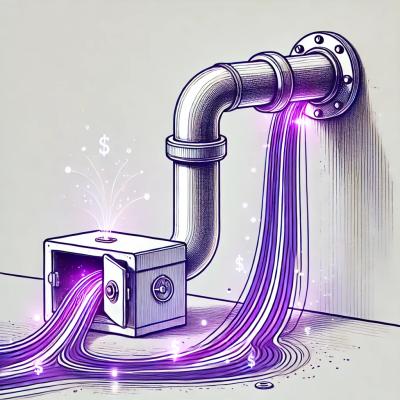
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
@benev/slate
Advanced tools
@benev/slate
🚧 prerelease wip under constructon subject to change
you're not supposed to make your whole app out of web components.
they're too cumbersome — web components are html-native, not typescript-native — so they don't take typesafe props, they're referred to by html tag names with poor ide support, and juggling their dom registrations is a pain.
this is why views are important, and are a central feature of slate — views are almost the same as components (they can use shadow-dom), except that views are ergonomically handled via javascript, they accept props, they don't need registration (they're simply imported) — and views enjoy full typescript typings.
you want to think of web components as the tip of your iceberg — they are the entrypoints to your ui — they are the universal control surfaces to help html authors interact with your systems — but below the surface, most of your internals can be made of easily-composable views.
npm i @benev/slate
import {Slate, Context} from "@benev/slate"
export const slate = new Slate(
new class extends Context {
// this theme is applied to all your components and views
theme = css`
* {
margin: 0;
padding: 0;
box-sizing: border-box;
}
`
// add anything app-level you'd like to make widely available
my_cool_thing = {my_awesome_data: 123}
}
)
import {html, css} from "@benev/slate"
you can create custom html elements that work in plain html or any web framework.
slate.shadow_component
— "carbon"const styles = css`span {color: yellow}`
export const MyCarbon = slate.shadow_component({styles}, use => {
const count = use.signal(0)
const increment = () => count.value++
return html`
<span>${count}</span>
<button @click=${increment}>increment</button>
`
})
slate.light_component
— "oxygen"export const MyOxygen = slate.light_component(use => {
const count = use.signal(0)
const increment = () => count.value++
return html`
<span>${count}</span>
<button @click=${increment}>increment</button>
`
})
import {register_to_dom} from "@benev/slate"
register_to_dom({
MyCarbon,
MyOxygen,
})
<section>
<my-carbon></my-carbon>
<my-oxygen></my-oxygen>
</section>
views are just like components, but are not registered to the dom as custom html elements.
instead, they are used via javascript.
you import them, and inject them into your lit-html templates.
they accept js parameters called props
, and are fully typescript-typed.
slate.shadow_view
— "obsidian"const styles = css`span {color: yellow}`
export const MyObsidian = slate.shadow_view({styles}, use => (start: number) => {
const count = use.signal(start)
const increment = () => count.value++
return html`
<span>${count}</span>
<button @click=${increment}>increment</button>
`
})
auto_exportparts
is enabled by default.
