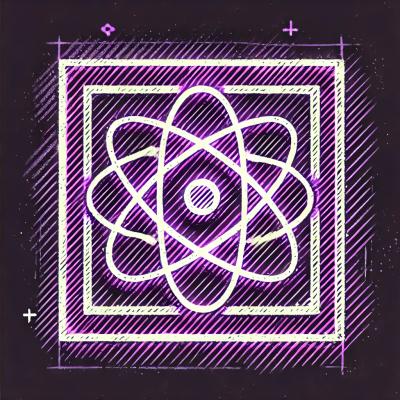
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
@bluejeans/web-client-sdk
Advanced tools
The BlueJeans Web Client Software Development Kit (SDK) enables embedding BlueJeans Video and Audio capabilities into web apps. BJNWebClientSDK is a single object that encapsulates all the API’s related to establishing a BlueJeans Video and Audio connection.
The design of the SDK emphasizes simplicity. Developers can quickly integrate BlueJeans video into their applications.
Not to be confused with:
Two steps to experience BlueJeans meetings using the web client SDK. They are as below :
As a pre requisite to using the BlueJeans Web Client SDK to join meetings, you need to have a BlueJeans meeting ID. If you do not have a meeting ID then you can create one using a meeting schedule option using a BlueJeans account as below
Integrate the SDK by using the following guidelines and use SDK APIs to join a meeting with the meeting ID generated.
To add @bluejeans/web-client-sdk
as a dependency to your package.json
file
npm i @bluejeans/web-client-sdk
Note: if you are using styled-components as a dependency in your package, please use version 3.*.*
. However recommended version is 3.4.5
import the BJNWebClientSDK
in your project using
import { BJNWebClientSDK } from '@bluejeans/web-client-sdk';
and to create a new instance of the SDK
let webClientSDK = new BJNWebClientSDK();
Note: Only one instance of BJNWebClientSDK can be present in app at a time. Creating multiple instances may cause unexpected errors.
BJNWebClientSDK has dependency on styled-components which internally has dependency on ReactJs. Please refer this article to get more info.
Hence this style of usage of BJNWebClientSDK requires the styled-components CDN bundles, react & related modules (before BJNWebClientSDK.js script)
<script crossorigin src="https://unpkg.com/react@16.12.0/umd/react.production.min.js"></script>
<script crossorigin src="https://unpkg.com/react-dom@16.12.0/umd/react-dom.production.min.js"></script>
<script crossorigin src="https://unpkg.com/react-is@16.12.0/umd/react-is.production.min.js"></script>
<script crossorigin src="https://unpkg.com/styled-components@3.4.5/dist/styled-components.js"></script>
<script crossorigin src="https://unpkg.com/@bluejeans/web-client-sdk@latest/dist/BJNWebClientSDK.js"></script>
Create a new instance of the SDK using
var webClientSDK = new BJNWebClientSDK.BJNWebClientSDK();
APIs are grouped into relevant services as shown in the architecture diagram. All the service objects are available all the time after SDK instantiation, however all services are not active at all the time. When inactive, APIs of the services will be null or undefined
These services are available as soon as we initialize the SDK, and are available throughout out-of-meeting & in-meeting.
Eg: MeetingService, VideoDeviceService, AudioDeviceService, LoggingService and PermissionService.
Unlike Globally active services, InMeeting services get activated when ConnectionState transitions to CONNECTED and get inactivated when meeting ends by the transition of connection state to IDLE
Eg: ContentShareService, ParticipantsService, PublicChatService, PrivateChatService, ModeratorControlsService
Detailed documentation of SDK functions is available here.
Following are the states which can be observed by subscribing to connectionState :
IDLE
- is a state which determines that a meeting is not in progress.VALIDATING
- meeting credentials are being validatedCONNECTING
- is a state which determines that meeting credentials were successfully authenticated and you are about to join the meeting.RECONNECTING
- this state is produced when a network glitch occursCONNECTED
- this state determines that you are finally into the meeting.Below is a diagram depicting the meeting state transitions:
webClientSDK.meetingService.joinMeeting("<Meeting_ID>","<PASSCODE>", "<DISPLAY_NAME>").then(() => {
console.log("Success");
},(error)=>{
console.error("Meeting join failed with error: ", JSON.stringify(error));
})
Note: The BJNWebClientSDK ties its session to the meeting ID given here. Also, since there can't be more than one instance of the SDK in the same webapp, if the meeting join fails for some reason or you would like to join another meeting after leaving the first one, you would need a page reload
Video views for Local video, Content video and Remote video can be added to your webpage as follows
Pass a video element for each of the following:
Now, attach these videos to the SDK
webClientSDK.meetingService.attachLocalVideo("<localVideoElement>");
webClientSDK.meetingService.attachRemoteContent("<contentvideoElement>");
Pass a div element for :
webClientSDK.meetingService.attachRemoteVideo("<remotevideoDivElement>");
Disconnect from the meeting using endMeeting
method in MeetingService.
