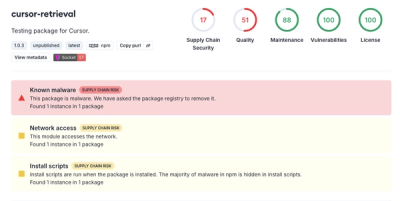
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
@bloomreach/spa-sdk
Advanced tools
Bloomreach SPA SDK provides simplified headless integration with Bloomreach Experience Manager for JavaScript-based applications. This library interacts with the Page Model API and exposes a simplified and framework-agnostic interface over the page model.
To get the SDK into your project with NPM:
npm install @bloomreach/spa-sdk
And with Yarn:
yarn add @bloomreach/spa-sdk
The following code snippet requests a related page model and shows the page's title.
import axios from "axios";
import { initialize } from "@bloomreach/spa-sdk";
async function showPage(path) {
const page = await initialize({
// The path to request from the Page Model API, should include query
// parameters if those are present in the url
path,
// The location of the Page Model API of the brX channel
endpoint: "http://localhost:8080/delivery/site/v1/channels/brxsaas/pages",
// The httpClient used to make requests
httpClient: axios,
});
document.querySelector("#title").innerText = page.getTitle();
}
showPage(`${window.location.pathname}${window.location.search}`);
(not applicable to Content SaaS)
The SDK provides basic Express middleware for seamless integration with the Relevance Module.
import express from "express";
import { relevance } from "@bloomreach/spa-sdk/lib/express";
const app = express();
app.use(relevance);
The middleware can be customized using the withOptions
method.
app.use(relevance.withOptions({ name: "_visitor", maxAge: 24 * 60 * 60 }));
The SPA SDK provides an API, Page.sanitize(html)
,
which sanitizes HTML content using the sanitize-html library,
to render the HTML content safely.
For example, in a React example, you may sanitize and render the HTML content which came from the backend like the following example:
{/* Suppose the content.value below contains HTML markups string. */}
<div>
{content && <div dangerouslySetInnerHTML={{ __html: page.rewriteLinks(page.sanitize(content.value)) }} />}
</div>
The same principle may apply in other frameworks. e.g, v-html
in Vue.js or [innerHTML]
in Angular.
Published under Apache 2.0 license.
The initialize
function supports several options you may use to customize page initialization.
Commonly used options |
---|
debug The option enabling debug mode. Required: no Default: false |
endpoint Base URL of the Page Model API (e.g. http://localhost:8080/delivery/site/v1/channels/brxsaas/pages or http://localhost:8080/site/channel/resourceapi ). This option is exclusive and should not be used together with options or cmsBaseUrl Required: yes Default: . none |
httpClient The HTTP client that will be used to fetch the page model. Signature is similar to Axios client. Required: yes Default: none |
path The path part of the URL, including a query string if present (e.g. /path/to/page?foo=1 Required: no Default: ) / . |
origin The brXM origin to verify an integration with the Experience Manager. This option should be used when the brXM is accessible from a host other than the Page Model API. By default, the origin from the apiBaseUrl or endpoint parameters is used. Required: no Default: none |
baseUrl Base URL of the SPA (e.g. /account or //www.example.com ). This option can only be used if endpoint is present. Required: no Default: "" |
apiBaseUrl Base URL of the Page Model API (e.g. http://localhost:8080/delivery/site/v1/channels/brxsaas/pages or http://localhost:8080/site/channel/resourceapi ). This option will be ignored if options is present. Required: no Default: cmsBaseUrl + "/resourceapi" |
spaBaseUrl Base URL of the SPA (e.g. /account or //www.example.com ). This option will be ignored if options is present. Required: no Default: "" |
Extra options |
---|
apiVersion Current API version. By default, the compatible with the current setup version will be chosen. Required: no Default: none |
apiVersionHeader API version header. Required: "Accept-Version" Default: none |
authorizationHeader Authorization header for the Page Model API. Required: no Default: "Authorization" |
authorizationQueryParameter The query string parameter used to pass authorization header value. Required: no Default: "token" |
authorizationToken Authorization token for the Page Model API. By default, the SDK will try to extract the token from the request query string using authorizationQueryParameter option. Required: no Default: none |
serverId Cluster node identifier. By default, the SDK will try to extract the value from the request query string using serverIdQueryParameter option. Required: no Default: none |
serverIdHeader Header identifying the current cluster node. Required: no Default: "Server-Id" |
serverIdQueryParameter The query string parameter used to pass a cluster node identifier. Required: no Default: "server-id" |
window A window object reference will be used to interact with brXM. It needs to be set when initialize is being called within an iframe or worker process. Required: no Default: window |
Relevance options (PaaS-only) |
---|
visitor An object holding information about the current visitor. The option takes precedence over request.visitor Required: no Default: . none |
visitor.id The current visitor identifier. Required: yes Default: none |
visitor.header An HTTP-header using to pass the visitor identifier to the Page Model API. Required: yes Default: none |
request Current user's request. Required: yes Default: none |
