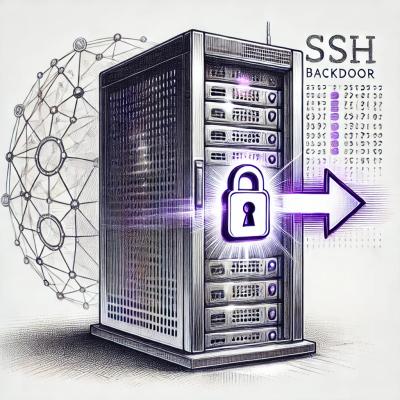
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@baigiel/editor
Advanced tools
A fully-featured rich text editor built with React and Tiptap. This library provides customizable components and extensions to create a seamless and interactive text editing experience.
Ensure you have Node.js installed.
Clone the Repository:
git clone https://github.com/yourusername/richtext-editor-library.git
cd richtext-editor-library
Install Dependencies:
npm install
# or
yarn install
Start the Development Server:
npm start
# or
yarn start
Import the Editor
component and include it in your React application.
import React, { useState } from 'react';
import Editor from './components/Editor';
const App = () => {
const [content, setContent] = useState({});
const handleChange = (doc, raw) => {
setContent(doc);
console.log(raw);
};
return (
<div className="container">
<Editor value={content} onChange={handleChange} />
</div>
);
};
export default App;
The main editor component allowing full editing capabilities.
import Editor from './components/Editor';
<Editor
id="main-editor"
value={initialContent}
onChange={handleChange}
closeOnBlur={true}
onBlur={handleBlur}
/>
A non-editable version of the editor for displaying content.
import ReadonlyEditor from './components/ReadonlyEditor';
<ReadonlyEditor
id="readonly-editor"
value={content}
readonly={true}
/>
Combines both editable and readonly states based on focus.
import RichEditor from './components/RichEditor';
<RichEditor
id="rich-editor"
value={content}
readonly={false}
closeOnBlur={true}
onChange={handleChange}
/>
A simple preview component showcasing the editor.
import Preview from './demo/Preview';
<Preview />
Provides a toolbar with various editing options.
import EditorMenu from './components/EditorMenu';
<EditorMenu editor={editorInstance} />
Reusable button component for editor actions.
import EditorButton from './components/EditorButton';
<EditorButton
onClick={handleClick}
leadingIcon={<FiBold />}
>
Bold
</EditorButton>
Actions for saving or canceling edits.
import EditorActions from './components/EditorActions';
<EditorActions
handleSave={saveContent}
handleBlur={cancelEdit}
/>
Enhance table functionalities with custom behaviors.
import Table from '@tiptap/extension-table';
import TableRow from '@tiptap/extension-table-row';
import TableCell from '@tiptap/extension-table-cell';
import Columns from './extensions/Columns';
import Column from './extensions/Column';
const editor = useEditor({
extensions: [
Table.configure({ ... }),
TableRow.configure({ ... }),
TableCell.configure({ ... }),
Columns,
Column,
// other extensions
],
});
Manage column layouts within the editor.
import Columns, { ColumnLayout } from './extensions/Columns';
editor.chain().focus().setColumns().run();
editor.chain().focus().setLayout(ColumnLayout.TwoColumn).run();
import Underline from '@tiptap/extension-underline';
import Link from '@tiptap/extension-link';
import Paragraph from '@tiptap/extension-paragraph';
const editor = useEditor({
extensions: [
Underline,
Link.configure({ ... }),
Paragraph,
// other extensions
],
});
Customize the editor's appearance and behavior using Tailwind CSS and by extending Tiptap's capabilities.
Utilize Tailwind CSS classes to style the editor components.
/* src/index.css */
@tailwind base;
@tailwind components;
@tailwind utilities;
/* Custom styles */
.editor-container {
@apply p-4 bg-white rounded-md shadow-md;
}
Add or modify editor functionalities by creating custom extensions.
// src/extensions/CustomExtension.ts
import { Extension } from '@tiptap/core';
const CustomExtension = Extension.create({
name: 'customExtension',
// Define extension behavior
});
export default CustomExtension;
cn
A utility function to conditionally join classNames using clsx and tailwind-merge.
import { cn } from './utils/cn';
<div className={cn('base-class', condition && 'conditional-class')} />
// src/utils/cn.ts
import { type ClassValue, clsx } from "clsx";
import { twMerge } from "tailwind-merge";
export function cn(...inputs: ClassValue[]) {
return twMerge(clsx(inputs));
}
useClickOutside
Detects clicks outside a specified element to trigger callbacks, useful for closing menus or dialogs.
import { useClickOutside } from './hooks/useClickOutside';
const ref = useRef<HTMLDivElement>(null);
useClickOutside(ref, () => setIsOpen(false));
// src/hooks/useClickOutside.ts
import { useEffect, RefObject } from "react";
export const useClickOutside = <T extends HTMLElement>(
ref: RefObject<T>,
callback: () => void
) => {
useEffect(() => {
const handleClickOutside = (event: MouseEvent) => {
if (ref.current && !ref.current.contains(event.target as Node)) {
callback();
}
};
document.addEventListener("mousedown", handleClickOutside);
return () => {
document.removeEventListener("mousedown", handleClickOutside);
};
}, []);
};
Contributions are welcome! Please follow these steps:
Fork the Repository
Create a Feature Branch
git checkout -b feature/YourFeature
Commit Your Changes
git commit -m "Add some feature"
Push to the Branch
git push origin feature/YourFeature
Open a Pull Request
Please ensure your code follows the project's coding standards and includes relevant tests.
Feel free to customize this README to better fit the specifics of your library and project structure.
FAQs
Baigiel rich text editor
The npm package @baigiel/editor receives a total of 545 weekly downloads. As such, @baigiel/editor popularity was classified as not popular.
We found that @baigiel/editor demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.