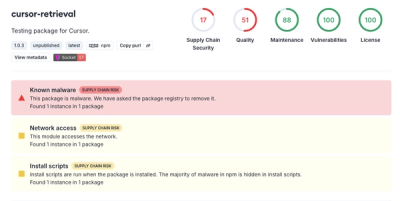
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@bloomreach/react-sdk
Advanced tools
Bloomreach React SDK provides simplified headless integration with Bloomreach Experience Manager for React-based applications. This library interacts with the Page Model API and Bloomreach SPA SDK and exposes a simplified declarative React interface over the Page Model.
Bloomreach Experience Manager (brXM) is an open and flexible CMS designed for developers and marketers. As the original headless CMS, brXM allows developers to build quickly and integrate with the systems. While it’s built for speed, it also provides top-notch personalization and channel management capabilities for marketers to drive results.
To get the SDK into your project with NPM:
npm install @bloomreach/react-sdk
And with Yarn:
yarn add @bloomreach/react-sdk
The following code snippet renders a simple page with a Banner component.
import React from 'react';
import axios from 'axios';
import { BrComponent, BrPage, BrPageContext, BrProps } from '@bloomreach/react-sdk';
function Banner({ component }: BrProps) {
return <div>Banner: {component.getName()}</div>;
}
export default function App() {
const config = { /* ... */ };
return (
<BrPage configuration={config} mapping={{ Banner }}>
<header>
<BrPageContext.Consumer>
{ page => <Link to={page.getUrl('/')} />Home</Link> }
</BrPageContext.Consumer>
<BrComponent path="menu"><Menu /></BrComponent>
</header>
<section>
<BrComponent path="main" />
</section>
<BrComponent path="footer">
<footer><BrComponent /></footer>
</BrComponent>
</BrPage>
);
}
The BrPage
component supports several options you may use to customize page initialization.
These options will be passed to the initialize
function from @bloomreach/spa-sdk
.
See here for the full configuration documentation.
The BrPage
component provides a way to link React components with the brXM ones.
It requires to pass the mapping
property that maps the component type with its representation.
The Container Items can be mapped by their labels.
import NewsList from './components/NewsList';
return <BrPage mapping={{ 'News List': NewsList }} />;
The Containers can be only mapped by their type, so you need to use constants from @bloomreach/spa-sdk
. By default, the React SDK provides an implementation for all the container types as it's defined in the documentation.
import { TYPE_CONTAINER_INLINE } from '@bloomreach/spa-sdk';
import MyInlineContainer from './components/MyInlineContainer';
return <BrPage mapping={{ [TYPE_CONTAINER_INLINE]: MyInlineContainer }} />;
From within the Container component, the Container Items can be accessed via the children
property.
This can be used to reorder or wrap child elements.
export default function MyInlineContainer() {
return (
<div>
{React.Children.map(props.children, child => (
<span className="float-left">
{child}
</span>
))}
</div>
);
}
The Components can be mapped by their names. It is useful for a menu component mapping.
import Menu from './components/Menu';
return <BrPage mapping={{ menu: Menu }} />;
There is also another way to render a component. In case you need to show a static component or a component from the abstract page, you can use inline component mapping.
return <BrComponent path="menu"><Menu /></BrComponent>
It is also possible to point where the component's children are going to be placed.
return (
<BrComponent path="footer">
<footer><BrComponent /></footer>
</BrComponent>
);
The component data in case of inline mapping can be accessed via the BrComponentContext
.
return (
<BrComponentContext.Consumer>
{component => (
<BrComponent path="footer">
<footer>
© {component.getName()}
<BrComponent />
</footer>
</BrComponent>
)}
</BrComponentContext.Consumer>
);
Or by using React Hooks.
import { BrComponentContext } from '@bloomreach/react-sdk';
export default function Menu() {
const component = React.useContext(BrComponentContext);
return <ul>{component.getName()}</ul>;
}
The React SDK is using Bloomreach SPA SDK to interact with the brXM. The complete reference of the exposed JavaScript objects can be found here.
This is the entry point to the page model.
This component requests and initializes the page model, and then renders the page root component with React children passed to this component.
The component also sets the page object into BrPageContext
.
Property | Required | Description |
---|---|---|
configuration | yes | The configuration of the SPA SDK. |
mapping | yes | The brXM and React components mapping. |
page | no | Preinitialized page instance or prefetched page model. Mostly that should be used to transfer state from the server-side to the client-side. |
This component points to where children or some component should be placed. BrComponent
can be used inside BrPage
or mapped components only. If React children are passed, then they will be rendered as-are. Otherwise, it will try to render all children components recursively.
Property | Required | Description |
---|---|---|
path | no | The path to a component. The path is defined as a slash-separated components name chain relative to the current component (e.g. main/container ). If it is omitted, all the children will be rendered. |
This component places a button on the page that opens the linked content in the document editor. The button will only be shown in preview mode.
Property | Required | Description |
---|---|---|
content | yes | The content entity to open for editing. |
This component places a button on the page that opens the linked menu in the menu editor. The button will only be shown in preview mode.
Property | Required | Description |
---|---|---|
menu | yes | The related menu model. |
The React Context holding the current brXM Component.
The React Context holding the current brXM Page.
Published under Apache 2.0 license.
FAQs
Bloomreach SPA SDK for React
The npm package @bloomreach/react-sdk receives a total of 3,632 weekly downloads. As such, @bloomreach/react-sdk popularity was classified as popular.
We found that @bloomreach/react-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.