request.connection Current request remote connection containing the remote address. This option is used in the Relevance Module Required: no Default: . none |
request.emit Emits an event in the request scope. This option can be used in Node.js integration Required: no Default: . none |
request.headers An object holding request headers. It should contain a Cookie header if rendering is happening on the server-side in the UrlRewriter-based setup. Required: no Default: {} |
request.path The path part of the URL, including a query string if present (e.g. /path/to/page?foo=1 ). The option is deprecated in favor of path Required: no Default: . / |
request.visitor An object holding information about the current visitor. Required: no Default: none |
These options are deprecated and will be removed in the next major release |
---|
cmsBaseUrl Base URL of the site (e.g. http://localhost:8080/site or http://localhost:8080/site/channel ). This option is exclusive and should not be used together with options or endpoint Required: exclusive Default: . none |
options The CMS URL options. This option is exclusive and should not be used together with cmsBaseUrl or endpoint . Use this property to enable the UrlRewriter-based setup. Required: exclusive Default: none |
options.live The CMS URL options for the live site. Required: yes Default: none |
options.live.apiBaseUrl Base URL of the Page Model API for the live site (e.g. http://localhost:8080/delivery/site/v1/channels/brxsaas/pages or http://localhost:8080/site/channel/resourceapi Required: no Default: ) options.live.cmsBaseUrl + "/resourceapi" . |
options.live.cmsBaseUrl Base URL of the live site (e.g. http://localhost:8080/site or http://localhost:8080/site/channel Required: yes Default: ) none . |
options.live.spaBaseUrl Base URL of the live SPA (e.g. /account or //www.example.com Required: no Default: ) "" . |
options.preview The CMS URL options for the preview site. Required: yes Default: none |
options.preview.apiBaseUrl Base URL of the Page Model API for the preview site (e.g. http://localhost:8080/site/_cmsinternal/resourceapi or http://localhost:8080/site/_cmsinternal/channel/resourceapi Required: no Default: ) options .preview.cmsBaseUrl + "/resourceapi" . |
options.preview.cmsBaseUrl Base URL of the live site (e.g. http://localhost:8080/site/_cmsinternal or http://localhost:8080/site/_cmsinternal/channel Required: yes Default: ) none . |
options.preview.spaBaseUrl Base URL of the live SPA (e.g. /site/_cmsinternal?bloomreach-preview=true or /site/_cmsinternal/channel?bloomreach-preview=true ). This path and query string parameters will be used to detect whether it is a preview mode or not. Required: no Default: "" |
Function | Description |
---|---|
initialize(config, model?): Promise<Page> | This function accepts a configuration object as an argument and returns a promisified JavaScript object representing the page model. In case, when the page model has already been fetched, you can pass this JSON blob as a second parameter. |
destroy(page: Page): void | Destroys the integration with the SPA page object. |
getContainerItemContent<T>(component: ContainerItem, page: Page): T | null | Returns the content object that belongs to the container item, or null if not found. |
isPage(value): boolean | Checks whether a value is a page. |
isComponent(value): boolean | Checks whether a value is a page component. |
isContainer(value): boolean | Checks whether a value is a page container. |
isContainerItem(value): boolean | Checks whether a value is a page container item. |
isContent(value): boolean | Checks whether a value is a content object. |
isDocument(value): boolean | Checks whether a value is a document object. |
isImageSet(value): boolean | Checks whether a value is an image set object. |
isMenu(value): boolean | Checks whether a value is a menu object. |
isMeta(value): boolean | Checks whether a value is a meta-data object. |
isMetaComment(value): boolean | Checks whether a value is a meta-data comment. |
isPagination(value): boolean | Checks whether a value is a pagination. |
isLink(value): boolean | Checks whether a value is a link. |
isReference(value): boolean | Checks whether a value is a content reference. |
relevance(request: IncomingMessage, response: OutgoingMessage, next?: () => void): void | Express middleware for seamless integration with the Relevance Module. |
relevance.withOptions(options?: Options): Handler | Customizes Express middleware for the Relevance Module integration. |
Constant | Description |
---|---|
META_POSITION_BEGIN | Meta-data following before a page component. |
META_POSITION_END | Meta-data following after a page component. |
TYPE_CONTAINER_BOX | A blocked container with blocked items. |
TYPE_CONTAINER_INLINE | A blocked container with inline items. |
TYPE_CONTAINER_NO_MARKUP | A container without surrounding markup. |
TYPE_CONTAINER_ORDERED_LIST | An ordered list container. |
TYPE_CONTAINER_UNORDERED_LIST | An unordered list container. |
TYPE_CONTAINER_ITEM_UNDEFINED | A container item without mapping. |
TYPE_LINK_EXTERNAL | Link to a page outside the current application. |
TYPE_LINK_INTERNAL | Link to a page inside the current application. |
TYPE_LINK_RESOURCE | Link to a CMS resource. |
TYPE_MANAGE_MENU_BUTTON | A manage menu button. |
TYPE_MANAGE_CONTENT_BUTTON | A manage content button. |
The Page
class represents the brXM page to render. This is the main entry point to the page model.
