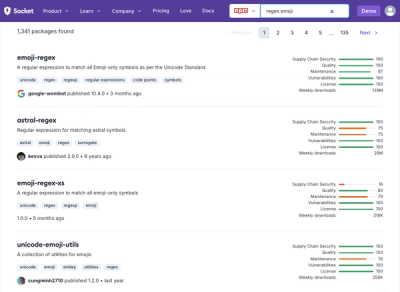
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
@bikeshaving/crank
Advanced tools
JSX-driven components with functions, promises and generators.
Crank is a new React-like library for building user interfaces. This is an early beta. A documentation website is coming soon as we dogfood the library.
Crank is available on NPM in the ESModule and CommonJS formats.
$ npm install @bikeshaving/crank
/* @jsx createElement */
import {createElement} from "@bikeshaving/crank";
import {renderer} from "@bikeshaving/crank/dom";
renderer.render(<div id="hello">Hello world</div>, document.body);
If your environment does not support ESModules (you’ll probably see a message like SyntaxError: Unexpected token export
), you can import the CommonJS versions of the crank like so:
/* @jsx createElement */
import {createElement} from "@bikeshaving/crank/cjs";
import {renderer} from "@bikeshaving/crank/cjs/dom";
renderer.render(<div id="hello">Hello world</div>, document.body);
function Greeting ({name}) {
return (
<div>Hello {name}</div>
);
}
function *Timer () {
let seconds = 0;
const interval = setInterval(() => {
seconds++;
this.refresh();
}, 1000);
try {
while (true) {
yield <div>Seconds: {seconds}</div>;
}
} finally {
clearInterval(interval);
}
}
async function IPAddress () {
const res = await fetch("https://api.ipify.org");
const address = await res.text();
return <div>Your IP Address: {address}</div>;
}
async function Fallback ({wait = 1000, children}) {
await new Promise((resolve) => setTimeout(resolve, wait));
return <Fragment>{children}</Fragment>;
}
async function *Suspense () {
for await (const {fallback, children} of this) {
yield <Fallback>{fallback}</Fallback>;
yield <Fragment>{children}</Fragment>;
}
}
async function RandomDog ({throttle=false}) {
if (throttle) {
await new Promise((resolve) => setTimeout(resolve, 2000));
}
const res = await fetch("https://dog.ceo/api/breeds/image/random");
const data = await res.json();
return (
<a href={data.message}>
<img src={data.message} width="250" />
</a>
);
}
function *RandomDogs () {
let throttle = false;
this.addEventListener("click", (ev) => {
if (ev.target.tagName === "BUTTON") {
throttle = !throttle;
this.refresh();
}
});
while (true) {
yield (
<Fragment>
<button>Show me another dog.</button>
<Suspense fallback={<div>Fetching a good boy…</div>}>
<RandomDog throttle={throttle} />
</Suspense>
</Fragment>
);
}
}
FAQs
Write JSX-driven components with functions, promises and generators.
The npm package @bikeshaving/crank receives a total of 179 weekly downloads. As such, @bikeshaving/crank popularity was classified as not popular.
We found that @bikeshaving/crank demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.