webClientSDK.meetingService.endMeeting()
Note: when meetingService.connectionState
changes to IDLE state, then it can be safely assumed, participant has left the meeting.
Each service which has observable properties also exposes an observe API/function. observe allows observing changes to properties. Provide the property which you are interested in and a callback. Whenever the property value changes, you will be notified via the callback. Please note, observe doesn't allow observing changes to methods mentioned in the services.
Note: As mentioned in API Architecture section, InMeeting services get activated when ConnectionState transitions to CONNECTED. Please register all callbacks for inMeeting Services after connection state becomes connected to get accurate information
webClientSDK.meetingService.observe("connectionState", function() {
console.info("Connection state is changed to : ", webClientSDK.meetingService.connectionState)
});
You can set log level for the SDK by using setLoggingMode
method in LoggingService to one of the logging mode mentioned in docs.
webClientSDK.loggingService.setLoggingMode("<LoggingMode>");
Logs can be uploaded while reporting an issue using uploadLog
method in LoggingService
webClientSDK.loggingService.uploadLog("<Comments/Issue description>","<emailId>");
Note:
While initializing sdk in SDK customizations with saveLogsToLocalStorage: false
. Logs will not be stored in local storage. Beacause of this, Upload logs functionality will not work.
Use setAudioMuted
method in MeetingService to mute/unmute self audio and
setVideoMuted
method in MeetingService to mute/umnute self video
webClientSDK.meetingService.setAudioMuted(false) //to unmute your audio
webClientSDK.meetingService.setAudioMuted(true) //to mute your audio
The webrtc SDK will use your default devices out of the box. If you want to choose which camera/speaker/mic to use. You can see the list of available devices by calling videoDeviceService.availableCameras
, audioDeviceService.availableMicrophones
or audioDeviceService.availableSpeakers
and then use videoDeviceService.selectCamera()
, audioDeviceService.selectMicrophone()
or audioDeviceService.selectSpeaker()
to choose camera, mic and speaker devices respectively.
Note: Most browsers expose the list of devices available only when the user has given audio/video permissions. So please ensure you have called either permissionService.requestAllPermissions or meetingService.joinMeeting before attempting to change devices
let availableCameras = webClientSDK.videoDeviceService.availableCameras;
webClientSDK.videoDeviceService.selectCamera(availableCameras[1]);
Additional Note:
Sometimes browser/OS restrict speaker/microphone selection.
Please ensure you have called either AudioDeviceService.isSpeakerSelectionAllowed
or AudioDeviceService.isMicrophoneSelectionAllowed
before doing speaker/microphone selection.
if(webClientSDK.audioDeviceService.isMicrophoneSelectionAllowed) {
let availableMicrophones = webClientSDK.audioDeviceService.availableMicrophones
webClientSDK.audioDeviceService.selectMicrophone(availableMicrophones[0])
}
if(webClientSDK.audioDeviceService.isSpeakerSelectionAllowed) {
let availableSpeakers = webClientSDK.audioDeviceService.availableSpeakers
webClientSDK.audioDeviceService.selectSpeaker(availableSpeakers[0])
}
Initiate screen share by using startContentShare
method in ContentShareService
if(webClientSDK.contentShareService.isContentShareSupported) {
webClientSDK.contentShareService.startContentShare();
}
To get a list of participants in a meeting, use participantService.participants
property. It returns an array of participant
.
To change your current video layout, call meetingService.setVideoLayout()
function with one of VideoLayout
mentioned in docs.
From sdk we have exposed PublicChatService & PrivateChatService
Helps in sending messages to all participants in a meeting. These messages will be visible to all participant.