Method | Description |
---|---|
getButton(type: string, ...params: any[]): MetaCollection | Generates a meta-data collection for the Experience Manager buttons. |
getChannelParameters(): object | Gets current channel parameters. |
getComponent(...componentNames): Component | undefined | Gets a component in the page (e.g. getComponent('main', 'right') ). If componentNames is omitted, then the page root component will be returned. |
getContent(reference: Reference | string): Content | T | undefined | Gets a content item used on the page. |
getDocument(): T | undefined | Gets the page root document. This option is available only along with the Experience Pages feature. |
getMeta(meta): MetaCollection | Generates a meta-data collection from the provided meta model. The method is deprecated and will be removed in the next major release. |
getTitle(): string | undefined | Gets the title of the page, or undefined if not configured. |
getUrl(link?: Link): string | Generates a URL for a link object. - If the link object type is internal, then it will prepend spaBaseUrl or baseUrl . In case when the link starts with the same path as in cmsBaseUrl , this part will be removed.- If the link object type is unknown, then it will return undefined .- If the link parameter is omitted, then the link to the current page will be returned. - In other cases, the link will be returned as-is. |
getUrl(path: string): string | Generates an SPA URL for the path. - If it is a relative path and cmsBaseUrl is present, then it will prepend spaBaseUrl .- If it is an absolute path and cmsBaseUrl is present, then the behavior will be similar to internal link generation.- If it is a relative path and endpoint is present, then it will resolve this link relative to the current page URL.- If it is an absolute path and endpoint is present, then it will resolve this link relative to the baseUrl option. |
getVersion(): string | undefined | Returns the Page Model version. |
getVisitor(): Visitor | undefined | Gets the current visitor information, or undefined if the Relevance Module is not enabled. The Visitor object consists of the following properties:- id: string - the current visitor identifier;- header: string - an HTTP-header using to pass the visitor identifier to the Page Model API. |
getVisit(): Visit | undefined | Gets the current visit information, or undefined if the Relevance Module is not enabled. The Visit object consists of the following properties:- id: string - the current visit identifier;- new: boolean - a flag showing that this is a new visit. |
isPreview(): boolean | Returns whether the page is in the preview mode. |
rewriteLinks(content: string, type?: string): string | Rewrite links to pages and resources in the HTML content. This method looks up for a tags with data-type and href attributes and img tags with src attribute. Links will be updated according to the configuration used to initialize the page. The type parameter is similar to mimeType parameter of the DOMParser. |
sync(): void | Synchronizes the brXM integration state (UI elements positions). |
toJSON(): object | A plain JavaScript object of the page model. |
The Component
class corresponds to page nodes, and it may hold other components inside.
Method | Description |
---|---|
getId(): string | Returns the component id. |
getMeta(): MetaCollection | Returns the component meta-data collection. |
getModels(): object | Returns the map of the component models. |
getUrl(): string | undefined | Returns the link to the partial component model. |
getName(): string | Returns the name of the component. |
getParameters(): object | Returns the parameters of the component. |
getProperties(): object | Alias for getParameters method. |
getChildren(): Component[] | Returns the direct children of the component. |
getComponent(...componentNames: string[]): Component | undefined | Looks up for a nested component. |
getComponentById(id: string): Component | undefined | Looks up for a nested component by its id. |
The Container
class represents a page node that is actually present in the DOM but as an element surrounding its children. Container extends the Component class, and therefore, all the Component methods are applicable here.
Method | Description |
---|---|
getType(): string | undefined | Returns the type of a container. |
The ContainerItem
objects are usually visible on the page and interact with the user. Container Item extends the Component class, and therefore, all the Component methods are applicable here.
Method | Description |
---|---|
getLabel(): string | undefined | Returns the label of a container item catalogue component. |
getType(): string | undefined | Returns the type of a container item. The available types depend on which container items have been configured in the backend (e.g. "Banner"). |
isHidden(): boolean | Returns whether the component should not render anything. Hiding components is only possible with the Relevance feature. |
on(eventName: string, listener: Function): Function | Subscribes for an event and returns the unsubscribe function. |
off(eventName: string, listener: Function): void | Unsubscribes from an event. |
getContentReference(): Reference | undefined | Returns a RFC-6901 JSON Pointer to the content of this container item. |
getContent(page: Page): T | null; | Returns the content of this component from the page that contains the content. |
The Content
object holds document data that is used by the page components.
