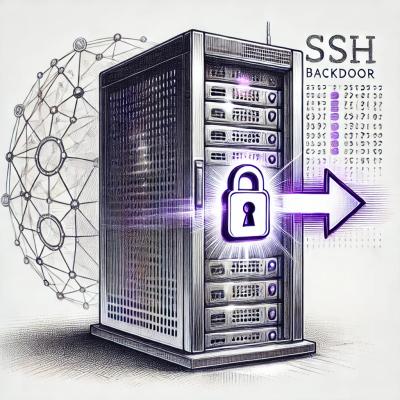
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
@bikeshaving/crank
Advanced tools
Crank is a JavaScript library for building user interfaces. This is an early beta.
function HelloMessage({name}) {
return <div>Hello {name}</div>;
}
function *Timer() {
let seconds = 0;
const interval = setInterval(() => {
seconds++;
this.refresh();
}, 1000);
try {
while (true) {
yield (<div>Seconds: {seconds}</div>);
}
} finally {
clearInterval(interval);
}
}
async function IPAddress() {
const res = await fetch("https://api.ipify.org");
const address = await res.text();
return <div>Your IP Address: {address}</div>;
}
async function Loading({message = "Loading…", wait = 1000}) {
await new Promise((resolve) => setTimeout(resolve, wait));
return message;
}
async function DogImage({throttle=false}) {
if (throttle) {
await new Promise((resolve) => setTimeout(resolve, 1000));
}
const res = await fetch("https://dog.ceo/api/breeds/image/random");
const data = await res.json();
return (
<figure>
<img src={data.message} height="400" />
<figcaption>
<a href={data.message}>{data.message}</a>
</figcaption>
</figure>
);
}
function RandomDogApp() {
this.addEventListener("click", (ev) => {
if (ev.target.className === "fetch") {
this.refresh();
}
});
return (
<div>
<div>
<button class="fetch">Fetch!</button>
</div>
<DogImage />
</div>
);
}
FAQs
Write JSX-driven components with functions, promises and generators.
The npm package @bikeshaving/crank receives a total of 179 weekly downloads. As such, @bikeshaving/crank popularity was classified as not popular.
We found that @bikeshaving/crank demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.