part
attribute, then it will automatically re-export all internal parts, using the part as a prefix.::part(search-input-icon)
.slate.light_view
— "quartz"export const MyQuartz = slate.light_view(use => (start: number) => {
const count = use.signal(start)
const increment = () => count.value++
return html`
<span>${count}</span>
<button @click=${increment}>increment</button>
`
})
html`<div>${MyQuartz(123)}</div>`
html`<div>${MyObsidian([123])}</div>`
<obsidian-view>
component, which is where the shadow root is attachedhtml`
<div>
${MyObsidian([123], {
content: html`<p>slotted content</p>`,
auto_exportparts: true,
attrs: {part: "cool", "data-whatever": true},
})}
</div>
`
use
hooks — for views and componentsconst [count, setCount] = use.state(0)
const increment = () => setCount(count + 1)
use.setup(() => {
const interval = setInterval(increment, 1000)
return () => clearInterval(interval)
})
const random_number = use.prepare(() => Math.random())
const scene = use.init(() => {
// called whenever dom is connected
const scene = setup_3d_scene_for_example()
return [
canvas, // value returned
() => scene.cleanup(), // cleanup called on dom disconnect
]
})
const count = use.signal(0)
const increment = () => count.value++
you can directly inject the whole signal into html
html`<span>${count}</span>`
const count = use.signal(2)
const tripled = use.computed(() => count.value * 3)
console.log(tripled.value) //> 6
const count = use.op()
count.run(async() => fetchCount("/count"))
const state = use.flatstate({count: 0})
const increment = () => state.count++
// access your own things on the context
use.context.my_cool_thing
use.element.querySelector("p")
use.shadow.querySelector("slot")
const attrs = use.attrs({
start: Number,
label: String,
["data-active"]: Boolean,
})
set them like normal js properties
attrs.start = 123
attrs.label = "hello"
attrs["data-active"] = true
get them like normal js properties
console.log(attrs.start) // 123
console.log(attrs.label) // "hello"
console.log(attrs["data-active"]) // true
components rerender when any attributes change from outsidegold and silver are "plain" elements, which are alternatives to LitElement.
they're used as primitives underlying our carbon and oxygen components.
for most cases you probably want to stick with carbon/oxygen, and only use gold/silver when you're doing some funky sorcery, or you yearn to go back to a simpler time, without hooks.
consider these imports for the following examples:
import {GoldElement, SilverElement, attributes, flat} from "@benev/slate"
export class MyGold extends GoldElement {
static styles = css`span {color: blue}`
#attrs = attributes(this as GoldElement, {
label: String
})
#state = flat.state({
count: 0,
})
render() {
return html`
<span>${this.#state.count}</span>
<button @click=${() => this.#state.count++}>
${this.#attrs.label}
</button>
`
}
}
export class MySilver extends SilverElement {
#attrs = attributes(this as SilverElement, {
label: String
})
#state = flat.state({
count: 0,
})
render() {
return html`
<span>${this.#state.count}</span>
<button @click=${() => this.#state.count++}>
${this.#attrs.label}
</button>
`
}
}
if you want plain elements to have reactivity or have the context's css theme applied, you'll want to run them through slate.components
before you register them:
register_to_dom({
...slate.components({
MyGold,
MySilver,
}),
})
you can extend the context with anything you'd like to make easily available:
export const slate = new Slate(
new class extends Context {
my_cool_thing = {my_awesome_data: 123}
}
)
but since your components are importing slate
, this context is being created at import-time.
you may instead prefer to defer the creation of your context until later, at run-time:
// define your context class
export class MyContext extends Context {
my_cool_thing = {my_awesome_data: 123}
}
// create slate *without yet* instancing the context
export slate = new Slate<MyContext>()
//
// ... later, maybe in your main.ts ...
//
// instance and assign your context, now, at runtime
slate.context = new MyContext()
// just be sure to assign context *before* you register your components
register_to_dom(myComponents)
if you're using slate's frontend components and views, you'll probably be using these utilities via the use
hooks, which will provide a better developer experience.
however, the following utilities are little libraries in their own right, and can be used in a standalone capacity.
signals are a simple form of state management.
this implementation is inspired by preact signals.
import {signal, signals} from "@benev/slate"
const count = signal(0)
const greeting = signal("hello")
count.value++
greeting.value = "bonjour"
console.log(count.value) //> 1
console.log(greeting.value) //> "bonjour"
signals.track(() => console.log("doubled", count.value * 2))
//> doubled 2
count.value = 2
//> doubled 4
html`<p>count is ${count}</p>`
const json = signals.op<MyJson>()
console.log(json.loading) //> true
await json.run(async() => {
const data = await fetch_remote_data()
return JSON.parse(data)
})
console.log(json.ready) //> true
console.log(json.payload) //> {"your": "json data"}
count.value = 1
const tripled = signals.computed(() => count.value * 3)
console.log(tripled.value) //> 3
const tripled = signals.computed(() => count.value * 3)
console.log(tripled.value) //> 3
count.value = 10
console.log(tripled.value) //> 3 (too soon!)