Method | Description |
---|---|
getId(): string | Returns the content id. |
getLocale(): string | undefined | Returns the content locale. |
getMeta(): MetaCollection | Returns the content meta-data collection. |
getName(): string | Returns the content name. |
getData(): object | Returns the content data as it is returned in the Page Model API. |
getUrl(): string | undefined | Returns the link to the content. |
The Document
object holds document data that is used by the page components.
Method | Description |
---|---|
getId(): string | Returns the document id. |
getLocale(): string | undefined | Returns the document locale. |
getMeta(): MetaCollection | Returns the document meta-data collection. |
getName(): string | Returns the document name. |
getData(): object | Returns the document data as it is returned in the Page Model API. |
getUrl(): string | undefined | Returns the link to the content. |
The ImageSet
object holds images collection that is used by the page components.
Method | Description |
---|---|
getDescription(): string undefined; | Returns the image set description. |
getDisplayName(): string | Returns the image set display name. |
getId(): string | Returns the document id. |
getFileName(): string | Returns the image set file name. |
getId(): string | Returns the image set id. |
getLocale(): string | undefined | Returns the image set locale. |
getName(): string | Returns the image name. |
getOriginal(): Image | undefined | Returns the original image. |
getThumbnail(): Image | undefined | Returns the thumbnail. |
The Image
object holds an image object that is used by the ImageSet
object.
Method | Description |
---|---|
getDisplayName(): string | Returns the image display name. |
getFileName(): string | undefined | Returns the image file name. |
getHeight(): number | Returns the image height. |
getMimeType(): string | Returns the image mime-type. |
getName(): string | Returns the image name. |
getSize(): number | Returns the image size. |
getUrl(): string | undefined | Returns the image link. |
getWidth(): number | Returns the image width. |
The Menu
object holds the page menu data with all the menu items.
Method | Description |
---|---|
getItems(): MenuItem[] | Returns the menu items. |
getMeta(): MetaCollection | Returns the menu meta-data collection. |
getName(): string | Returns the menu name. |
getSelected(): MenuItem | undefined | Returns the current menu item. The method is deprecated and will be removed in the next major release. |
The MenuItem
object holds a menu item that is used by the Menu
object.
Method | Description |
---|---|
getChildren(): MenuItem[] | Returns the child items. |
getDepth(): number | Returns the menu item depth level. |
getLink(): Link | undefined | Returns the menu item link. |
getName(): string | Returns the menu item name. |
getParameters(): object | Returns the menu item parameters. |
getUrl(): string | undefined | Returns the menu item url. |
isExpanded(): boolean | Returns whether the menu item is expanded. |
isRepositoryBased(): boolean | Returns whether the menu item is repository based. |
isSelected(): boolean | Returns whether the menu item is selected. |
Method | Description |
---|---|
clear(): void | Clears previously rendered meta-data objects. |
render(head: HTMLElement, tail: HTMLElement): () => void; | Renders meta-data objects on the page and returns the callback clearing rendered objects. |
The Meta
objects are being used by the brXM to page and its components.
Method | Description |
---|---|
getData(): string | Returns the meta-data. |
getPosition(): string | Returns the meta-data position relative to the related element. |
The Pagination
object holds the pagination data with all the pagination items.
Method | Description |
---|---|
getCurrent(): PaginationItem | Returns the current page. |
getFirst(): PaginationItem | Returns the first page. |
getItems(): Reference[] | Returns the current page items. |
getLast(): PaginationItem | Returns the last page. |
getNext(): PaginationItem | undefined | Returns the next page. |
getOffset(): number | Returns the number of items before the current page. |
getPages(): PaginationItem[] | Returns currently listed pages. |
getPrevious(): PaginationItem | undefined | Returns the previous page. |
getSize(): number | Returns the number of items listed on the current page. |
getTotal(): number | Returns the total number of items. |
isEnabled(): boolean | Returns whether the pagination is enabled. |
The PaginationItem
object holds a pagination item that is used by the Pagination
object.
Method | Description |
---|---|
getNumber(): number | Returns the page number. |
getUrl(): string | undefined | Returns the page URL. |
spa-sdk-17.0.1
18 October 2022
#90
#89
#88
#87
#SPASDK-129
#SPASDK-129
#SPASDK-129
#SPASDK-129
#SPASDK-129
#SPASDK-129
#SPASDK-129
#SPASDK-133
#SPASDK-133
#SPASDK-133
#SPASDK-133
#SPASDK-128
#SPASDK-118
#SPASDK-118
#SPASDK-116
FAQs
Bloomreach SPA SDK
The npm package @bloomreach/spa-sdk receives a total of 8,725 weekly downloads. As such, @bloomreach/spa-sdk popularity was classified as popular.
We found that @bloomreach/spa-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.