Send: a new public chat message using sendMessage in PublicChatService
webClientSDK.meetingService.publicChatService.sendMessage("Hello World public");
Receive: You can get the entire list of messages in a meeting (including new messages) by observing chatHistroy.
webClientSDK.meetingService.publicChatService.observe("chatHistory", function() {
console.info("public chatHistory : ", webClientSDK.meetingService.publicChatService.chatHistory)
});
Users can also subscribe to newMessage
event in PublicChatStoreEvents to get new messages.
Helps in sending messages to individual private messages to selected participant. These messages will be visible to only selected participant.
Send a new private chat message using sendMessage in PrivateChatService
webClientSDK.meetingService.privateChatService.sendMessage("Hello participant", "<participantGuid: string>");
Receive You can get the entire list of messages in a meeting (including new messages) by observing chatHistoryByParticipant. This would give a map of participants and your direct message history with them.
webClientSDK.meetingService.privateChatService.observe("chatHistory", function() {
console.info("private chatHistory updated: ", webClientSDK.meetingService.privateChatService.chatHistoryByParticipant);
});
Users can also subscribe to newMessage
event in PrivateChatStoreEvents to get new messages.
Closed Captioning is the ability to provide text for the words spoken in meetings. BlueJeans is providing means for the meeting participant to turn-on or turn-off Closed Captioning while in the meeting. Please note that Closed Captioning setting should be enabled on your meeting. As of now, only english language is supported.
startClosedCaptioning()
stopClosedCaptioning()
isClosedCaptioningAvailable
provides availability of the closed captioning feature. Feature can be enabled or disabled at the scheduling options or the meeting feature associated with the account.closedCaptionText
provides closed captioning text.closedCaptioningState
provides the current state of closed captioning, can be started or stopped or null (when not in meeting)isClosedCaptioningOn
checks if closed captioning is active in a meeting which has closed captioning capabilityDetailed documentation of ClosedCaptioningService functions is available here
Using BlueJeansSDKInitParams
one can customize the SDK experience by setting
Options listed in customizationParams will help customize different background colours
let webClientSDK = new BJNWebClientSDK({
customizationParams:{
audioTileColor:"red",
containerColorOfAllTiles:"blue",
videoTileBackgroundColor:"green"
}
})
This initial parameter saveLogsToLocalStorage will help customers configure logs saved to local storage
let webClientSDK = new BJNWebClientSDK({
saveLogsToLocalStorage: true
})
Note : Default value of saveLogsToLocalStorage is true. Once set to false, logs will not be saved to local storage, and also can not be uploaded. Also refer Upload-logs section.
Remote Video refers to the video coming in from other participants. When a participant joins a meeting with other participants already in it. There will multiple tiles/boxes which will contain other participants.
Each tile has some features attached to it:
On other browsers we support layout of remote video as described above. However on firefox, we support remote video as following:
The Web Client SDK makes use of WebRTC APIs exposed by the browsers to get streams from Camera and Microphone. Due to security concerns, most browsers do not expose the WebRTC APIs in an http page. We recommend you use https while developing locally as well. Most modern build tools like webpack (through webpack dev server) support https out of the box.
We have bundled two sample apps in this repo. One for package manager and another for Vanilla Js setup. It showcases the integration of BlueJeans SDK for permission flow and joins the flow. They have got a basic UI functionality.
BlueJeans collects data from app clients who integrate with SDK to join BlueJeans meetings like device information (ID, OS, etc.), coarse location (based on IP address), and usage data.
The BJNWebClientSDK is closed source and proprietary. As a result, we cannot accept pull requests. However, we enthusiastically welcome feedback on how to make our SDK better. If you think you have found a bug, or have an improvement or feature request, please file a GitHub issue or reach out to our support team at https://support.bluejeans.com/s/contactsupport and we will get back to you. Thanks in advance for your help!
Copyright © 2022 BlueJeans Network. All usage of the SDK is subject to the Developer Agreement that can be found in our repository as License file. Download the agreement and send an email to api-sdk@bluejeans.com with a signed version of this agreement. Before any commercial or public facing usage of this SDK.
Use of this SDK is subject to our Terms & Conditions and Privacy Policy.
FAQs
BlueJeans Web Client SDK
The npm package @bluejeans/web-client-sdk receives a total of 0 weekly downloads. As such, @bluejeans/web-client-sdk popularity was classified as not popular.
We found that @bluejeans/web-client-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 25 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.