await signals.wait
console.log(tripled.value) //> 30 (there we go)
import {SignalTower} from "@benev/slate"
const signals = new SignalTower()
signals
, but you can create your ownflatstate help you create state objects and react when properties change.
flatstate is inspired by mobx and snapstate, but designed to be super simple: flatstate only works on flat state objects, only the direct properties of state objects are tracked for reactivity.
import {flat} from "@benev/slate"
const state = flat.state({count: 0})
flat.reaction(() => console.log(state.count))
//> 0
state.count++
//> 1
import {Flat} from "@benev/slate"
const flat = new Flat()
// what happens in this flat, stays in this flat.
// you should probably just use the
// default `flat` that comes with slate.
const flat = new Flat()
const state = flat.state({amount: 100})
state.amount = 101
console.log(state.amount) //> 100 (old value)
await flat.wait
console.log(state.amount) //> 101 (now it's ready)
const stop = flat.reaction(() => console.log(state.count))
stop() // end this particular reaction
// clear all reactions on this flat instance
flat.clear()
flat.reaction(() => console.log(state.count))
flat.reaction(
() => ({count: state.count}),
({count}) => console.log(count),
)
flat.deepReaction(() => console.log(state.count))
.auto
and .manual
reactions
discovery
and debounce
(you can turn off the debouncer)const flat1 = new Flat()
const flat2 = new Flat()
const state = flat.state({count: 0})
const rstate = Flat.readonly(state)
state.count = 1
await flat.wait
console.log(rstate.count) //> 1
rstate.count = 2 // !! ReadonlyError !!
import {apply} from "@benev/slate"
const MyElement2 = mixin.flat(flat)(MyElement)
// can also be a class decorator
const elements2 = apply.flat(flat)(elements)
utility for ui loading/error/ready states.
useful for implementing async operations that involve loading indicators.
you get a better dev-experience if you use ops via signals, but here is the documentation for plain ops on their own, without signals.
import {Op} from "@benev/slate"
Op.loading()
//= {mode: "loading"}
Op.error("a fail occurred")
//= {mode: "error", reason: "a fail occurred"}
Op.ready(123)
//= {mode: "ready", payload: 123}
let my_op = Op.loading()
await Op.run(op => my_op = op, async() => {
await nap(1000)
return 123
})
const count = use.op()
count.run(async() => {
await sleep(1000)
return 123
})
// type for op in any mode
// v
function example(op: Op.Any<number>) {
// branching based on the op's mode
Op.select(op, {
loading: () => console.log("op is loading"),
error: reason => console.log("op is error", reason),
ready: payload => console.log("op is ready", payload)
})
const payload = Op.payload(op)
// if the mode=ready, return the payload
// otherwise, return undefined
}
// bad
register_to_dom(
apply.signals(signals)(
apply.flat(flat)(
apply.css(theme)(
requirement.provide(context)(elements)
)
)
)
)
import {Pipe} from "@benev/slate"
// good
Pipe.with(elements)
.to(requirement.provide(context))
.to(apply.css(theme))
.to(apply.flat(flat))
.to(apply.signals(signals))
.to(register_to_dom)
.done()
when you want to return the resultno time to document these fully, but they're there
debounce
— is a pretty good debouncerdeep_equal
— compare jsondeep_freeze
— make stuff immutableexplode_promise
— get a promise flipped inside-outgenerate_id
— generate a crypto-random hexadecimal id stringpub
— easy pub/sub toolrequirement
— pass required data to a group of thingsFAQs
frontend web stuff
The npm package @benev/slate receives a total of 623 weekly downloads. As such, @benev/slate popularity was classified as not popular.
We found that @benev/slate demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.