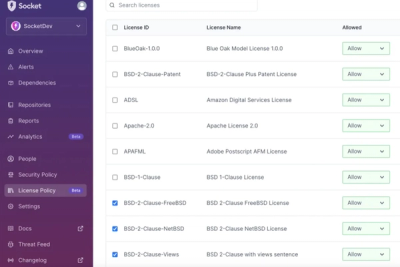
Product
Introducing License Enforcement in Socket
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
@balmy/sdk
Advanced tools
This repository contains the code for the Balmy sdk.
yarn add @balmy/sdk
npm install @balmy/sdk
import { buildSdk } from "@balmy/sdk";
const sdk = buildSdk(config);
const accountBalances = await sdk.balanceService.getBalancesForTokens({
account: "0x000000000000000000000000000000000000dead",
tokens: {
// [chainId]: [0xTokenAddress]
[Chains.ETHEREUM.chainId]: [
// Ethereum
"0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48", // USDC
"0x6b175474e89094c44da98b954eedeac495271d0f", // DAI
],
[Chains.OPTIMISM.chainId]: [
// Optimism
"0x7f5c764cbc14f9669b88837ca1490cca17c31607", // USDC
"0xda10009cbd5d07dd0cecc66161fc93d7c9000da1", // DAI
],
},
// Optional config
config: {
timeout: "30s",
},
});
const usdcBalanceOnEthereum =
accountBalances[Chains.ETHEREUM.chainId][
"0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48"
];
const accountAllowances = await sdk.allowanceService.getAllowances({
chainId: Chains.ETHEREUM.chainId,
token: "0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48", // USDC
owner: "0x000000000000000000000000000000000000dead",
spenders: ["0x6666666600000000000000000000000000009999"],
});
const amountThatDevilCanSpend =
accountAllowances["0x6666666600000000000000000000000000009999"];
const allQuotes = await sdk.quoteService.getAllQuotesWithTxs({
request: {
chainId: Chains.ETHEREUM.chainId, // Ethereum
sellToken: "0xa0b86991c6218b36c1d19d4a2e9eb0ce3606eb48",
buyToken: "0xc02aaa39b223fe8d0a0e5c4f27ead9083c756cc2",
order: {
type: "sell",
sellAmount: utils.parseUnits("1000", 6), // 1000 USDC
},
slippagePercentage: 1, // 1%
takerAddress: signer.address,
// Optional gas speed
gasSpeed: {
speed: "instant",
},
},
// Optional config
config: {
sort: {
by: "most-swapped-accounting-for-gas",
},
},
});
const bestTradeBySort = allQuotes[0];
await signer.sendTransaction(bestTradeBySort.tx);
yarn install
WIP - Will be at docs.balmy.xyz
FAQs
This repository contains the code for the Balmy sdk.
The npm package @balmy/sdk receives a total of 349 weekly downloads. As such, @balmy/sdk popularity was classified as not popular.
We found that @balmy/sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Ensure open-source compliance with Socket’s License Enforcement Beta. Set up your License Policy and secure your software!
Product
We're launching a new set of license analysis and compliance features for analyzing, managing, and complying with licenses across a range of supported languages and ecosystems.
Product
We're excited to introduce Socket Optimize, a powerful CLI command to secure open source dependencies with tested, optimized package overrides.